Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial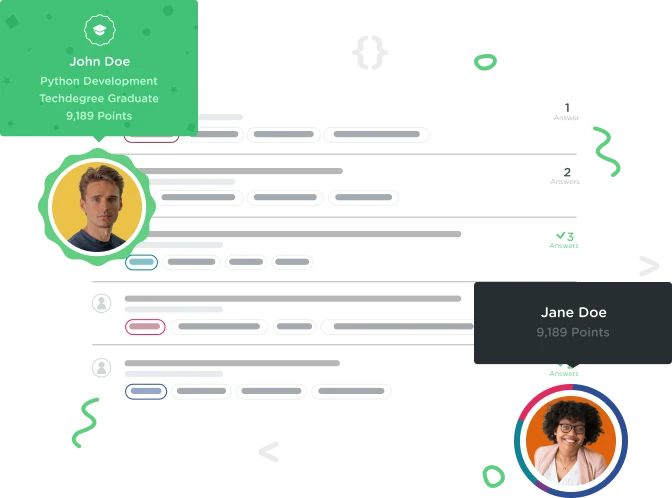
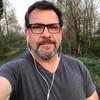
Scott George
19,060 PointsPracticing type conversion
Hi guys! Seems I'm only stuck on a small detail, I've gone over the video again, but not getting it yet. Thanks
using System;
class Program
{
// YOUR CODE HERE: Define a Divide method!
static double divide(firstNum, secondNum){
int wholeNumber = (int)5.0;
double fractionalNumber = 2.0;
Console.WriteLine(wholeNumber.GetType());
Console.WriteLine(fractionalNumber.GetType());
return;
}
static void Main(string[] args)
{
// This should print "2.5".
Console.WriteLine(Divide(5, 2));
// This should print "3.3333333333..."
// (Or a value close to that, since it can't be
// infinitely precise.)
Console.WriteLine(Divide(10, 3));
}
}
1 Answer
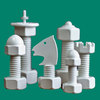
Steven Parker
231,269 PointsIt looks like there are a few issues:
- the parameter types must be declared with their names ("
(int firstNum, int secondNum)
") - the method name should be "Divide" (with a capital "D") instead of "divide"
- the parameters should be used in the calculation
- you won't need to create any new variables
- the final result must be returnβed
- you won't need to output ("WriteLine") anything
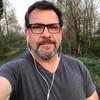
Scott George
19,060 PointsHey Steven, just got to update my code
using System;
class Program {
// YOUR CODE HERE: Define a Divide method!
static double Divide(int param1 2.5 , int param2 2.0)
{
int param1 = (int) 2.5;
double param2 = 2.0;
return(Divide);
}
static void Main(string[] args)
{
// This should print "2.5".
Console.WriteLine(Divide(5, 2));
// This should print "3.3333333333..."
// (Or a value close to that, since it can't be
// infinitely precise.)
Console.WriteLine(Divide(10, 3));
}
}
The only error I'm getting now is " Program.cs(7,37): error CS1003: Syntax error, ',' expected [/workdir/workspace.csproj] Program.cs(7,54): error CS1003: Syntax error, ',' expected [/workdir/workspace.csproj] "
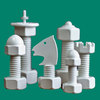
Steven Parker
231,269 PointsSeveral issues still need to be addressed, but now there's some new causes:
- the parameter declarations should only have types and names (like "
(int firstNum, int secondNum)
") - the parameters should still be used in the calculation
- you won't need to create any new variables (particularly not ones with the same names as the parameters)
- the final calculation result must be returnβed (not the method itself)
You may also want to review method syntax in the video before trying again.
Scott George
19,060 PointsScott George
19,060 PointsForgot to add the error I'm getting. "error CS1001: Identifier expected"