Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial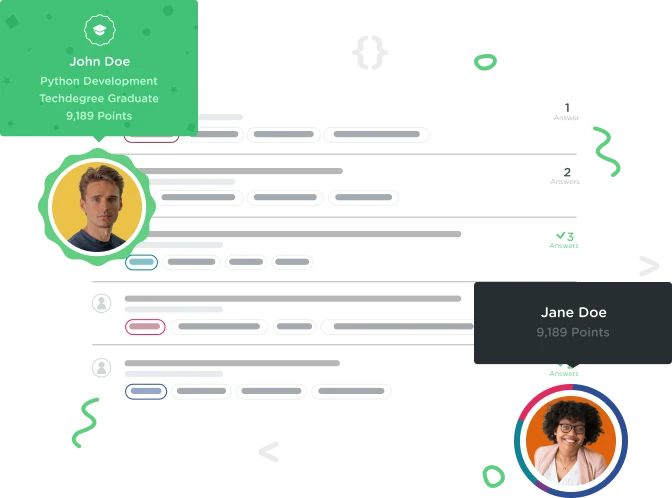
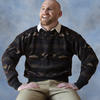
Kevin Kenger
32,834 PointsPOST to user_friendships_path causes 422 (Unprocessable Entity)
Initially, when I load the profile page, clicking the "Add Friend" button opens the new user friendship page – which is its default action – even though we specify in the user_friendships.js file that we want to prevent this.
user_friendships.js
$(document).ready(function () {
$("#add-friendship").click(function(event) {
event.preventDefault();
var addFriendshipBtn = $(this);
$.ajax({
url: Routes.user_friendships_path({user_friendship: { friend_id: addFriendshipBtn.data("friendId") }}),
dataType: "json",
type: "POST",
success: function(e) {
addFriendshipBtn.hide();
$("#friend-status").html("<a href='#' class='button'>Friendship Requested</a>");
}
});
});
});
user_friendships_controller.rb
class UserFriendshipsController < ApplicationController
before_filter :authenticate_user!
respond_to :html, :json
def index
@user_friendships = current_user.user_friendships.all
end
def accept
@user_friendship = current_user.user_friendships.find(params[:id])
if @user_friendship.accept!
flash[:success] = "You are now friends with #{@user_friendship.friend.first_name}."
else
flash[:error] = "That friendship could not be accepted."
end
redirect_to user_friendships_path
end
def new
if params[:friend_id]
@friend = User.where(profile_name: params[:friend_id]).first
raise ActiveRecord::RecordNotFound if @friend.nil?
@user_friendship = current_user.user_friendships.new(friend: @friend)
else
flash[:error] = "Friend required"
end
rescue ActiveRecord::RecordNotFound
render file: "public/404", status: :not_found
end
def create
if params[:user_friendship] && params[:user_friendship].has_key?(:friend_id)
@friend = User.where(profile_name: params[:user_friendship][:friend_id]).first
@user_friendship = UserFriendship.request(current_user, @friend)
respond_to do |format|
if @user_friendship.new_record?
format.html do
flash[:error] = "There was a problem creating that friend request."
redirect_to profile_path(@friend)
end
format.json { render json: @user_friendship.to_json, status: :precondition_failed }
else
format.html do
flash[:success] = "Friend request sent."
redirect_to profile_path(@friend)
end
format.json { render json: @user_friendship.to_json }
end
end
else
flash[:error] = "Friend required"
redirect_to root_path
end
end
def edit
@user_friendship = current_user.user_friendships.find(params[:id]).decorate
@friend = @user_friendship.friend
end
def destroy
@user_friendship = current_user.user_friendships.find(params[:id])
if @user_friendship.destroy
flash[:success] = "Friendship destroyed."
end
redirect_to user_friendships_path
end
end
After I refresh the page, that no longer happens, and when I click the "Add Friend" button, nothing appears to happen (which I'm assuming means the "event.preventDefault();" code is working) and I get this error in the console:
Chrome console
POST http://localhost:3000/user_friendships?user_friendship%5Bfriend_id%5D=kevin 422 (Unprocessable Entity) jquery.js?body=1:27
Z.cors.a.crossDomain.send jquery.js?body=1:27
ab.extend.ajax jquery.js?body=1:26
(anonymous function) user_friendships.js?body=1:6
ab.event.dispatch jquery.js?body=1:26
q.handle jquery.js?body=1:25
Any help is greatly appreciated!
3 Answers

Naomi Freeman
Treehouse Guest TeacherThere's this: http://restpatterns.org/HTTP_Status_Codes/422_-_Unprocessable_Entity
So it sounds like things are correct and it's just rejecting it. Rails 4 strong params might cause that if they're not configured.
What I see missing in your code that my code has is the replacement for attr_accessible Are you in Rails 4?
You may need these at the bottom of your controller:
def friendships_params
params.require(user_friendship).permit(:user_id, :friend_id, :user, :friend, :state, :first_name, :user_friendship, :full_name, :last_name)
end
This chunk of code says "here are the things I will allow through to my server"
Do you have similar params in your other controllers?

Naomi Freeman
Treehouse Guest TeacherHmm ... well, feel free to look at my controller and see if you can find the difference. https://github.com/summerspirit/laughing-wookie/blob/master/app/controllers/user_friendships_controller.rb
That's the completely finished product though.
One interesting thing to note is that, when I tried to push it live, the add friendships button wouldn't work because I hadn't configured Devise mailers. It was fine when it was local but not live. Now that I'm starting to configure them correctly though, it's causing problems.
I haven't figured out the mailers piece yet. I wonder if it might be tripping you up too.
Let me know how it goes. I'm prepping for an open data project tonight, but will have more time on the weekend to try and debug code if it's still not working for you.
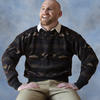
Kevin Kenger
32,834 PointsWell thank you so much. I couldn't find any differences in our controllers (obviously other than the friendship_association method).
I had actually thought about the mailer messing me up before – just because I've been doing so much reading about this and came across a few places where that was suggested – but I'm pretty sure that only occurs once the site is live, depending on where you deploy your application. I know Heroku for instance, doesn't have an email engine so you have to use one of their add-ons like Postmark.
But I'll keep chopping away at it, and I'll let you know when I finally figure it out. I appreciate the debugging offer by the way, there's a good chance I'll need to take you up on that. In the mean time thank you for the help, and good luck with your open data project!
Kevin Kenger
32,834 PointsKevin Kenger
32,834 PointsThank you for the reply!
Yeah I'm in Rails 4 and using strong params, but I added the friendships_params method to my controller to no avail. Unfortunately I'm still getting the same error that I was before :(