Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial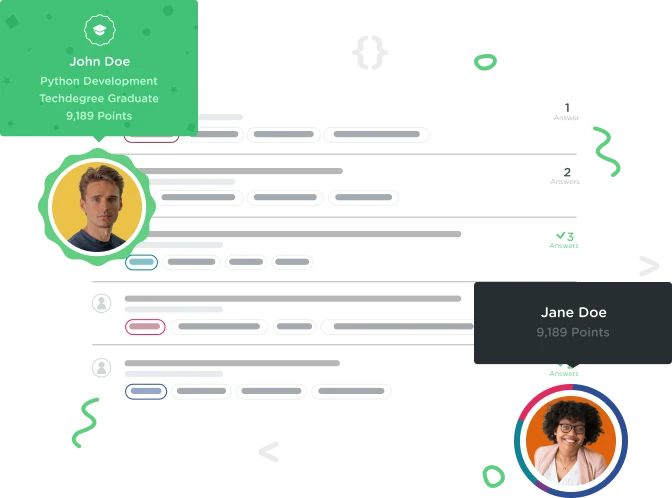

Aviral Dixit
232 Pointsplease help not able to find error
please help
class RaceCar:
laps=0
def __init__(self,color,fuel_remaining,**kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key,value in kwargs.items():
setattr(self,key,value)
def run_lap(self,length,fuel_remaining):
laps=laps+1
self.fuel_remaining=self_remaining
self.length=length
return self.fuel_remaining-(length*0.125)
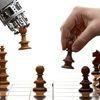
horus93
4,333 PointsSo first things first, be careful because you're doing to much here, the challenge only asks for the new method to take length as an additional argument, but you've also added in fuel_remaining.
class RaceCar:
laps = 0
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
def run_lap(self, length, fuel_remaining): # fuel_remaining is unnecessary, you already defined it in def__init__
laps = laps + 1 # you're not telling the method to refer to the laps attribute
self.fuel_remaining = self_remaining # what is self_remaining?
self.length = length
return self.fuel_remaining - (length*0.125) # you don't need to return anything here, but also, see how you're calling fuel_remaining from self.fuel_remaining? It's not using the self.fuel_remaining = self_remaining you defined here a couple lines up, which isn't doing anything other than redefining the variable you'd already set for no reason.
Remember challenge 2 asks only for the length argument to be added as a variable of the method, and it doesn't need to return anything, it's just changing the already stored values for fuel_remaining and the laps attribute. Like myself and I'd wager many new programmers you're just overthinking the problem, run_lap for task 2 only needs 2 lines of code to achieve what the task desires. Mull it over and I think you can get it, i'll check back in later.
1 Answer

KRIS NIKOLAISEN
54,969 PointsFor the task you don't need to pass in fuel_remaining as it will be calculated. There is also nothing to return.
def run_lap(self,length):
self.laps=self.laps+1
self.fuel_remaining = self.fuel_remaining-(length*0.125)
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsEwerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsI don't know exactly what the challenge asks you to do, but looking at your code I've spotted some errors. I'm gonna use your code and comment how the line probably should be written. Take a look:
Again, I'm gonna rewrite below the comments:
If you still have questions, feel free to ask.
EDIT: I just saw KRIS NIKOLAISEN answer to you. He knows what challenge you're in, so he's answer should be more specific. I just commented line by line on your code based on what I find you probably wanted to write.