Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial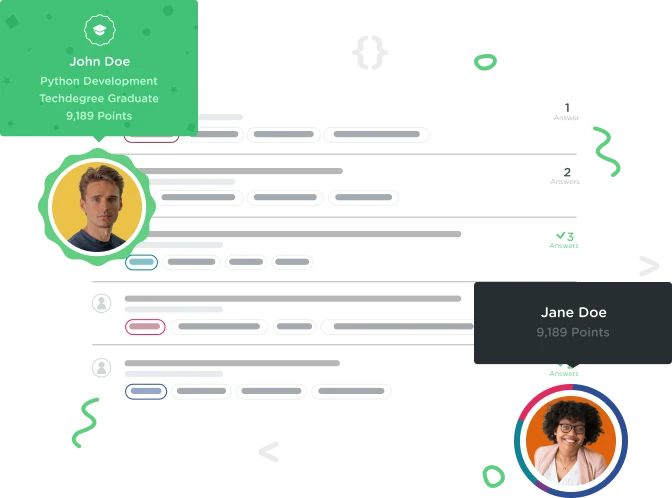

Leo Marco Corpuz
18,975 PointsPrice challenge
How would you update the _price attribute on this challenge? My guess would be calling the price property and then adding it to _price if that makes any sense.
class Product:
_price = 0.0
tax_rate = 0.12
def __init__(self, base_price):
self._price = base_price
@property
def price(self):
return self._price + (self._price * self.tax_rate)
@price.setter
def price(self):
_price+=
return _price
1 Answer
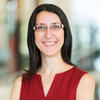
Louise St. Germain
19,424 PointsHi Leo,
Your instincts are going in the right direction! _price does need to change. :-)
But to clarify, the setter method takes a value, and assigns it to a property within a class - in this case, price. So running the setter tells the program, "Hey, I want you to set [property name] to [the value I'm telling you]."
This means is that the setter method needs not just self as a parameter, but it also needs a second parameter that takes in the value you want to assign to the property (in this case, the new price). So you might set up the first line of the setter like this:
# Instead of...
@price.setter
def price(self):
# Use this:
@price.setter
def price(self, new_value):
# The new_value will get passed to the setter from elsewhere
# That way, it knows what you want the new value to be!
Next, you need to assign the new value to the property that goes with it. In this case, that's _price, but specifically, _price for that instance of the class. So you will need to use self, to make sure Python knows which specific object you want the price change on. You can see an example of it up in the property definition:
self._price
Now, set it equal to something; in this case, the new value that got passed to the setter:
self._price = new_value
And that's it! You don't need to return anything. You see in the property definition that it returns self._price, but that's because it's a getter. In a getter, information is flowing from the object to the user - so you need to return some information to the user. In the setter function, information is going in the reverse direction: from the user to the object. So you don't need to return anything here. They are passing information to you in the extra parameter.
I hope this helps - give it another try, and I'm sure you can figure it out!
Leo Marco Corpuz
18,975 PointsLeo Marco Corpuz
18,975 PointsThanks! That helps a lot!
Louise St. Germain
19,424 PointsLouise St. Germain
19,424 PointsYou're welcome! Glad to help! :-)