Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial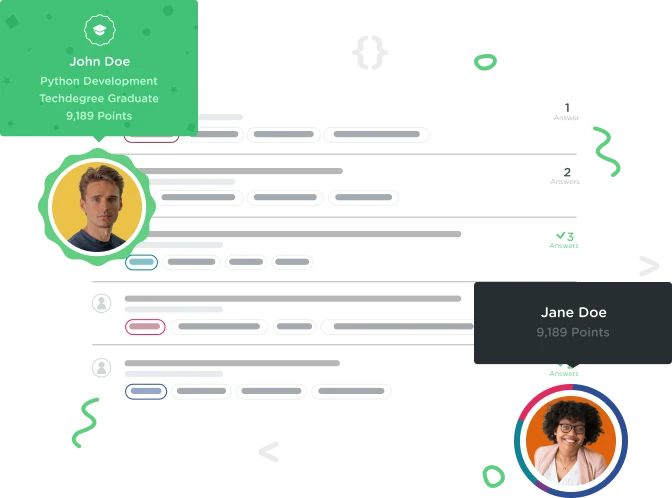
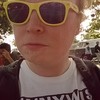
Jeremy O'Bryan
16,672 PointsPresidents Game (Python)
Hey Everyone,
I'm a bit stuck on this project... What I'm ultimately trying to do is create a game in which the player will be prompted with the name of a U.S. President, and they will need to enter that presidents' corresponding number (1 for George Washington, 2 for John Adams, etc). Where I'm stuck is trying to associate the specific name with their corresponding number from the list. Here's what I have so far:
import random
import os
import sys
presidents = ["Donald Trump" , "Barack Obama" , "George W. Bush" , "Bill Clinton" , "George H.W. Bush" , "Ronald Reagan" , "Jimmy Carter" , "Gerald Ford" , "Richard Nixon" , "Lyndon B. Johnson" , "John F. Kennedy" , "Dwight D. Eisenhower", "Harry S. Truman" , "Franklin D. Roosevelt" , "Herbert Hoover" , "Calvin Coolidge" , "Warren G. Harding" , "Woodrow Wilson" , "Howard Taft" , "Theodore Roosevelt" , "William McKinley" , "Grover Cleveland" , "Benjamin Harrison" , "Grover Cleveland" , "Chester A. Arthur" , "James Garfield" , "Rutherford B. Hayes" , "Ulysses S. Grant" , "Andrew Johnson" , "Abraham Lincoln" , "James Buchanan" , "Franklin Pierce" , "Millard Fillmore", "Zachary Taylor" , "James K. Polk" , "John Tyler" , "William Henry Harrison" , "Martin Van Buren" , "Andrew Jackson" , "John Quincy Adams" , "James Monroe" , "James Madison" , "Thomas Jefferson" , "John Adams" , "George Washington"]
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
randomname = random.choice(presidents)
number = presidents.index(randomname)
def namegame():
while True:
print("Input the corresponding president's number or press n to quit")
answer = input(randomname + ": ")
if answer == "n":
break
elif answer == number:
print("Yay! You got it!")
clear_screen()
elif answer != number:
print("Nope, that's not it! Try again")
elif answer != number or "n":
print("I don't understand")
namegame()
I'm not sure if a list is the solution here, or if I should be using a dictionary (I think that might be the way to go, possibly keying each name to their individual number?). Also, the indexing is throwing me off, as The presidents' names are in order in the list starting with the most recent (and of course the indexing starts with 0, which is also an issue). I'm also having trouble with the conditional that prints "I don't understand" if the player inputs anything other than a number or "n" to quit. This is turning out to be a lot harder than I thought! Any help would be appreciated!
3 Answers
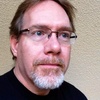
Chris Freeman
Treehouse Moderator 68,423 PointsFirst, with randomname
and number
defined outside of the game loop, there values will not change. Move these assignments inside the while
loop so they change each game.
As for the number and president association, there are two ways
- use a dict where the keys are the "numbers" and the values are the president names
- use a list with the index is the presidents number. Skipping 0, of course:
presidents = [None, 'George Washington', 'John Adams', 'Thomas Jefferson', 'James Madison', 'James Monroe', 'John Quincy Adams', 'Andrew Jackson', 'Martin Van Buren', 'William Henry Harrison', 'John Tyler', 'James K. Polk', 'Zachary Taylor', 'Millard Fillmore', 'Franklin Pierce', 'James Buchanan', 'Abraham Lincoln', 'Andrew Johnson', 'Ulysses S. Grant', 'Rutherford B. Hayes', 'James Garfield', 'Chester A. Arthur', 'Grover Cleveland', 'Benjamin Harrison', 'Grover Cleveland', 'William McKinley', 'Theodore Roosevelt', 'Howard Taft', 'Woodrow Wilson', 'Warren G. Harding', 'Calvin Coolidge', 'Herbert Hoover', 'Franklin D. Roosevelt', 'Harry S. Truman', 'Dwight D. Eisenhower', 'John F. Kennedy', 'Lyndon B. Johnson', 'Richard Nixon', 'Gerald Ford', 'Jimmy Carter', 'Ronald Reagan', 'George H.W. Bush', 'Bill Clinton', 'George W. Bush', 'Barack Obama', 'Donald Trump']
For the list to work, you will have to add the repeated presidencies to their respective positions so the index will be the desired value.
Interesting project. Good luck!!
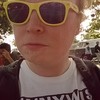
Jeremy O'Bryan
16,672 PointsThanks everyone, that helped. My game is progressing nicely. I decided to go with a dictionary instead of a list and added some error checking, and it is working for the most part:
import random
import os
import sys
presidents = {45: "Donald Trump" , 44: "Barack Obama" , 43: "George W. Bush" , 42: "Bill Clinton" , 41: "George H.W. Bush" , 40: "Ronald Reagan" , 39: "Jimmy Carter" , 38: "Gerald Ford" , 37: "Richard Nixon" , 36: "Lyndon B. Johnson" , 35: "John F. Kennedy" , 34: "Dwight D. Eisenhower", 33: "Harry S. Truman" , 32: "Franklin D. Roosevelt" , 31: "Herbert Hoover" , 30: "Calvin Coolidge" , 29: "Warren G. Harding" , 28: "Woodrow Wilson" , 27: "Howard Taft" , 26: "Theodore Roosevelt" , 25: "William McKinley" , 24: "Grover Cleveland" , 23: "Benjamin Harrison" , 22: "Grover Cleveland" , 21: "Chester A. Arthur" , 20: "James Garfield" , 19: "Rutherford B. Hayes" , 18: "Ulysses S. Grant" , 17: "Andrew Johnson" , 16: "Abraham Lincoln" , 15: "James Buchanan" , 14: "Franklin Pierce" , 13: "Millard Fillmore", 12: "Zachary Taylor" ,11: "James K. Polk" , 10: "John Tyler" , 9: "William Henry Harrison" , 8: "Martin Van Buren" ,7: "Andrew Jackson" , 6: "John Quincy Adams" , 5: "James Monroe" , 4: "James Madison" , 3: "Thomas Jefferson" , 2: "John Adams" , 1: "George Washington"}
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def namegame():
choice = None
while choice != "q":
try:
randomname = random.choice(list(presidents.values()))
print("Welcome to the presidents game! Try to correctly guess the number that is associated with the name of the President that you are prompted with. They are ordered from first to current \n")
print("Input the corresponding president's number, or press 'q' to quit")
choice = input(randomname + ": ")
if randomname == presidents[int(choice)]:
input("Yay! You got it! (press any key to continue)")
clear_screen()
else:
print("Nope, try again")
except (KeyError, ValueError):
if choice != "q":
print("I don't understand")
continue
except KeyboardInterrupt:
break
namegame()
However, I want to be able to have the player keep guessing until they get it right (I plan on adding strikes / scoring later) . The way it's written now, they player will be given a new President if they get their answer wrong as well as if they get it right. Any ideas? I was thinking perhaps to append each answer to a list? That way I can also work in avoiding duplicates in a given play session, but I'm not sure.
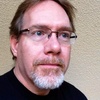
Chris Freeman
Treehouse Moderator 68,423 PointsSince the loop keeps the gaming going, you could move the random selection outside of the while
loop, then the president will stay the same until guessed. If they guess it correctly, you could call namegame()
to start the game over.
If you don't want repeats, you can pop()
the random value from the dictionary, then is will not be available until the program restarts.
Oszkár Fehér
Treehouse Project ReviewerOszkár Fehér
Treehouse Project ReviewerHi Jeremy, If u don't need random number for the names than dict it's better, for indexing u can try -1 from the index, but i still suggest dict(). but even there u can add a random generator witch generates number from a set(), set() numbers doesen't repeat, like this any name can have his own unic number(check out the random library, it's a lot of cool stuff there there). For the conditional there is methods witch can check if the user input it's a number or string and wrapped with try and except blocks. I am sorry for a short answer, but if you still have questions, i will do my best and take a little extra time to help you out. Happy codding( and i am sorry for the gramma ,it's my third language)