Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial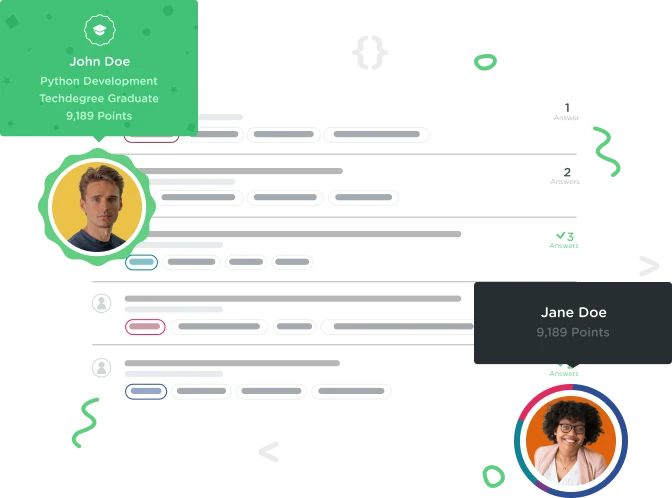

Joseph Bachaalany
4,007 Pointspreventing same color to appear twice in a row
How to prevent the same background color and text to appear twice in a row? I mean when you press on the button sometimes the text or color will stay the same.
3 Answers

Ben Deitch
Treehouse TeacherHey Joseph! You could store which color the background currently is, and then when picking a new background color, use a while loop to keep picking a new color until you get a different one. Hope that helps!

Kresimir Fijacko
6,025 Pointstry this code
public class FunFactsActivity extends AppCompatActivity {
// Declare our view variables
private TextView factTextView;
private Button showFactButton;
private RelativeLayout relativeLayout;
private int currentColor;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Assign the views from the layout file to the corresponding variables
factTextView = findViewById(R.id.factTextView);
showFactButton = findViewById(R.id.showFactButton);
relativeLayout = findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
ColorWheel colorWheel = new ColorWheel();
int newColor;
do {
newColor = colorWheel.GetColor();
} while (newColor == currentColor);
currentColor = newColor;
// Update the screen with our new fact
factTextView.setText(FactBook.GetFact());
relativeLayout.setBackgroundColor(newColor);
showFactButton.setTextColor(newColor);
}
};
showFactButton.setOnClickListener(listener);
}
}

ROBERT RUBY II
10,586 PointsTo do this I created a class called ColorGenerator-- Pasted code below.
package com.example.bobby.funfacts;
import android.graphics.Color;
import java.util.Random;
class ColorGenerator {
// Fields or Member Variables - Properties about the object
int r = 0;
int g = 0;
int b = 0;
int maxNum = 255;
// Methods
int getColor(){
// Create random r,g,b values
Random randomGen = new Random();
r = randomGen.nextInt(maxNum);
g = randomGen.nextInt(maxNum);
b = randomGen.nextInt(maxNum);
// Get a hex value from r,g,b
String hexColor = String.format("#%02x%02x%02x", r, g, b); // see https://stackoverflow.com/questions/3607858/convert-a-rgb-color-value-to-a-hexadecimal
// Get int based color in intColor from hexColor
int intColor = Color.parseColor(hexColor); // see https://stackoverflow.com/questions/6935057/convert-hex-color-value-ffffff-to-integer-value
// Return integer based color for setBackgroundColor
return intColor;
}
}