Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial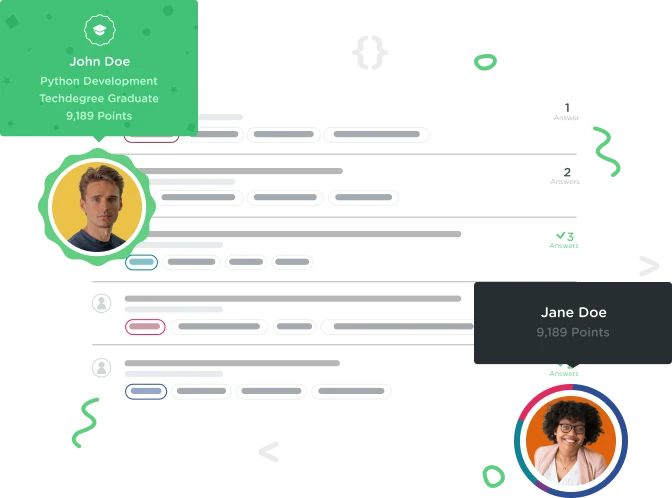
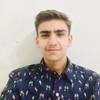
andrewgabriel
18,106 PointsPrime number program issue
I am trying to create a program to take any user input and calculate to see whether it's prime or not and return 0 or 1. 1 would be true and 0 false. I got the main core of the program written and it does work however when I wrap it with while (cin >> n) so that it keeps going as long as there is a prompt to enter a value from the user, it starts getting random and returning inaccurate results. I am writing this in C++ however it's pretty similar concept to C# or any language. Here is my code:
int main() {
int n; // initialize variable for user input
bool isPrime = true; // initialize variable for prime state
cin >> n;
while (cin >> n) {
if (n == 1) {
isPrime = false;
break;
}
for (int i = 2; i <= n/2; i++) {
if (n % i == 0) {
isPrime = false;
break;
}
}
cout << isPrime << "\n";
}
return 0;
}
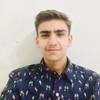
andrewgabriel
18,106 PointsSteven Parker The numbers I am using are between 1 to maybe 1000 nothing too crazy to potentially introduce any confusion in the program
1 Answer
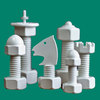
Steven Parker
230,274 PointsYou need to reset isPrime each time you begin the loop.
Since you initialilze isPrime outside the loop, once you get a False result, all other results will be False. You need to reset it to True as the loop begins again.

Jason Anello
Courses Plus Student 94,610 Points1 is not a prime number.
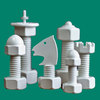
Steven Parker
230,274 PointsI guess it's excluded by definition. It's easy to get confused because it meets the criteria of having "only whole-number factors are 1 and itself".
Steven Parker
230,274 PointsSteven Parker
230,274 PointsWhat size number are you using? Are you exceeding integer precision?