Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial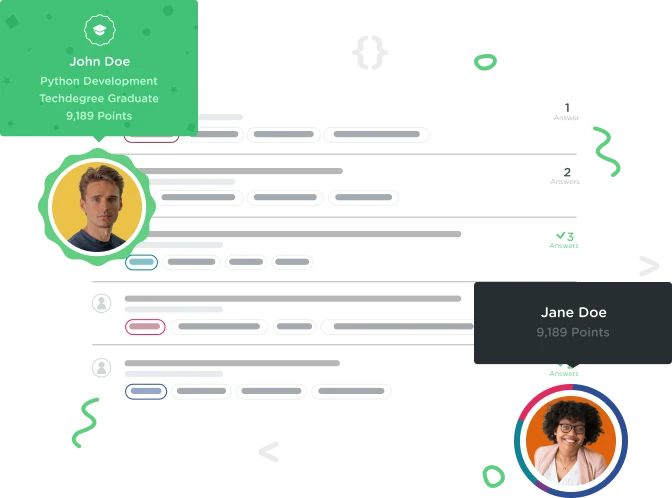
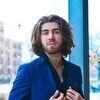
Ali Colak
2,192 PointsPractice Creating and Using Functions in Python
What do I have to do to get this to work? I'm a little lost.
num = int(input("Put in a number: "))
def is_odd(num):
if is_odd % 2 != 0:
return false
else:
return true
2 Answers
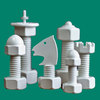
Steven Parker
230,274 PointsThere are several issues, here are some hints:
- the name of the function is "is_odd", it should not be referenced inside the function code
- you will need to test the value of the function argument, which is "num"
- this challenge asks you only to construct the function, you won't need any code outside the function
- you won't need to
input
anything - the function needs to return True when the argument is not divisible by 2
- the Python values of "True" and "False" both start with a capital letter
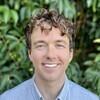
Asher Orr
Python Development Techdegree Graduate 9,408 PointsHi Ali!
When you click "Check Work" on a Treehouse challenge, a program will run your code. It will replace your function's parameter with a specific argument.
Here's what I mean:
Let's say we wrote this code for a Challenge Task.
def name_function(name):
print("Hello {}".format(name))
You don't need to define the "name" parameter before. When you click "Check Work," Treehouse will run this code under the hood. It might look like this:
name_function("Ali")
In which case, the checker would see the string "Hello Ali!"
It can confuse the checker to define a function argument beforehand, so I would avoid that (unless you're specifically instructed to.)
So:
num = int(input("Put in a number: "))
#remove the line of code above.
def is_odd(num):
if is_odd % 2 != 0:
return false
else:
return true
Now, let's get into your function. First:
def is_odd(num):
if is_odd % 2 != 0:
# you want the if statement to check the num- not the function is_odd.
It should look instead like this:
def is_odd(num):
if num % 2 != 0:
Also, think about the logic in your function:
if num % 2 != 0:
return False
# side note: If you return True or False, make sure to capitalize the first letter.
# otherwise, Python will think you're referring to a variable named "true" or "false"
As Stephen said, the function needs to return True when the argument is not divisible by 2.
I hope this helps. Let me know if you have any questions!