Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial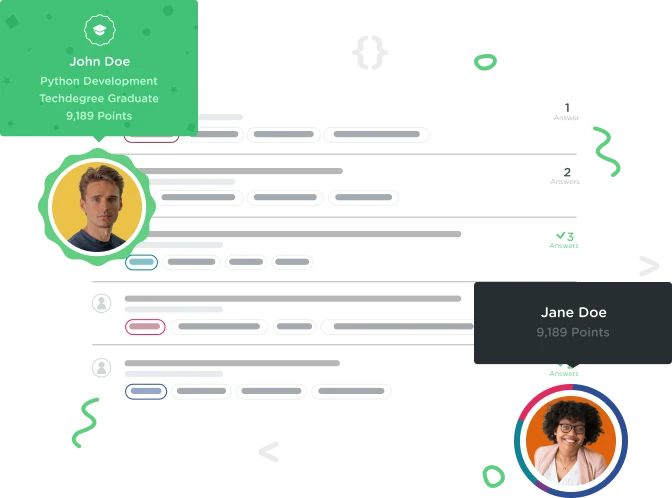

Deividas Strioga
12,851 PointsPlease help with challenge task 3
This is what i have done:
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
import java.util.HashMap;
public class QuickFix {
public void addForgottenVideo(Course course) {
Video video = new Video("The beginning bits");
int index = 1;
course.getVideos().add(index, video);
// TODO(1): Create a new video called "The Beginning Bits"
// TODO(2): Add the newly created video to the course videos as the second video.
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
if (videosByTitle(course).containsKey(oldTitle)); {
videosByTitle(course).put(newTitle, videosByTitle(course).get(oldTitle));
videosByTitle(course).remove(oldTitle);
}
}
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> byTitle = new HashMap<>();
for (Video video : course.getVideos()){
byTitle.put(video.getTitle(), video);
}
return byTitle;
}
}
Task 3 asks to fix fixVideoTitle method. There is no compilation error, but when i try to check the answer, it says Bummer! Are you sure you updated the videos title? Why my method doesn't change the title?
Thanks in advance.
2 Answers
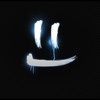
Grigorij Schleifer
10,365 PointsHi Deividas,
here my QuickFix.class:
import com.example.model.Course;
import com.example.model.Video;
import java.util.Map;
import java.util.HashMap;
import java.util.Map;
public class QuickFix {
public void addForgottenVideo(Course course) {
Video video = new Video("The beginning Bits");
course.getVideos().add(1, video);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Video videoValue = videosByTitle(course).get(oldTitle);
// store the old titled video in videoValue
videoValue.setTitle(newTitle);
// change the state of videoValue to new Title
}
public Map<String, Video> videosByTitle(Course course) {
Map<String, Video> byTitle = new HashMap<>();
for (Video video: course.getVideos()) {
byTitle.put(video.getTitle(), video);
}
return byTitle;
}
}
I have changed my fixVideoTitle method and made it more readable :)
Let me know if it was helpfull
Grigorij

Deividas Strioga
12,851 PointsJust before i saw this code i passed the challenge with this fixVideoTitle method (inspired by your last post):
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
for (Video video : course.getVideos()){
if (videosByTitle(course).containsKey(oldTitle)); {
video.setTitle(newTitle);
}
}
}
Your code works as well, and it is way more simpler! I guess I over-complicate the code :) Thank you so much for your help and support Grigorij! Good luck with your tasks!
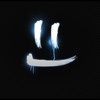
Grigorij Schleifer
10,365 PointsHey Deividas,
thank you too !
For answering your question I repeated the challenge and created this new simpler approach :)
See you in the forum !
Grigorij

Denis Migounov
17,657 PointsHi Grigorij, I think that, having changed a video title in fixVideoTitle, we need to rebuild the HashMap by calling videosByTitle(course), otherwise the map would still contain oldTitle as a key and we wouldn't be able to find newTitle in the map.
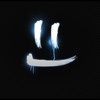
Grigorij Schleifer
10,365 PointsHI Deividas,
try this:
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
Map<String, Video> map = videosByTitle(course);
// creating a Map and using the videoByTitle method to generate a Map
for (Video vid: course.getVideos()) {
// βfor every video in all videos from the courseβ
if (vid.getTitle().equals(oldTitle)) {
// vid.getTitle() gets you the title from every video
// equals(oldTitle) and compares it with the old title
// so if the condition is true (vid.getTitle() gives you a title that is equal to oldTitle
vid.setTitle(newTitle);
// set a new title
// baaaaaaaaam !!!
}
}
Hope that helps
Grigorij

Deividas Strioga
12,851 PointsHey Grigorij,
Thank you for your answer. But your code doesn't work as well :) The task asks to use previously created videosByTitle(Course course) method. Any thoughts?

Danilo ANRIA
1,571 Pointswhy did you create the map ? I passed the challenge without using the videosbyTitle() map. I could not find a way to use it properly until I saw Deividas Strioga use the containsKey() method.

Iheke Iheke-agu
Full Stack JavaScript Techdegree Student 2,307 PointsThanks. Nice Job.
Ronnie Barua
17,665 PointsRonnie Barua
17,665 PointsYou are missing a carly brace.