Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial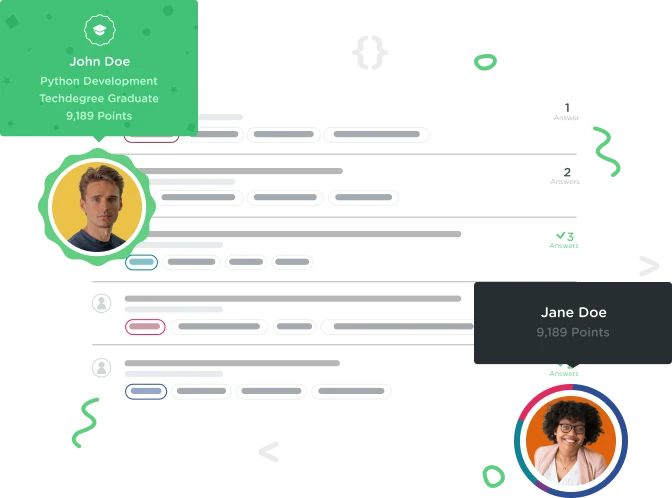

Victor Katsande
3,094 Pointsplease help with this one also, i have been stuck for a week.
The question wants me to remove all vowels from the word that is passed in the function [disemvowel(word)]. It also says i should check for both upper and lower cases of the vowels.
def disemvowel(word):
if word.upper():
word.remove("a")
word.remove("e")
word.remove("i")
word.remove("o")
word.remove("u")
elif word.lower():
word.remove("A")
word.remove("E")
word.remove("I")
word.remove("O")
word.remove("U")
return word
3 Answers

Erik Linden
14,313 PointsThere looks like a couple of items in here to do differently. Since word is a string, I don't believe that 'remove' is something that can be called and then passed a list. It should cause an error. Also the condition is just testing the string method of upper and lower. It is frustrating sometimes when the challenges just say "Bummer". Sometimes I have to open a workspace and try editing my code snippets there, so I can see the actual error python is giving.
This solution really needs a "for" loop, and if you force each letter to lower, just for testing, then case shouldn't matter.
This might be better if you use a var to hold the return value. Then test every letter in the word against the list of vowels. Don't lower the letter except for the testing so that if the return is expected to have an upper then it still will. If the letter isn't in the vowel list, pass it to the return var.
Maybe something like this:
def disemvowel(word):
out = ''
vowel = ["a", "e", "i", "o", "u"]
for l in word:
if l.lower() in vowel:
continue
else:
out+=l
return out
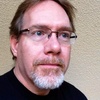
Chris Freeman
Treehouse Moderator 68,459 PointsThere are two basic issues with your solution.
The argument passed in is a string. A string is an immutable object. That is, it cannot be changed. The string type
str
does not have aremove
method.The method
upper()
returns a new copy of the string. In a true/false context of anif
statement, this will always be seen asTrue
making theelif
unreachable.
Also remember that the other non-vowels in word
should not change case.
A for
loop works well on this challenge. Try looping over each char in the word. If the char is an upper or lower vowel, then drop it, otherwise include the char in the output. This can be by appending to a list and using join
to change the list back to a string.
Post back if you need more help. Good luck!!

Yougang Sun
Courses Plus Student 2,086 PointsThe answer may be:
def disemvowel(word):
vowel=['a','e','i','o','u']
for letter in word:
if letter.lower() in vowel:
word.remove(letter)
return(word)