Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial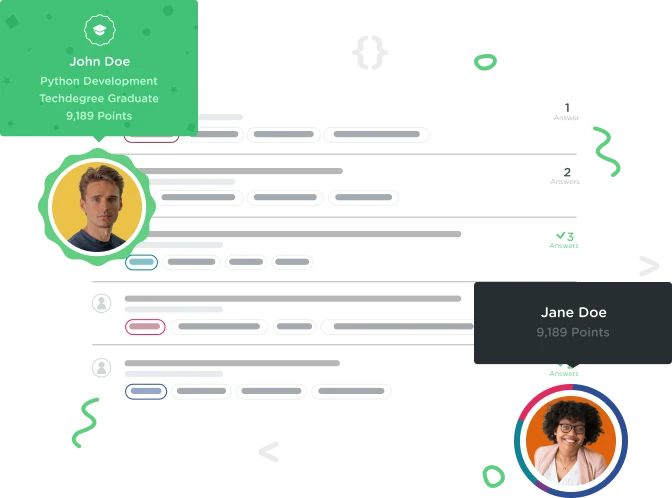
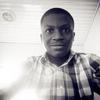
Eugene Paitoo
3,922 PointsPlease whats the problem with my code
The Feedback i get is "while you could solve it using an if statement, try returning the result of the expression
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
// TODO: Add the tile to tiles
tiles += tile;
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
boolean isTile = tiles.indexOf(tile) != 1;
if (isTile) {
return isTile;
} else {
return false;
}
}
}
4 Answers
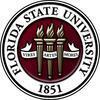
A Y
1,761 PointsHi Eugene,
You don't have to use the if conditional statement here as your return from boolean isTile = tiles.indexOf(tile) != 1; is true/false already so just typr return isTile; and it will work.
Change these: boolean isTile = tiles.indexOf(tile) != 1; if (isTile) { return isTile; } else { return false; }
To: boolean isTile = tiles.indexOf(tile) != -1; return isTile;
And it will work.
Regrds, Amir

Samuel Ferree
31,722 PointsYour hasTile method can be shortened
public boolean hasTile(char tile) {
boolean isTile = tiles.indexOf(tile) != 1; //Sidenote: I think this should be -1, not 1
return isTile
}
It can be shortened further (and correctly imo)
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
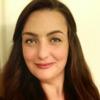
Jennifer Nordell
Treehouse TeacherHi there! While you could do it the way you are doing it, it's generally considered less readable. Ideally, we want to avoid a situation where we have something like "if this is true return true" and "if it's false return false". We'd want it to read more like "return whether or not this is true or false". Let's take an example. Imagine that we want to return true if a number is greater than 10 or false if it's not. We could do it like this:
int myNumber = 20;
if(myNumber > 10) {
return true;
} else {
return false;
}
But it's much shorter and more readable to return the evaluation of the expression directly as it can only be true or false. So we could write the same thing this way:
int myNumber = 20;
return myNumber > 10;
It's going to run the evaluation of myNumber > 10
which will have a boolean value of true or false. We then immediately return the result of that evaluation.
Your code is doing the evaluation just fine, but you can return it in this same manner without all the extra stuff. So you can remove the if else statements, and you don't even need the isTile
variable. Simply replacing boolean isTile =
with return
and erasing all the if
and else
will cause the challenge to pass!
Hope this helps!
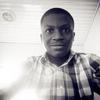
Eugene Paitoo
3,922 PointsThanks so much for your input