Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial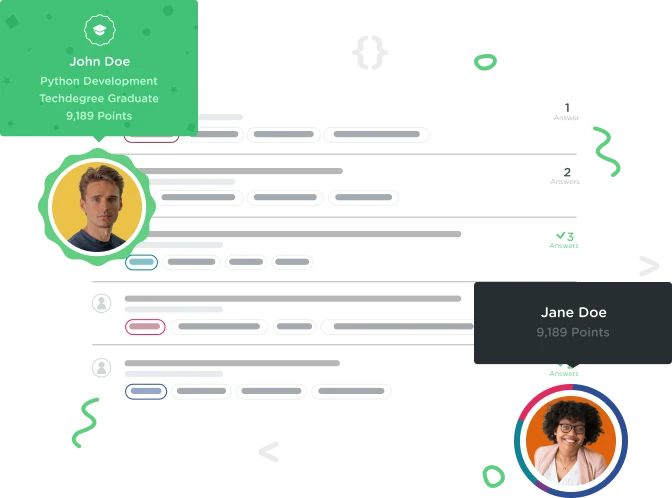

Navalkrushna Allurwar
3,557 PointsPrint out using System.out.printf the color of the new object, using the getColor method.
Print out using System.out.printf the color of the new object, using the getColor method.
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart parameter = new GoKart("Red");
System.out.printf(GoKart.getColor());
}
}
2 Answers
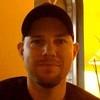
Jeremy Hill
29,567 PointsYour code should look like this:
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
GoKart kart = new GoKart("Red");
System.out.printf("The color of the new GoKart is %s", kart.getColor()); // we used the instantiated object's getColor() method here
}
}
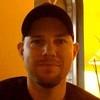
Jeremy Hill
29,567 PointsAnytime that you create a class with methods and then you instantiate that object you have access to all of its methods; so if you create a class called 'Car' you can give it all the member variables that you see fit and then add as many methods as needed. Now once it has been completed you can instantiate that object as many times as you want giving it different attributes each time. So you can have 10 car objects each having different colors, sizes, speeds, etc. That is the beauty of Object Oriented Programming (OOP); I will provide you with a little code to clear this up:
public class Car{
// the code below are all of the member variable declarations; this comes before the constructor
private String mColor;
private int mMotorSize;
private int mVelocity;
private String mMake;
public Car(String color, double motorSize, int velocity, String make){ // this is the constructor
// the code below sets the member variables to the arguments passed in.
mColor = color;
mMotorSize = motorSize;
mVelocity = velocity;
mMake = make;
}
// getter methods
// These are used because we set the member variables to private so this is the only way to access them outside of the class
public String getColor(){
return mColor;
}
public double getSize(){
return mMotorSize;
}
public int getSpeed(){
return mVelocity;
}
public String getMake(){
return mMake;
}
} // end of class Car
The code above concludes the class Car; I could have kept going and gave it some safety features and an XM satellite radio but that would have been overkill for this explanation. Now with all that being said we will instantiate the Car class object; one thing to remember is when that when you are instantiating an object you need to know how the constructor is set up. If you have just the default constructor (no parameters) then you can just do Car car = new Car(); but if you do not have a default constructor setup, meaning if you have a constructor with parameters then you have to include those arguments in your instantiation otherwise you will get an error; so here is the code to instantiate an object from class Car:
Car car1 = new Car("Blue", 5.3, 150, "Chevrolet"); // first car object
Car car2 = new Car("Green", 4.6, 100, "Ford"); // second car object
Car car3 = new Car("Red", 4.7, 130, "Dodge"); // third car object
System.out.println(car1.getMake()); // this will print out "Blue"
System.out.println(car2.getSpeed()); // this will print out 100
System.out.println(car3.getSize()); // this will print out 4.7
Each object has its own set of methods and attributes. If I was to add a setter method in there I could change the attributes but the change would only affect the object in which the change was made.
I hope this helps.