Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial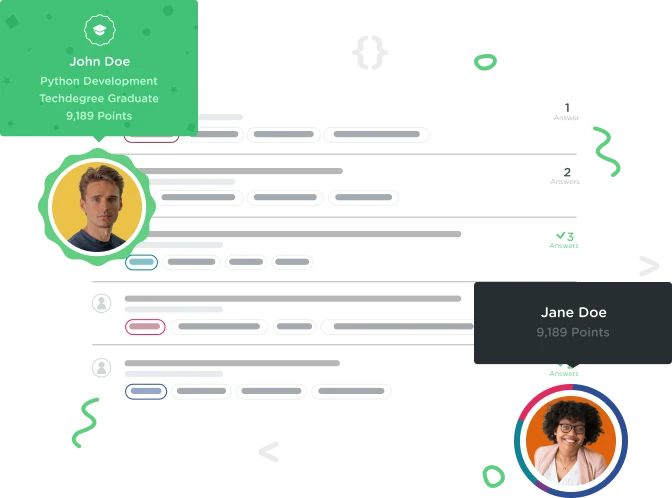

Kiran P
Courses Plus Student 919 PointsPlease review the code to check why Python is skipping the consonants as soon as it hits the first vowel in word.
Please review the code to check why Python is skipping the consonants as soon as it hits the first vowel in word.
def disemvowel(word):
print("the word is {}".format(word))
word = list(word)
print("the word is {}".format(word))
print("\n")
vowels = ['a','e','i','o','u']
temp = None
print("The temp value is {}".format(temp))
for mem in word:
print(mem)
print("The mem is {}".format(mem))
print("\n")
if mem.lower() in vowels:
print("{} is a vowel".format(mem))
word.remove(mem)
print("{} removed".format(mem))
else:
if temp == None:
temp = mem
print("The value of temp is {}".format(temp))
print("\n")
else:
temp += mem
print("The value of temp is {}".format(temp))
print("\n")
word = temp
return word
disemvowel('DytEQi')
1 Answer
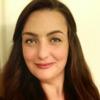
Jennifer Nordell
Treehouse TeacherHi there! The problem with using the remove
is that you're changing the thing you're iterating over. Essentially, it's messing up the indexing of the iterable. Every time you remove
something, the following letter (whatever it is) will not be checked.
I've posted a couple of answers and threads on this. Take a look:
- https://teamtreehouse.com/community/removing-vowels-from-word-in-python
- https://teamtreehouse.com/community/having-difficulty-in-the-disemvowel-challenge
On the first, one I give a step by step account of what is happening at every iteration, but you will have to scroll down to the bottom of the thread to read it.
Hope this helps!
Kiran P
Courses Plus Student 919 PointsKiran P
Courses Plus Student 919 PointsThank you, Jennifer!
Kiran P
Courses Plus Student 919 PointsKiran P
Courses Plus Student 919 PointsI copied word list to a temp variable and iterated on word list and removed the vowels in temp, but Python still skips the letter after removing one.
I tried the copy(), that worked.
Could you explain why Python is skipping again even though I'm removing the items in temp, a copy of word list?
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherYou would have to show me your code for me to be able to answer that.
Kiran P
Courses Plus Student 919 PointsKiran P
Courses Plus Student 919 PointsJennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherKiran P Ah ha! the problem with this version of your code is that you didn't make a copy but rather a reference to the original list.
Take a look at this line:
temp = word
This tells Python that anything you do to
temp
, you also want to do toword
, which is exactly the thing we're trying to avoid. To make a copy, you could change that line to:temp = word[:]
This makes a copy of the word and stores it into
temp
.I made an example for you to run in workspaces so you can see the difference between manipulating a copy and manipulating a reference.
Try running this in workspaces and take a look at what prints out:
Hope this helps!
Kiran P
Courses Plus Student 919 PointsKiran P
Courses Plus Student 919 PointsThank you again, Jennifer!