Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial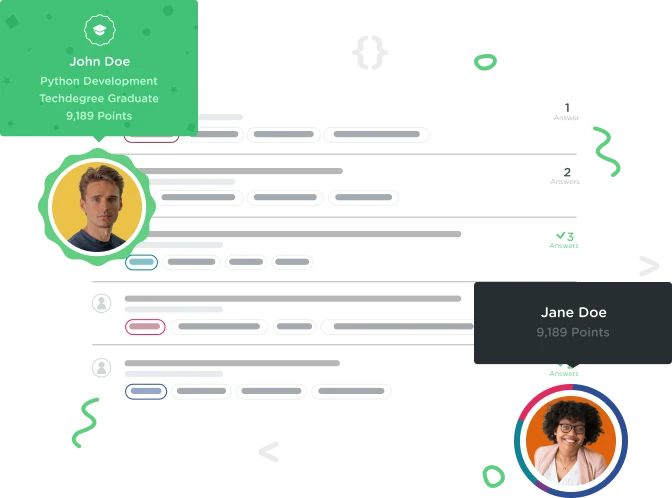

Morgan Norelius
682 Pointspoorly worded question: concatenate two arrays and rename the resulting expression with the same name as original?
I understand the concept of concatenating two arrays, but I am lost as to how to rename the result the same as one of the originals. It seems like circular logic.
How can a + b = a ?
I found this example on stack overflow: let array1 = [1, 2, 3] let array2 = [4, 5, 6]
let flattenArray = array1 + array2 print(flattenArray) // prints [1, 2, 3, 4, 5, 6]
// Enter your code below
var arrayOfInts: [Int] = [0, 1, 2, 3, 4, 5]
arrayOfInts.append(6)
4 Answers
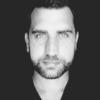
Jhoan Arango
14,575 PointsHello,
This is simple, once you have created an array of Ints that hold six values, you should then re-use the arrayOfInts and concatenate a new array with one value in it.
var arrayOfInts: [Int] = [1,2,3,4,5,6]
arrayOfInts.append(7) // We use the append method
arrayOfInts = arrayOfInts + [8] // Then we use the arrayOfInts and concatenate a new array with one value in it
Hope this helps

KRIS NIKOLAISEN
54,971 PointsIt wouldn't be a + b = a
. It would be a = a + b
or shorthand a += b
. Keep in mind it is an assignment not an equation.
Shorthand for the challenge might be:
arrayOfInts += [7]

Morgan Norelius
682 PointsThanks. I still struggle with the logic of what seems like a circular reference, but with the guiding of your example, I can accept the idea that the compiler will use the old value of arrayOfInts in the concatenation. I appreciate the help!

KRIS NIKOLAISEN
54,971 PointsYou will see this all the time - especially in loops. What is on the right hand side is evaluated then assigned to the left hand side.
A JavaScript example:
var i;
for (i = 0; i < 10; i++) {
console.log(i);
}
could also be written:
var i;
for (i = 0; i < 10; i = i + 1) {
console.log(i);
}
or:
var i;
for (i = 0; i < 10; i += 1) {
console.log(i);
}

Morgan Norelius
682 PointsThanks! visualizing the code as "right hand side" and "left hand side" is helpful. Now I understand how a = a + b