Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial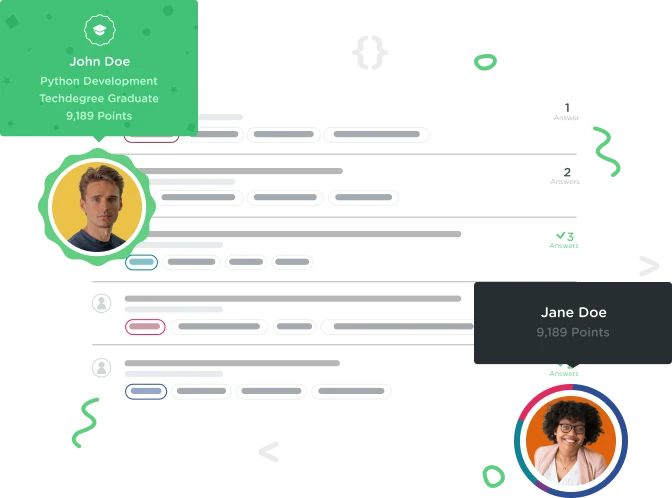

Muhammad Ali
6,752 PointsPost is not working
Response
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Error</title>
</head>
<body>
<pre>Cannot POST /qoutes</pre>
</body>
</html>
Code
const express = require('express');
const app = express();
const records=require('./records.js')
app.use(express.json());
app.get('/qoutes', (req, res) => {
records.getQuotes().then(
(qoutes) => {
res.json(qoutes)
}
);
})
app.get('/qoute/:id', (req, res) => {
records.getQuote(req.params.id).then((qoute) => {
res.json(qoute)
})
app.post('/qoutes', async (req, res) => {
const quote = await records.createQuote({
quote: req.body.quote,
author: req.body.author,
});
res.json(quote);
});
})
app.listen(3000, () => console.log('Quote API listening on port 3000!'));
1 Answer
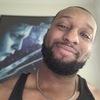
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsHi Muhammad,
If you could send a link to your complete repo, I could take a deeper look for you. But Iβm thinking the issue might be that youβre not requiring a module to parse the body so that you can check the request body for data.
If you think that might be the issue then check out this link. It explains how to use one of the more popular body parsers for Node and Express.