Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial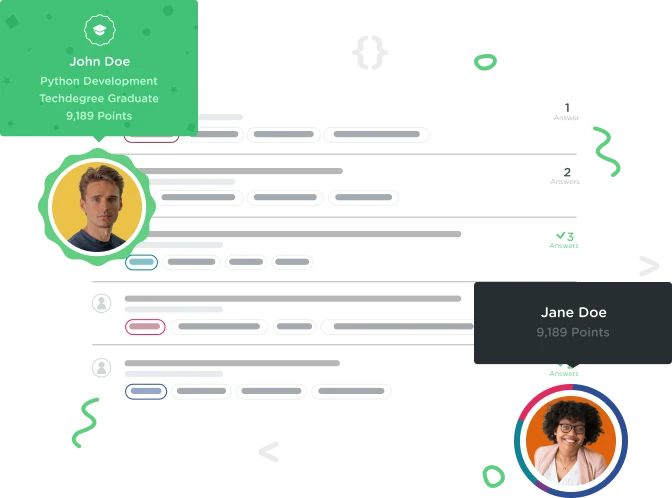

Gopin Cherupally
iOS Development Techdegree Student 74 PointsPlease help with this
Please help with this
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red,green,blue,alpha){
red.self = red
green.self = green
blue.self = blue
alpha.self = alpha
}
description = "red: \(red),green: \(green),blue: \(blue),alpha: \(alpha)"
}
2 Answers
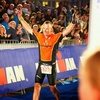
Steve Hunter
57,712 PointsHi there,
That looks mainly fine, bar some spacing issues in your string - there's a few issues, though:
The description
assignment needs to be inside your init
method, so move the closing braces to after that.
You want to have the self
keyword before the stored property name, like: self.red = red
.
You need to put types on your parameters; they are all of type Double
.
That all looks like:
init(red: Double, green: Double, blue: Double, alpha: Double){
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
Steve.

Saud Alfaris
1,084 PointsUse this code:
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}