Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial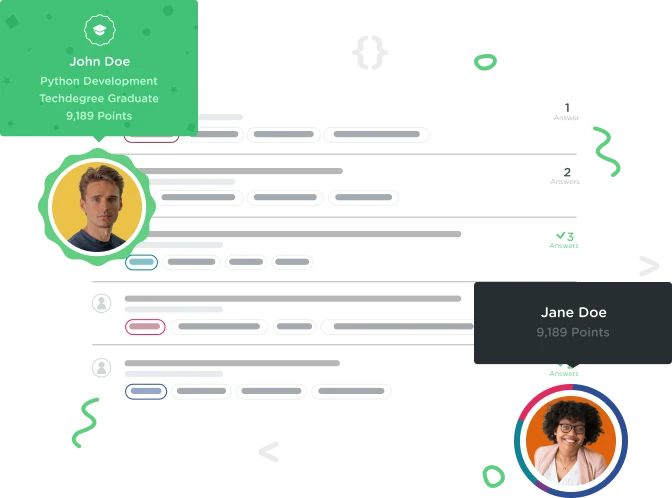
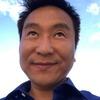
Martin Wilter
iOS Development Techdegree Student 8,782 PointsPossible bug
Won't compile in quiz, but runs fine in Playground.
There is also an issue on Line 20 | print("Do nothing! I'm a machine!"). Quiz compiler doesn't distinguish between " and '.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I\'m a machine!")
}
}
// Enter your code below
class Robot: Machine {
init(x: Int, y: Int) {
super.init()
}
override func move(direction: String) {
switch direction {
case "Up":
self.location.y += 1
case "Down":
self.location.y -= 1
case "Left":
self.location.x -= 1
case "Right":
self.location.x += 1
default:
break
}
}
}
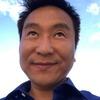
Martin Wilter
iOS Development Techdegree Student 8,782 PointsHi Chris,
Yes, seems like an issue with the display in the quiz editor. Even though everything after the ' has syntax color as comment I was able to pass the quiz after I removed the initializer.
Displays the same way in the quiz editor when I escape the double quotes.
1 Answer

Anjali Pasupathy
28,883 PointsI'm pretty sure your code won't compile because you have an init method in your subclass when you don't need one. Your init method takes in two integers, but then it calls super.init(), which does nothing with these integers. While this won't cause any compiler or runtime errors, you're still asking the user to input two values that have no impact on your code. If you were to instantiate Robot, you would find that no matter what values you use for x and y, the initial location is always Point(x: 0, y: 0).
let robot = Robot(x: -3, y: 42)
print("robot\'s location is (\(robot.location.x), \(robot.location.y)).")
There isn't anything technically erroneous about your code, besides the fact that x and y don't do anything in your init method; but because you're not adding any properties or changing any properties, you don't need this init method, so the quiz compiler doesn't expect to see it. I've found in my short time at Team Treehouse that when you feed the quiz compiler something it doesn't expect, even if your code does what you want it to do, the quiz compiler may still mark it wrong. It's a little annoying, but it's only to be expected when the quiz is designed to test you on not just whether or not you can make the code output what you want it to output, but whether or not you use a specific implementation to generate that output.
I hope this helps!
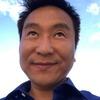
Martin Wilter
iOS Development Techdegree Student 8,782 PointsThanks Anjali for the good explanation. I was able to pass the quiz by removing the initializer as you indicated.
Quick follow-up question. Would this be the correct implementation if I want to be able to define x and y when I instantiate a Robot:
class Robot: Machine {
init(x: Int, y: Int) {
super.init()
self.location = Point(x: x, y: x)
}
override func move(direction: String) {
switch direction {
case "Up":
self.location.y += 1
case "Down":
self.location.y -= 1
case "Left":
self.location.x -= 1
case "Right":
self.location.x += 1
default:
break
}
}
}

Chris McKnight
11,045 PointsI agree. I would move the init method from Robot
to the Machine
class.

Anjali Pasupathy
28,883 PointsYou're welcome!
There doesn't appear to be anything functionally wrong with that implementation. If you wanted, you could even leave out the call to self in self.location, and just use location.
I, personally, would want to change the Machine init so you could specify the initial location for any Machine object, not just Robot objects. But that's my personal preference, because I find it strange to be able to specify the initial location of a Robot object, but not that of a Machine object. But if, for whatever reason, you chose not to change the Machine init method or found yourself unable to change the Machine init method, your implementation seems like a valid way to define x and y upon instantiation.
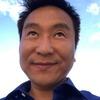
Martin Wilter
iOS Development Techdegree Student 8,782 PointsThanks again Chris and Anjali.
Chris McKnight
11,045 PointsChris McKnight
11,045 PointsPretty interesting.
Your code is valid but the escaping of the single quote is superfluous.
Have you tried
print("Do nothing! I'm a machine!")
? You don't need to escape single quotes when using double quotes but that could be an edge case in the challenge runner.