Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial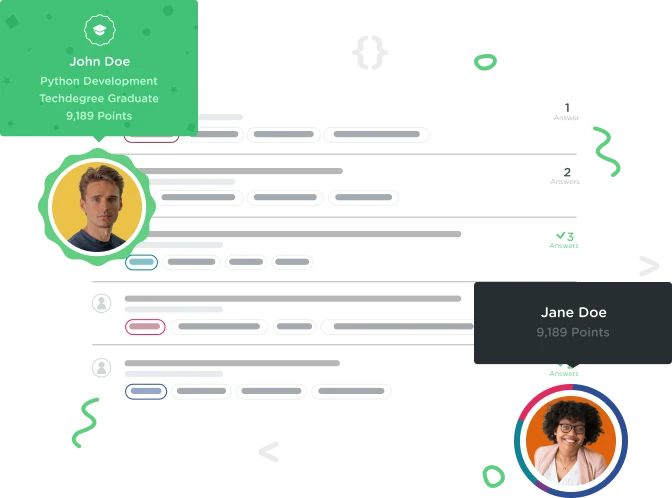
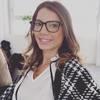
Chloé Claes
19,451 PointsPractice Getters and Setters in JavaScript: Create a setter method called radius inside the Circle class.
I have a question about the following code challenge:
"Challenge Task 1 of 2 Create a setter method called radius inside the Circle class. This method should store the value of the radius using a backing property, _radius. It should also set two additional properties on the Circle class, area, and circumference. These do not need to be backing properties. For the purposes of this challenge, use 3.14 to represent π. The formula for circumference is circumference = 2*π*radius, the formula for area is area = π*r^2. "
The more I read this challenge the more confused I get. Most exercises are clear and obvious but this one has been worded in such a confusing way that I don't understand what it is that you are asking.
I'm pretty sure that it's going to be something small and obvious, but at the moment i'm just not seeing it. I'll keep trying but maybe this challenge should be phrased differently.
Kind regards, Cc
7 Answers
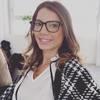
Chloé Claes
19,451 PointsMother of frustration but I figured it out! This challenge should be described and formulated better.
class Circle {
set radius(radius){
this._radius = radius;
this.area = 3.14 * Math.pow(radius, 2);
this.circumference = 2*(3.14)*radius;
}
}
const circ = new Circle();
this is also valid for area:
this.area = 3.14 * radius * radius;
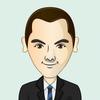
stjarnan
Front End Web Development Techdegree Graduate 56,488 PointsGood job figuring it out!
I might have to agree with you regarding the description. It could probably be explained in a more simple way.
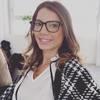
Chloé Claes
19,451 PointsHa, glad to hear you agree. ^_^
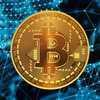
Yannis Bouacida
9,169 PointsThank you! i couldn't figure it out but now i understand :)

Matt Fredericks
5,399 PointsCan someone please change the challenge description to make it clearer as previously requested. I found it confusing as well. Something a bit more verbose. For example, should we just assume r means radius in the area formula? It is spelled out 'radius' in every preceding line.

Óscar Hernández sandoval
9,257 PointsMaybe you are confuse with π = 3.14 pie, observe very well.

vamosrope14
14,932 PointsWhy do we not need a constructor in this scenario? Are we allowed to just have getters and setters without constructors?
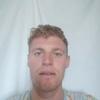
Ashley Campbell
Front End Web Development Techdegree Graduate 21,004 Pointshi everyone! i've also just spent an hour staring at this challenge. Glad i am not alone! Should definitely be worded better!

Óscar Hernández sandoval
9,257 PointsI did it in this way class Circle { set radius(radius){ this._radius = radius; this.circumference = 2 * 3.14 * radius ; this.area = 3.14*100;
}
}
const circ = new Circle(5); circ.radius = 10;
claude
Front End Web Development Techdegree Graduate 20,452 Pointsclaude
Front End Web Development Techdegree Graduate 20,452 PointsAgree...
luther wardle
Full Stack JavaScript Techdegree Student 18,029 Pointsluther wardle
Full Stack JavaScript Techdegree Student 18,029 Pointsthanks so much! this worked perfectly, yes the description was a little disorienting to me too lol
Faiz Hamdan
5,215 PointsFaiz Hamdan
5,215 PointsOw! Mother of frustration Feisty!! Hahahahah! I assume the reason why they put it this way is for us to refer line by line. Imagine if it were displayed differently, it can be anything. There are 1001 ways to do it. If you were to read carefully, it's like showing us how to do it in sequence. Like for example :
1). Create a setter method 2). Store value of the radius using backup property. 3). It should also set two additional properties on the Circle class, area, and circumference. (This can be anywhere inside the class Circle) but the key sentence is "These do not need a backing property" meaning its inside of the setter method not outside like how we do with constructor.
4). use 3.14 to represent π 5). The formula for circumference is circumference = 2*π*radius 6). the formula for area is area = π*r^2.
All we have to do now is, to produce the syntax and calculate the Math itself. Using the information in MDN.
To conclude everything, they want us to practice the syntax from creating a class, to getter and setter method.
Rebeckah McDaniel
8,566 PointsRebeckah McDaniel
8,566 PointsThank you so much! That makes perfect sense now! Something as simple as still adding this in front of the area and circumference. It was worded strangely to me as well, but they would be stored in the setter method without the backing property.