Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial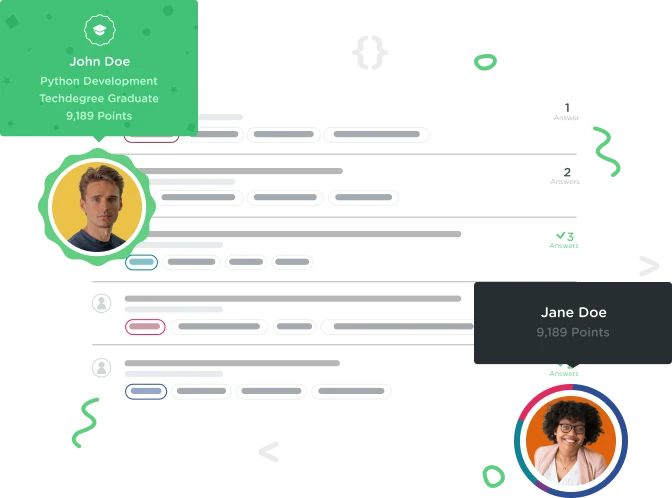

Takunda Chimbuya
3,096 Pointspopular board game, hands.py
please assist, getting value error
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
class Die():
def score_sixes(self):
return sum(hand.sixes)
2 Answers
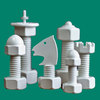
Steven Parker
231,269 PointsHere's a hint: When they say "First off, make sure it always rolls two D6s.", you probably will want to override __init__
so you can pass some specific arguments to the base class.

Taig Mac Carthy
8,139 PointsNot all heroes wear capes
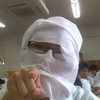
Zinaldo Park
15,469 Pointsfrom dice import D6
class Hand(list): def init(self, size=0, die_class=None, *args, **kwargs): if not die_class: raise ValueError("You must provide a die class") super().init()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand): def init(self, *args, **kwargs): super().init(size=2, die_class=D6, *args, **kwargs)
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
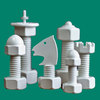
Steven Parker
231,269 Points FYI: According to a moderator, explicit answers without any explanation are strongly discouraged by Treehouse.
Also, since the code has not been formatted for Markdown, what is being displayed is not correct.
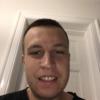
Andrii Gorokh
14,809 Pointsthis doesn't seem to work...
Takunda Chimbuya
3,096 PointsTakunda Chimbuya
3,096 Pointsthe question is We're playing a popular board game about snatching up real estate in Atlantic City. I need you finish out the CapitalismHand class. First off, make sure it always rolls two D6s.