Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial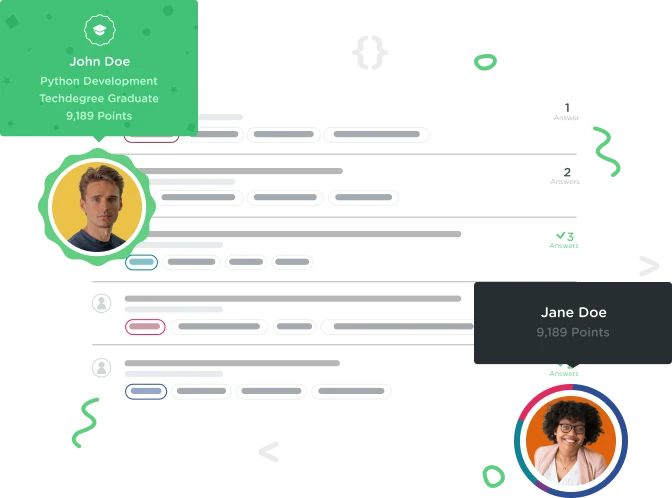

Adam Ocasio
1,742 Pointsplease help with basic shadowing concept
var person = "Jim";
function whosGotTheFunc() { person = "Andrew"; }
person = "Nick";
whosGotTheFunc();
console.log(person);
so this question on the quiz asks what is the output of the 'console.log'. The correct answer is Andrew on the quiz, but i can't for the life of me understand why. Shouldn't the answer be Nick?
I know since Andrew does not have the 'var' in front it changes the global var person. But doesn't 'person = Nick' also change the global var?
3 Answers
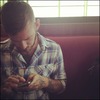
Erik McClintock
45,783 PointsAdam,
Yes, both of those change the global variable, and that's where you need to take the physical position of each assignment into consideration. The function that changes the variable is declared above the direct change to it, but then the CALL to the function (which is what will actually run the code inside that physically alters the variable's value) happens AFTER the direct assignment, thus, by the time the console.log statement is called, the value held within the "person" variable has just been changed back to Andrew.
Breaking it down, it goes like this:
//here, we declare and instantiate a variable called person, setting it equal to the value of "Jim"
var person = "Jim";
//here, we declare a function. that's all that we're doing here. functions do NOTHING until we explicity USE (i.e. "call") them
function whosGotTheFunc() { person = "Andrew"; }
//here, we are changing the value of the global variable "person" to equal "Nick"
person = "Nick";
//here, we are actually USING (i.e. "calling") the "whosGotTheFunc" function, which runs the code inside the function, which in this case is another override of the value assigned to the global variable "person", making it now equal "Andrew"
whosGotTheFunc();
//here, we are logging the current value of "person" to the console, and since the "whosGotTheFunc" function was just run, the value of the "person" variable at this point in time is indeed "Andrew", as it was assigned in that function
console.log(person);
Erik

Adam Ocasio
1,742 PointsAhhhh thank you so much. I love this site I get such timely responses it's great.
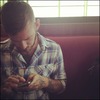
Erik McClintock
45,783 PointsAgreed! The community in these forums is fantastic, just like the teachers and the people who put it all together, which is incredible. Treehouse has done a great job of cultivating a very respectable, much-needed group of designers and developers that are willing and able to help their fellow students/colleagues, as opposed to the all-too-common elitist types that you can run into in the field.
Should you need assistance in the future, don't hesitate to post! Just make the effort to provide enough information in your post to clearly outline your problem (as you did here!) and what you've tried on your own to solve it (if applicable), and you'll get solid, clear help in no time!
Happy coding!
Erik
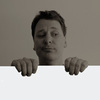
Sean T. Unwin
28,690 PointsI know since Andrew does not have the 'var' in front it changes the global var person. But doesn't 'person = Nick' also change the global var?
Yes, however whosGotTheFunc();
is being called after person = "Nick";
So the history of value of person
is:
var person = "Jim"; // person = 'Jim'
function whosGotTheFunc() { person = "Andrew"; }
person = "Nick"; // person = 'Nick'
whosGotTheFunc(); // person = 'Andrew'
console.log(person);
You can test this by placing console.log(person);
after each line. You will see that after function whosGotTheFunc() { person = "Andrew"; }
is declared that person
is still 'Jim` because that is declaring the function, not calling it.