Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial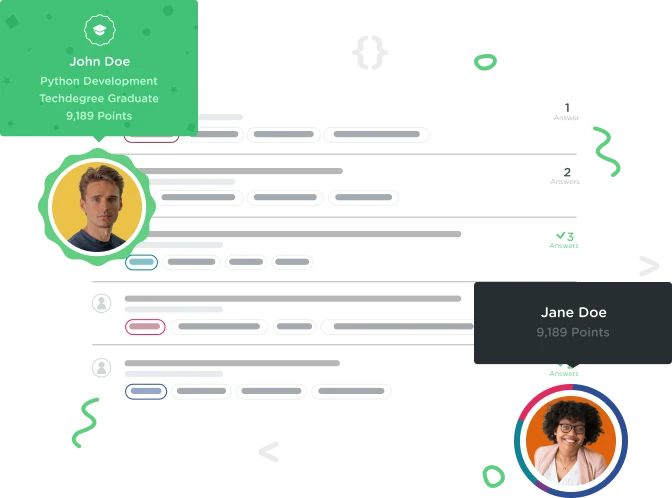

liamthornback2
9,618 PointsPost method for creating new question not working, app just freezes.
I've played around with my code using console.log and I've found that the question.save method is not working. The pre method is working and the question exist but it is not saving (is not added to the questions document) and the callback function is not running.
Does anyone have any idea what might have when wrong? Node doesn't show any errors in my code and the Get method works just fine from what I can see.
UPDATE
here is the code from my app.js file:
'use strict';
const express = require('express');
const app = express();
const routes = require('./routes');
const jsonParser = require('body-parser').json;
const logger = require('morgan');
app.use(logger('dev'));
app.use(jsonParser());
//Mongoose Database:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/qa');
const db = mongoose.connection;
db.on('error', (error) => {
console.error("connection error: ", error);
});
db.once('open', () => {
console.log("connection successful");
});
//api url routes
app.use('/questions', routes);
//catch 404 not found errors
app.use((req, res, next) => {
const error = new Error("Not Found");
error.status = 404;
next(error);
});
//custom json error handler
app.use((err, req, res, next) => {
res.status(err.status || 500);
res.json({
error: {
message: err.message
}
});
});
//port and listen
const port = process.env.PORT || 3000;
app.listen(port, () => {
console.log("working");
});
And here is the code from my routes.js file:
'use strict';
const express = require('express');
const router = express.Router();
const Question = require("./models").Question;
router.param("qID", (req, res, next, id) => {
Question.findById(id, (error, doc) => {
if(error) {
return next(error);
}
if(!doc) {
error = new Error("Not Found");
error.status = 404;
return next(error);
}
req.question = doc;
return next();
});
});
router.param("aID", (req, res, next, id) => {
req.answer = req.question.answers.id(id);
if(!doc) {
error = new Error("Not Found");
error.status = 404;
return next(error);
}
return next();
});
// ROUTES FOR QUESTIONS:
//returns all of the posted questions
router.get('/', (req, res, next) => {
Question.find({})
.sort({createdAt: -1})
.exec((error, questions) => {
if(error) {
return next(error);
}
res.json(questions);
});
});
//creating a new question
router.post('/', (req, res, next) => {
const question = new Question(req.body);
question.save(function(error, question) {
console.log('something');
if(error) {
return next(error);
}
res.status(201);
res.json(question);
});
});
//returns a specific quesiton
router.get('/:qID', (req, res, next) => {
res.json(req.question);
});
// ROUTES FOR ANSWERS:
//creating an answer
router.post('/:qID/answers', (req, res, next) => {
req.question.answers.push(req.body);
req.question.save((error, question) => {
if(error) {
return next(error);
}
res.status(201);
res.json(question);
});
});
//editing an answer
router.put('/:qID/answers/:aID', (req, res, next) => {
req.answer.update(req.body, (error, result) => {
if(error) {
return next(error);
}
res.json(result);
});
});
//deleting an answer
router.delete('/:qID/answers/:aID', (req, res, next) => {
req.answer.remove((error) => {
if(error) {
return next(error);
}
req.question.save((error, question) => {
if(error) {
return next(error);
}
res.json(question);
});
});
});
//voting on an answer
router.post('/:qID/answers/:aID/:vote',
(req, res, next) => {
if(req.params.vote.search(/^(vote-up|vote-down)$/) === -1) {
const error = new Error("Not Found");
error.status = 404;
next(error);
} else {
req.vote = req.params.vote;
next();
}
},
(req, res, next) => {
req.answer.vote(req.vote, (error, question) => {
if(error) {
return next(error);
}
res.json(question);
});
});
module.exports = router;

liamthornback2
9,618 PointsOkay I've updated my post. I hope that provides enough information.

liamthornback2
9,618 PointsOkay this post was from almost a month ago. I've been working on other content in the mean time, but It would be great to be able to just finish this course. If anyone can see what I did wrong that would be great.

Putra Aryotama
36,050 PointsHi Liam! does this error still persist? Can we see your code in models.js?
1 Answer

Mike Hardy
Full Stack JavaScript Techdegree Graduate 16,511 PointsIt definitely sounds like a piece of middleware is missing a next();
I'd check the models.js file, specifically the QuestionSchema.pre(); That specific chunk has to do with question.save()
Shlomi Bittan
6,718 PointsShlomi Bittan
6,718 PointsHi Liam, I want to help you, but i need to see the code.