Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial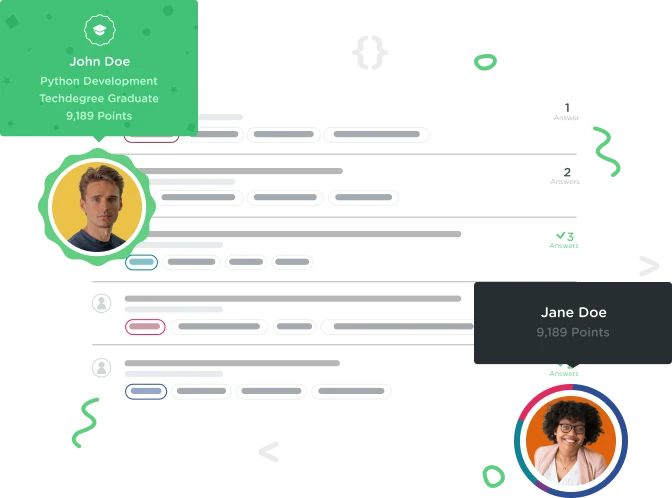
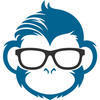
Ceil-Ian Maralit
Front End Web Development Techdegree Graduate 19,434 PointsPop vs Del
Hello!
This got me confused a bit. Does pop() and del have the same purpose?
Because Craig said that del is used to just delete the label and not the actual value. But he also said in the latter part that del is great if you want to delete the value and that he recommends pop() when we don't want the items deleted to be garbage collected.
BUT we can still access the values of the items deleted from the list when we assign it first to a variable before executing the command to delete it. Now, what's the difference between del and pop()? and when to use them?
Thanks in advance!
3 Answers
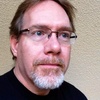
Chris Freeman
Treehouse Moderator 68,457 PointsGood question! A simple difference is pop()
returns the item removed so it can be assigned a different label while del
simply removes the item.
If you use pop()
and do not assign a new label to the returned object it is essentially performing the same function as del
.
The list of book title strings is actually a list of references that point to string objects stored in memory. Each string object "id" is the address in memory of the stored object.
b1 = "book title 1"
b2 = "book title 2"
b3 = "book title 3"
b4 = "book title 4"
book_list = [b1, b2, b3, b4]
print(book_list)
#['book title 1', 'book title 2', 'book title 3', 'book title 4']
print([id(b1), id(b2), id(b3, id(b4)])
# [139996358750368, 139996358750256, 139996358750592, 139996358750648]
print([id(book) for book in book_list])
# [139996358750368, 139996358750256, 139996358750592, 139996358750648]
saved = book_list.pop(0)
print(saved)
# book title 1
print(id(saved))
# 139996358750368
In a more detailed look, pop()
returns the "id" reference removed so that it can be assigned to another label or used in a subsequent statement. The label saved
is assigned to the first book popped from the book list.
So, use pop()
when you want to have access to the removed item for another purpose, and use del
when you no longer want the item.
Edit: to comment on “garbage collection”. Garbage collection happens when an object is removed from memory and the memory freed for other uses. This occurs when all references to an object are removed.
pop()
removed the list’s reference to object (count goes down by 1). If count is now zero, object maybe garbage collected. If a label is assigned to the popped object, this adds a new reference (count goes up by 1). So labeling a pop becomes a wash (no net change in reference count).
del
removes the label (the count goes down by 1) but not the object. Using del b4
simply removes the label b4
but not the object referenced by b4
since the string “book title 4”
is still referenced by the list.
Post back if you have more questions. Good luck!!
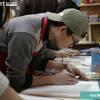
Cao Tru
Courses Plus Student 1,103 PointsReally good questions and answers. Thank you so much!

nikel Hayo
1,943 Pointsı was confused and Chris made crystal clear. Thanks a lot for both question and answer.
Ceil-Ian Maralit
Front End Web Development Techdegree Graduate 19,434 PointsCeil-Ian Maralit
Front End Web Development Techdegree Graduate 19,434 PointsHi! Thank you so much for this.
Though I still have a question. So how come we can still access the value deleted using del when we assign it to a variable before deleting it(I tried accessing them before deleting it with pop() and del, which made me really confused), if del means to delete it permanently, as we say it, garbage collected? Does del also provide id reference?
I do understand that it is recommended to use pop() when we want to access it still, after deleting it. Just curious why we can still do it with del. Thank you! :)
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsAssigning a variable is more like giving a label to an object reference. This increases the “reference count” of an object. Think of
del
as deleting the label reference to an object rather than deleting the object itself. If all label references to an object are removed (reference count become zero), then garbage collection will eventually remove the object from memory because it has no labels referencing that object.In the case above, this repl does not have strong garbage so the object can still be referenced by id value even though the last label had been removed. A bit later, the same attempt crashed the repl because garbage collection had removed the object!
Ceil-Ian Maralit
Front End Web Development Techdegree Graduate 19,434 PointsCeil-Ian Maralit
Front End Web Development Techdegree Graduate 19,434 PointsOhhh, okay. Now, I see the difference between the two. Though I did not understand the ctypes thing in the code coz I'm just a beginner in Python, but it doesn't matter. It still made sense to me. Thank you so much!
Firuz Abduganiev
3,140 PointsFiruz Abduganiev
3,140 Pointsvery cool example
nicolaspeterson
8,569 Pointsnicolaspeterson
8,569 PointsGreat answer Chris, but how can we assign a new label to an object after it's been returned using pop?
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 Pointsnicolaspeterson, I might be miss understanding your question, but it’s as simple as:
popped_obj = some_list[2]
. What’s really happening is a bit deeper. Comments added below to show a different way to think about how things work.In the end, it’s all about assigning labels to object ids.
some_list[2]
is just a reference for an id. A list is just an array of ids!