Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial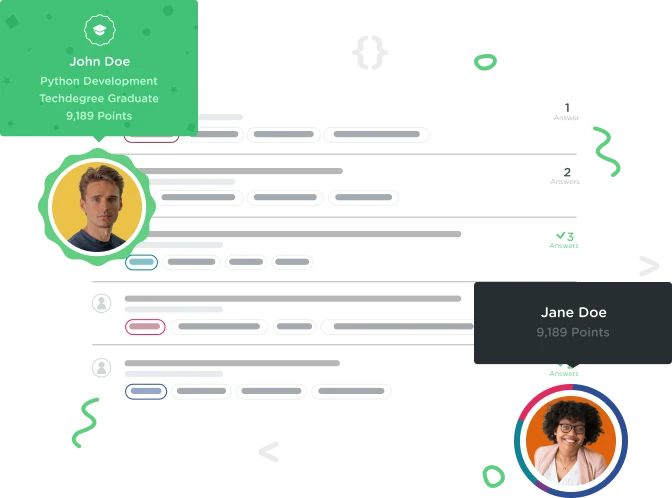
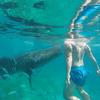
David Gross
19,443 PointsPopulating the database
I populated my database so I can have a better understanding of how to use queries to find large data. I did this using the gem (gem 'ffaker', '~> 1.24.0'). I put it in my lib/task file and have named it populating_accounts.rake. I am bit stuck on how to populate the account_entries, projects and employee_projects. If any one could add onto this it would be greatly appreciated.
Below is my code. to use it make sure you rake db:reset rake db:populate
task :sample_data => :environment do
require 'faker'
end
namespace :db do
desc "Fill database with sample data"
task populate: :environment do
make_customers
make_employees
end
end
def make_customers
Account.create!(name: "Done Bingerston",
email: "Bingerston@example.com",
type: "Customer",
about: "I enjoy fishing and scub diving"
)
800.times do |n|
name = Faker::Name.name
email = Faker::Internet.email
city = Faker::AddressUS.city_suffix
zipcode = Faker::AddressUS.zip_code
state = Faker::AddressUS.state
about = Faker::Company.bs
type = 'Customer'
employees = (rand * 90).to_i
Account.create!(name: name,
about: about,
city: city,
state: state,
employees: employees,
zipcode: zipcode,
email: email,
type: type)
end
end
def make_employees
Account.create!(name: "Danny stanzia",
email: "stanza@example.com",
type: "Employee"
)
45.times do |n|
name = Faker::Name.name
email = Faker::Internet.email
city = Faker::AddressUS.city_suffix
state = Faker::AddressUS.state
zipcode = Faker::AddressUS.zip_code
type = 'Employee'
Account.create!(name: name,
email: email,
type: type,
state: state,
city: city,
zipcode: zipcode)
end
end
2 Answers

Neil Northrop
5,095 PointsHey David,
I was checking out your setup and did it a little different to make it work on my machine.
First, I added faker to my Gemfile and ran ruby bundle install
.
Second, I used the seed.rb file in your db folder of your application. In the file, I extracted only this part of the code you were using and changed a couple things. Every time it loops, I'll create a new Account, fill it with the faker info, and save it to the database. The second thing I changed was the fact that Rails didn't let me save the 'Customer' data to a type field that you had, so I changed it to role. And the third thing I changed was AddressUS to just Address.
800.times do |n|
a = Account.new
a.name = Faker::Name.name
a.email = Faker::Internet.email
a.city = Faker::Address.city_suffix
a.zipcode = Faker::Address.zip_code
a.state = Faker::Address.state
a.about = Faker::Company.bs
a.role = 'Customer'
a.employees = (rand * 90).to_i
a.save
end
After that, I went to the console and just ran: ruby rake db:seed
That populated the db and I was able to look through all that fake data.
Let me know if that helps you at all. I know you were trying to make a new rake task but maybe just using the rake task supplied with Rails would be easier for you.
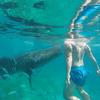
David Gross
19,443 PointsThanks for your feed back Niel. After I finished the course I realized they put how to use sample data in AR extensions. I am still struggling on how to figure out how to populate the account_entries data. I know I can do this with Random number method to use for ID but then I will get errors on my <%= entry.account.name %>
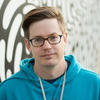
Jim Withington
12,025 PointsThanks for mentioning that this is covered later on! Gonna go check it out now, before I move along.