Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial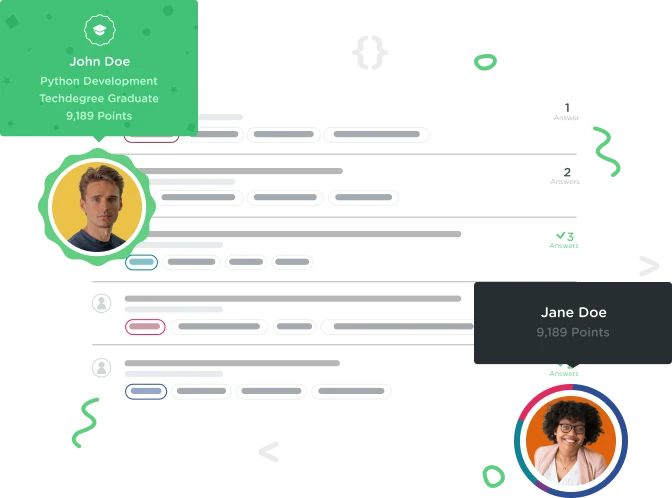

Anna Gibson
Front End Web Development Techdegree Student 4,468 PointsPractice Object literals-How do I access a global object within a function?
These are my instructions:
Create a function named addInventory(). The function should accept 2 parameters -- the product object, and the number to add to the inventory. The function adjusts the product object's inventory property by adding the number passed into the function. For example, if the inventory of the product is currently 2, calling addInventory(product, 3) will set the value of inventory to 5. Add a console.log() message to the function that includes a message that looks something like this: "3 chairs added to the inventory" In this example, "chair" is the name of the product.
var product = {
name: "SkullCandy Headphones",
inventory: 6,
unit_price: 49.99
}
addInventory(product, numOfProducts) {
this.inventory + numOfProducts
console.log(numOfProducts + this.name + 'added to inventory');
}
addInventory(product, 6);
What could I be doing wrong? I'm trying to access what seems to be a global variable, and the function is OUTSIDE the object, so I'm not sure if I'm supposed to be using 'this'. It's just my best guess at this point. Is there any valuable info you can provide me to help me out here? Atm it's outputting a bunch of module information when I log it to the console.
1 Answer
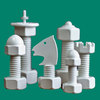
Steven Parker
231,007 PointsYou don't need to be concerned about anything global (or not), since the object the function is working with is passed as an argument.
And the object should be referenced inside the function by using the parameter name. The term "this" is for when you reference an object from within its own methods.
And you need to start the function definition with the word "function".