Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial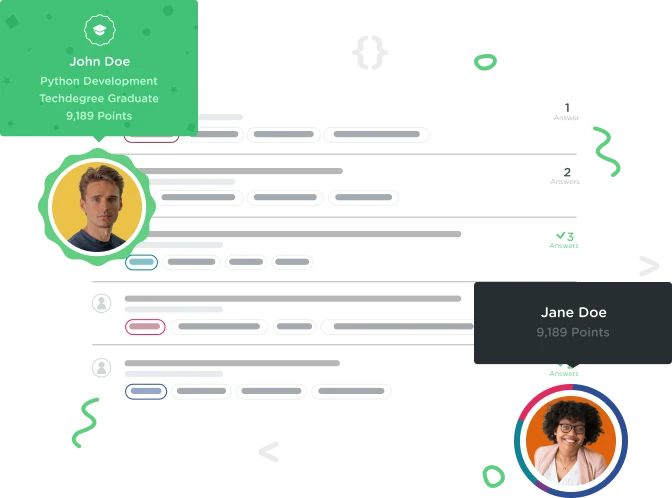
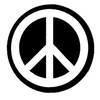
john larson
16,594 PointsPracticing managing keys came across "bool is not iterable"
So I looked it up and found a post about how a bool needs to be converted to a string to be iterated. After that I noticed that I was using .values(), instead of .items(). I fixed that and now my bool iterates fine. Any clarification would be great. Here is a snipet of my code that works as intended.
def employed(job):
employed = input("emplyed in {}? Y/n ".format(job)).lower()
if employed == "y":
employed = "employed as {} is {}".format(job, True)
else:
employed = "employed as {} is {}".format(job, False)
return employed
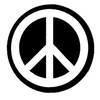
john larson
16,594 PointsHi Jason, I guess I was all over the place there. I just was curious about bool not being iterable. Cause now that I fixed my for loop, bool seems to iterate fine.
me = {"Name": name, "Job": job, "Employed": employed}
for key, value in me.items():
print(key, value)
It is just confusing cause I found a post on stack overflow that says bool is not iterable, and to fix it by converting it to a string. But my code works fine without converting bool to a string. There must be some nuance I'm missing.

Jason Anello
Courses Plus Student 94,610 PointsWhat code did you have that produced the 'bool is not iterable' message?
I'm still not seeing where you're trying to iterate over a bool.
You're iterating over a dictionary here.
It is true that you can't iterate over things like bool's and int's
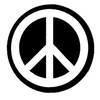
john larson
16,594 Pointswhen I had my for loop as:
for key, value in me.values():
I got the error of "bool" is not iterable. Which I can see in insight that was not my problem. I was using values() instead of items(). But it looks to me like I'm iterating over bool? Because True and False are in job. And I'm looping over the dict me, that holds the var employed, that holds the bool True or False?
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsI think there must have been some other code that produced your error.
I get a different error when I try the following:
>>> me = {"Name": "Joe", "Job": "janitor", "Employed": True}
>>> for key, value in me.values():
... print(key, value)
...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: too many values to unpack (expected 2)
Anyways, in the working version you're iterating over a dictionary. One of the values happens to be a boolean but that's not considered iterating over a boolean.
Here's an example of trying to iterate over a bool and also an int and you get similar error messages as what you posted.
>>> for index in True:
... print(index)
...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'bool' object is not iterable
>>> for index in 10:
... print(index)
...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not iterable
I'm not saying that's what you did but you must have been using a boolean value somewhere that an iterable was expected in order to get 'bool' object is not iterable
error
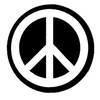
john larson
16,594 PointsThanks Jason. I think I understand that, as long as the Boolean isn't directly in the dict..it's ok. Since the var employed only HOLDS the bool, it's ok? I can see the the example above: for index in True: How that would be trying to iterate a bool. That doesn't look like a mistake I would be tempted to do. Thanks for you help:D

Jason Anello
Courses Plus Student 94,610 PointsIf I understand correctly, yes you can have a literal boolean directly in there. It doesn't have to be in a variable.
The example I gave has this. I didn't use your employed
variable. I put True directly in there.
me = {"Name": "Joe", "Job": "janitor", "Employed": True}
Let me know if I've misunderstood the question.
I don't know if this will help but you want to distinguish between iterating over a boolean and iterating over an iterable which contains boolean values.
This is attempting to iterate over a boolean and will lead to an error:
>>> for value in True:
... print (value)
...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'bool' object is not iterable
This is iterating over a list which happens to contain boolean values for each item and will work fine:
>>> for value in [True, True, False, True]:
... print (value)
...
True
True
False
True
It's ok whether or not you have literal boolean values in there or you have variables which are storing the boolean values.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi John,
I'm not sure about your question. Did you want to know why the non-working code didn't work? You didn't post what it was.
You mention .values() and .items() but I don't see that in the code.