Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial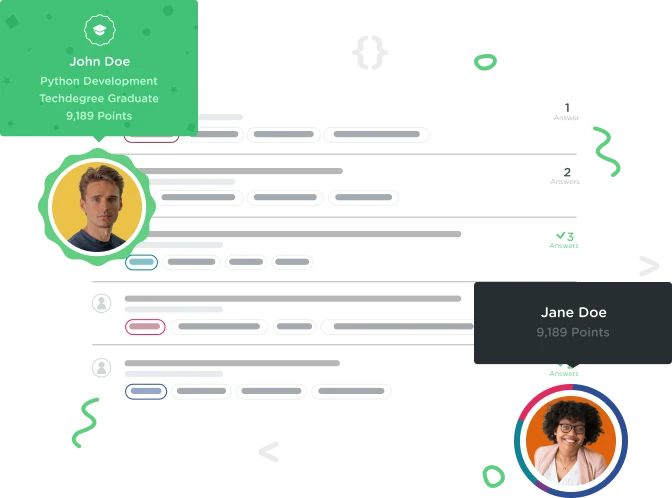

Albert Terc
2,413 PointsPrinting a whole object that's within an array.
Is there a way to print an entire object that's within an array? For example,
var tasks = [
{
name: "Do homework",
due: "01/27/2016",
complete: false,
assignedTo: "Dave"
}
];
3 Answers
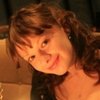
Olga Zarovskaya
1,620 PointsJSON.stringify([{a: 10, b: {c: 20}}])

Albert Terc
2,413 PointsThank you! :)
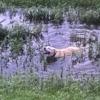
Kyle Vassella
9,033 PointsWhat if I wanted to return all the values from a single object instead?
The JSON.stringify method prints every object, every property name and every value within the array...I'm just trying to access one object at a time and list its values only.
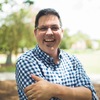
Dave McFarland
Treehouse TeacherKyle Vassella You could try this:
var tasks = [
{
name: "Do homework",
due: "01/27/2016",
complete: false,
assignedTo: "Dave"
}
];
// loop through each task in the array
for (var i = 0 ; i<tasks.length; i++) {
var task = tasks[i]
// loop through each property in the task and log value
for (var prop in task) {
console.log(task[prop]);
}
}
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherYes, so to log out that
tasks
object to the console:console.log(JSON.stringify(tasks));