Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial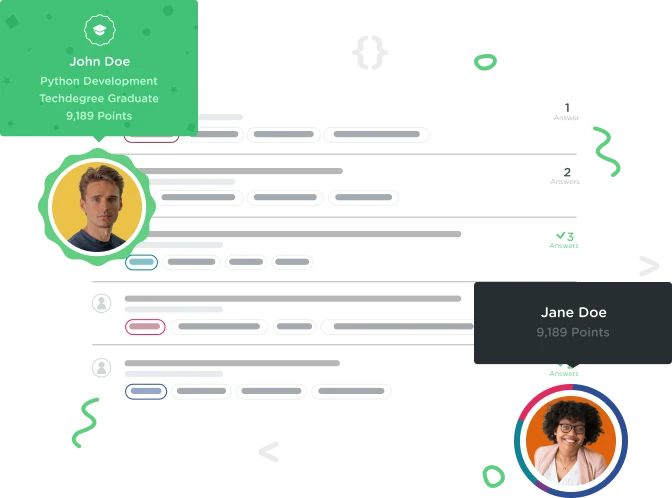
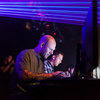
Adam Duffield
30,494 PointsPossible to return the string value of a key in php?
Hey All,
I have an array, I'll give you a similar example...
$search_keywords = array(
"Manikins" => array("Manikins", "Dummies","Training"),
"Signs" => array("Signage","Danger sign","Stuff we love to look at")
);
In this code I'm trying to extract the values where it says "Manikins & Signs as the keys if a value exists in the array.
E.g. If i type in my searchbar the word "Dummies" I want my code to give back to me the "Manikins" that the keyword "Dummies" is under.
I'm sure there will probably be someway to do this but unfortunately the treehouse courses I've done so far haven't covered this.
Cheers,
Adam
6 Answers
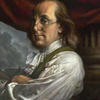
Jeff Busch
19,287 PointsHi Adam,
Maybe one of these two will be useful.
Jeff
<?php
function search_for($searchTerm) {
$search_keywords = array(
"Manikins" => array(
"Manikins2",
"Dummies",
"Training"),
"Signs" => array(
"Signage",
"Danger sign",
"Stuff we love to look at")
);
foreach ($search_keywords as $key => $value) {
foreach ($value as $theValue) {
/*** If $theValue equals search term ***/
if($theValue == $searchTerm) {
$searchFound = $theValue;
$searchFoundKey = $key;
}
}
}
if($searchFound = $searchTerm) {
printf("<br>Key is: %s | Value is: %s",$searchFoundKey, $searchFound);
} else {
printf("<br>Sorry, no match found.");
}
}
search_for("Dummies");
?>
<?php
$search_keywords = array(
"Manikins" => array(
"Manikins2",
"Dummies",
"Training"),
"Signs" => array(
"Signage",
"Danger sign",
"Stuff we love to look at")
);
$searchTerm = "Dummies";
foreach ($search_keywords as $key => $value) {
foreach ($value as $theValue) {
/*** If $theValue equals search term ***/
if($theValue == $searchTerm) {
$searchFound = $theValue;
$searchFoundKey = $key;
}
}
}
if($searchFound = $searchTerm) {
printf("<br>Key is: %s | Value is: %s",$searchFoundKey, $searchFound);
} else {
printf("<br>Sorry, no match found.");
}
?>
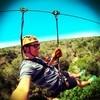
thomascawthorn
22,986 Pointskey() returns the index element of the current array position. If you scroll down to the examples, you should be able to see how it works. I'm not sure if you'll get similar results with a two dimensional array like you have.. Worth shot!
array_keys will allow you to search all the array keys and return the name of the key if successful.
array_search is probably exactly what you're looking for? Thought I'd find it last! But again, not sure how this works with multidimensional array, maybe this will point you in the right direction!
If you think one of these fits what you're looking for but you need more help, just hit us back :-)
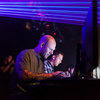
Adam Duffield
30,494 PointsI've been trying all three of those with no luck so far, I think the problem is the fact that it's a multidimensional array which makes things a bit more complicated, I'll give them all another try and see if I get any luck, I'll let you know.
Cheers,
Adam
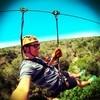
thomascawthorn
22,986 PointsWhat's the background to your code? I can try and recreate - love a challenge! Someone types a query in search bar and then the term is found in the bottom level array?
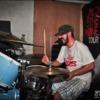
Mike Costa
Courses Plus Student 26,362 PointsHeres a quick solution I came up with but it only matches exact queries in the array. What's happening here is you have the $search_keywords array setup. You loop through it with a foreach loop, so $key will equal to all your keys, and $array is the inner array of that $key. You include an if statement to check if your query string exists inside of any of those arrays. If so, it will return that key and leave the function, but if no search term was found, it will reach the end of the function and return "No search results".
Being that this was a quick solution, I didn't account for multiple keys being returned (if "Dummies" existed in other arrays), or if part of the term existed in the inner array (searching for 'to look').
Hope this helps.
<?php
function search_term($query){
$search_keywords = array(
"Manikins" => array(
"Manikins2",
"Dummies",
"Training"),
"Signs" => array(
"Signage",
"Danger sign",
"Stuff we love to look at")
);
foreach($search_keywords as $key => $array){
if(in_array($query, $array)){
return $key;
}
}
return "No search results";
}
$key = search_term('Training');
?>
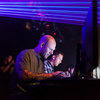
Adam Duffield
30,494 PointsCheers everyone for the help! Managed to find it out in the end thanks to Mr Jeff!
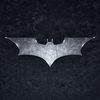
Logan R
22,989 PointsGlad you got it all sorted out :)