Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial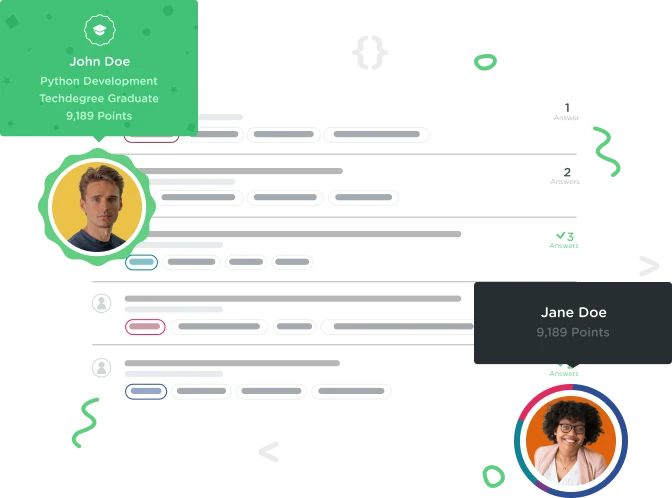

Pedro nieto Sanchez
28,169 PointsPlease I need help here in this string. Or maybe I am not getting right the question.
I have copied the string exactly the same but still i keep getting the message that says: that I am not usint the common_name. Could anyone help me?
<?php
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
class Trout extends Fish {
public $species;
function __construct($name, $flavor, $record, $species) {
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
public function getInfo(){
return $this->species . " ". $this->name . "tastes ". $this->flavor . ". The record ". $this->species . " ". $this->name . " weighted ". $this->record . ".";
}
}
$brook_trout = new Trout("Trout","Delicious","14 pounds 8 ounces","Brook");
echo $brook_trout->getInfo();
?>
2 Answers
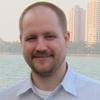
Ryan Field
Courses Plus Student 21,242 PointsHey, Pedro. Since the class Trout extends
Fish, you'll want to use the same attributes as its parent class. Right now you have:
<?php
public function getInfo(){
return $this->species . " ". $this->name . "tastes ". $this->flavor . ". The record ". $this->species . " ". $this->name . " weighted ". $this->record . ".";
}
?>
but you should be using $this->common_name
and $this->record_weight
.

mereigh
9,211 PointsHey Pedro! I struggled with this one for a bit and ultimately decided returning a full string was too long and complex. Here is a simpler way to complete this task:
<?php
class Trout extends Fish {
public $species;
public function __construct($name, $flavor, $record, $species) {
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
public function getInfo() {
$output = "{$this->species} {$this->common_name} tastes {$this->flavor}. ";
$output .= "The record {$this->species} {$this->common_name} weighed {this->record_weight}.";
return $output;
}
}
?>
Pedro nieto Sanchez
28,169 PointsPedro nieto Sanchez
28,169 PointsYou are right, i am sure the teamtreehouse put that example for people like me to fall in the trick. :)