Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial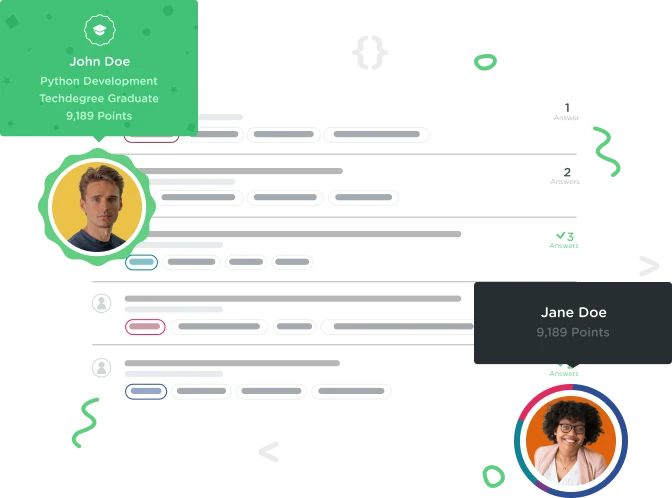

Lawrence Chang
4,659 PointsPopulate hash with array keys and default value
The question asked was: Complete the solution so that it takes an array of keys and a default value and returns a hash with all keys set to the default value.
def solution(keys, default_value)
hash = Hash.new
keys.each { |k| hash[k] = default_value }
hash
end
keys.each { |k| hash[k] = default_value }
Can someone explain what hash[k] = default_value does in the iteration. Thank you!
3 Answers

Nick Fuller
9,027 PointsHi Lawrence!
Remember you can access the value of a hash by calling it's key right? Like so
Given I have the following hash...
hash = { 'age' => 30, 'first_name' => 'Nick' }
When I pass a key to it with the [] syntax I wan to get the value for that key
hash['age']
=> 30
Likewise we can use the same mechanism to create a new key, value pair into the hash
Given I have the same hash as above
When a pass a value to the [] syntax with a new key I can set it's value; just like creating a normal variable
hash['last_name'] = 'Fuller'
=> "Fuller"
Now using the same logic above I can get that new value from that key
hash['last_name']
=> "Fuller"
And if we look at the entire hash we have
hash
=> {"age"=>30, "first_name"=>"Nick", "last_name"=>"Fuller"}
So your method would work like this...
candy = ["Skittles", "Starbursts", "Tacos"]
solution(candy, "$0.99")
=> { "Skittles" => "$0.99", "Starbursts" => "$0.99", "Tacos" => "$0.99" }

Lawrence Chang
4,659 Pointsnick you are freaking awesome! thank you!

Nick Fuller
9,027 PointsMy pleasure :)