Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial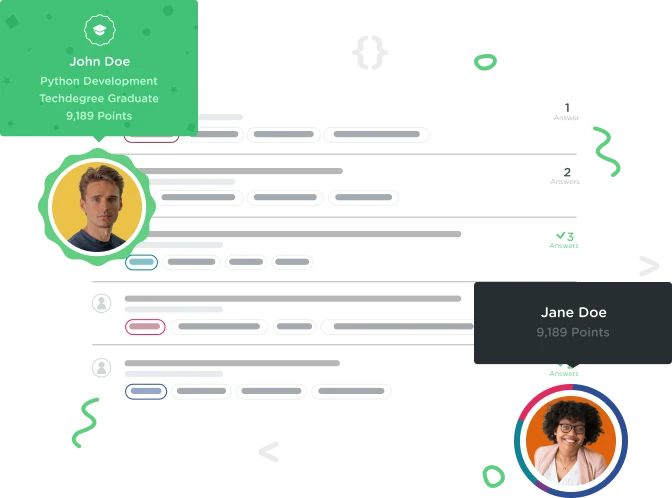
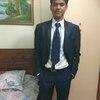
Ashmit Pathak
4,441 Pointsplz help me with a solution plz
can't understand what to do next. the result shown is "BUMMER"
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count("treehouse"):
return {"t": 1 ,
"r": 2,
"e": 3 ,
"e": 3 ,
"h": 4,
"o": 5,
"u":6 ,
"s":7,
"e": 3
}
1 Answer

Paul Kamarudin
5,292 PointsWhen i was doing the course i could always find the answers but there was never an explanation on why things were being done and so sometimes i would just stare at an answer for a while. Hopefully the logic i have outlined here helps.
When i started coding i found it easy to break the questions down into the individual requirements. I then would try to piece together on paper how the function would work. I know this might sound long winded but it gets you thinking in the right way and then eventually you simply do this in your head.
- Write a function named word_count passing string
- Needs to return a dictionary
- Dictionary keys will be each of the words in the string so will need to break string into words
- Dictionary keys need to be lowercased
- Value will be how many times the word appears in the string
So starting off, define the function and get it to pass the word string. You just need to change your variable to string to match what i am doing here.
Then we want to get the data passed by the string and break the string into words. At this point of the course, i think you just learn't how to use the split function and so you will need to use that.
word_list = string.lower().split()
I have added the lower function so that it turns the result into lowercase. The split function needs to pass all white space and so is left empty. Think that a space between words might be a space or even a tab.
Then you need to return a dictionary and start with it being empty.
dictionary = {}
Now for the harder part of walking thru the logic. We want to step thru the words in the string. While stepping thru the words we need to check to see if the word we are currently passing appears again in the string.
So one FOR loop to walk thru the words and another FOR with an IF to check if the current word is equal to any of the words in the list. IF yes then we increase a counter for each word.
Start with walking thru the words in the word_list and set out counter to 0.
for word in word_list:
count = 0
Then we need to check if the word repeats in the string (word_list). the original word (word) equal the repeated word (word_repeat) and if so then count the occurrence.
for word_repeat in word_list:
if word == word_repeat:
count +=1
After that we need to assign the count to the dictionary for the individual word.
dictionary[word] = count
Lastly you need to write the code to return the dictionary and hopefully will now have a working solution. You need to check that your indentation is correct and it should pass.
Let me know if this has helped.