Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial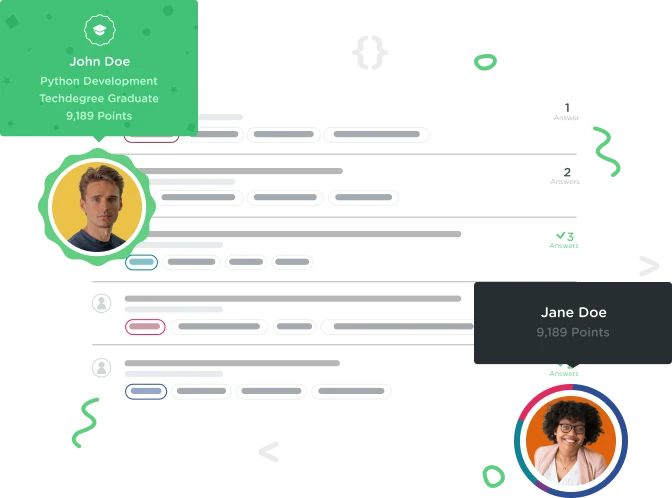

Randy Cole
Courses Plus Student 2,494 PointsPost and Pre Incrementing?
I'm a little confused on what is happening when post incrementing returns the same value then adds one to the value after. What affect does post or pre incrementing have on the code?
3 Answers

Aaron Arkie
5,345 PointsHey randy, Post incrementing means that the variable will increment after it is processed. This will take a copy of the variable and then add it to the next number when the compiler gets to this line of code. So lets say we have a line of code that say's i++. This translates to i = i+1. You see here that i needs to be recreated and then incremented by one.
Preincrement means the value will be incremented before it is processed. Generally both increments get the job done the same way. However, one requires a little less work and can save memory on heavy memory consuming programs. However, the performance gain is very little but just keep in mind that Postincrement duplicates the value in the variable then adds to it while pre increment just adds to what is already there.
Think of it like this:
Preincrement = (counting directly from 1 - 10) 1, 2, 3, 4, 5 ,6 ,7 8, 9, 10
Postincrement = (counting directly from 1 - 10) 1+1 = 2, 2+1=3, 3+1 =4, 4+1 = 5......
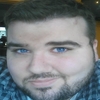
Marcus Parsons
15,719 PointsHi Randy,
You can really see how post and pre incrementing/decrementing variables works when you use arrays in Java. Let's say you have an array named mylist
that has a few decimal values. Let's also say you have a variable named index
that you are going to use to replace some values in this array. We are going to set the indices of the array to certain values depending on what the index is. Different behaviors occur when you use post and pre increment/decrement behaviors.
If you prefix the index variable with an increment/decrement, the index variable will go up by 1 (or down by 1) and then the compiler will use that value in the index
variable as the value for the index of the array. If you postfix the increment/decrement, the compiler will use the current value of index
for the index of the array and then increase/decrease.
//create array
double[] mylist = { 1.2, 2.4, 4.6 };
//create indexing variable
int index = 0;
//First let's prefix
//this causes index to be 1 and then replaces the value at index 1 with 7.2
mylist[++index] = 7.2;
//mylist is now { 1.2, 7.2, 4.6 }
//when you run the command below
//the current value of index is used which means that the index is 1
//and then index is increased and becomes 2
mylist[index++] = 9.8;
//mylist is now { 1.2, 9.8, 4.6 }
Hopefully now you can see how the post and pre increment/decrement work.
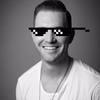
Seph Cordovano
17,400 PointsTo avoid all this over explanation, here's the skinny:
Post Increment or Post++ uses the current value of Post until the end of that exact statement. Then it uses the incremented version.
Pre Increment or ++Pre uses the incremented version of Pre right there in the current statement.
Most people just use pre incrementation unless it's a special case