Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial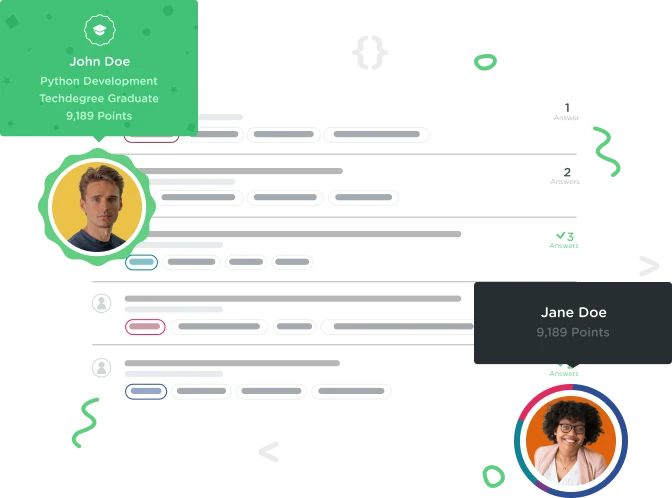
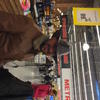
Isam Al-Abbasi
2,058 PointsPlease help with IllegalArgumentException!!
Here is the question: Okay, so let's throw an IllegalArgumentException from the drive method if the requested number of laps cannot be completed on the current battery level. Make the exception message "Not enough battery remains".
And as usual I don't know what to do!!
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
if (mBarsCount < 0){
throw new IllegalArgumentException("Not enough battery remains");
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers
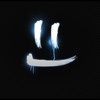
Grigorij Schleifer
10,365 PointsHi Isam,
you need to catch the exception if namber of laps (your argument of the drive method) is greater that mBarsCount. Put an If condition inside the drive method and throw the exception if there are less bars then laps available.
Your code could look like this:
public void drive(int laps) {
if (mBarsCount - laps < 0) {
// if number of laps is greater then mBarsCount the result is less the 0
throw new IllegalArgumentException("Not enough battery remains");
} else {
mBarsCount -= laps;
// subtract the number of laps of the mBarsCount
}
}
Grigorij
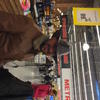
Isam Al-Abbasi
2,058 PointsI get it now, thanks a lot for your help :)

Derek Markman
16,291 PointsJust to throw in my 2 cents to this discussion. Say if when the IllegalArgumentException is thrown and then handled in your try-catch block, your program automatically ends. If that is the case, then the 'else' portion of the if-else statement would be redundant(not necessary) because no other code would be able to run or execute after your exception is thrown.
But in a lot of programs, even when an exception is thrown the program will still continue to run, and if that is the case, then the 'else' portion would be completely necessary to make sure no other code inside of the method that throws the exception continues to run, or gets invoked.
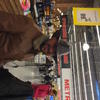
Isam Al-Abbasi
2,058 PointsThanks Derek, your 2 cents are helping a lot ;)

Derek Markman
16,291 PointsNo problem.
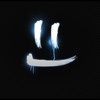
Grigorij Schleifer
10,365 PointsGreat explanation Derek, as always :)

Derek Markman
16,291 PointsThanks :)
Isam Al-Abbasi
2,058 PointsIsam Al-Abbasi
2,058 PointsThank you for the answer Grigorij, but what is this else keyword you used please? and why you used it?
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHi Isam,
you can transfer it into a real world language. The method takes the number of laps you want to drive. The if condition says "if the number of Bar minus number of laps is less then 0 ( more laps then Bars) throw an exception". ELSE do something. Here subtract the number of laps of the Bars count.
So if there are more bars then laps ... no exception is thrown, but bars count is diminisched.
Makes sense?
Isam Al-Abbasi
2,058 PointsIsam Al-Abbasi
2,058 PointsIt does now :) But should the if statement be something like this: if (mBarsCount - laps = 0)!! and about else keyword why not using catch like Craig was using in the video? I mean if it wasn't for you I would've never knew that there is ELSE keyword in java :D
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHey bro,
if you take this if (mBarsCount - laps = 0) it means that there are same number of bars as laps available. So you can drive until there are no more bars left. And the exception is thrown only if you plan to drive more laps then bars available. Makes sense?
You have 10 bars and the plan is to drive 10 laps ... no exceoption needed. But if you wanna drive 11 laps ... exception is thrown because not enough bars ...
Right?