Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial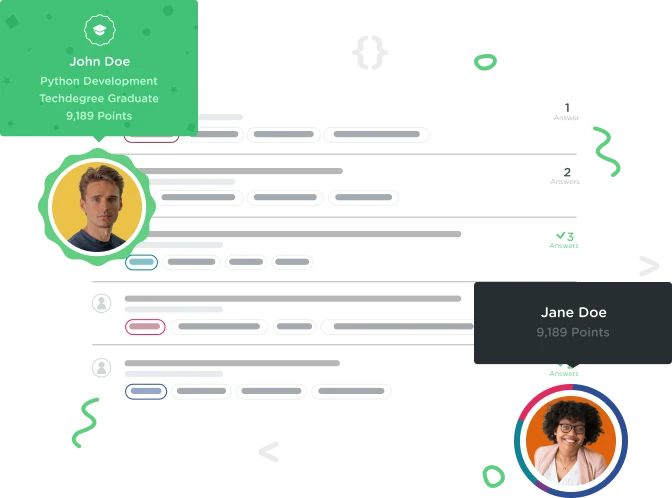
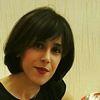
Sahar Nasiri
7,454 Pointsprint(item for item in shopping_list)
I use this code to print out my items but I get weird line which is: <generator object <genexpr> at "Numbers"> . How can I convert this to letters?
6 Answers
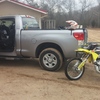
Ryan Ruscett
23,309 PointsOhhhhhhhhh oookk.
print(item for item in shopping_list) << doesn't actually do anything. Check below.
>>> a_list = ["beer", "pizza"]
>>> print(item for item in a_list)
<generator object <genexpr> at 0x00000000010375E8>
This returns a generator object. Way outside the scope of this course. It uses yield instead of return, as return statements end a function yield doesn't. It remembers the body and allows you to call the next item in a list one by one. When it comes to iterator objects and using lambda to perform an anonymous function on each item in the list so you don't have to create a whole new function. Iterator objects will give you all the results at once. It iterates through until it hits stopiteration. A for loop uses iterator object. So you can't ever pause a for loop. Generator expressions allow you to perform an action on every item in the list and call it only when you need it in order. Google iterable, iterator and generator for more information.
Generator expression should be used to perform an action on every item in a list and return that item on demand. Except you are not needing to perform any action on any item of the list. You just want them all back at once.
See how I add a string to each item in a list and call those items individually?
>>> a
['beer', 'pizza']
>>> b = (item + " is good" for item in a)
>>> b
<generator object <genexpr> at 0x00000000033398B8>
>>> next(b)
'beer is good'
>>> next(b)
'pizza is good'
>>>
You can do it in your example, but you can't just print it. Why do you have to do it that way? Plus it wont work that way. It's just what happens when people respond on the internet and don't know what they are talking about.
>>>shopping_list = ["beer", "pizza", "Doritos"]
>>>Genexp = (item for item in shopping_list)
>>> next(g)
'beer'
>>> next(g)
'pizza'
>>>
This is all you need and is so much easier!!! Just stick with this.
shopping_list = ["beer", "pizza", "Doritos"]
for item in shopping_list:
print(item)
Let me know if that helps clear things up for you or confuses you more. But you find those mixed in on the web when people who don't know what they are doing respond to questions.
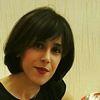
Sahar Nasiri
7,454 PointsI write the exact code as a title of my question. You can use this line of code to see the feedback. I use this line instead of " for item in shopping_list: print(item) " and as I search in google I have to get the same feedback from this line.
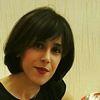
Sahar Nasiri
7,454 PointsTnx for your time :) it helps me a lot :)
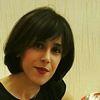
Sahar Nasiri
7,454 PointsThere are two questions. I'd be glad to get the answers :) First what does next do? and why our variable named Genexp but we call next g in the next line?
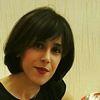
Sahar Nasiri
7,454 PointsThere are two questions. I'd be glad to get the answers :) First what does next do? and why our variable named Genexp but we call next g in the next line?
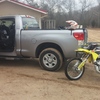
Ryan Ruscett
23,309 PointsHello,
Well, genexp is what we call a generator expressions. A for loop calls an iterator object. This iterator object is what allows python to iterate through a list and return the results. An iterator is all or nothing. It iterates the entire list and returns the results all in one shot.
When you use a function or method and you use the return keyword. Return stops the function or method and exits out of that. A generator expression which returns a generator object. Doesn't have return statements. It has what is known as Yield. Yield is like passing something to a function or method. Once that function or method receives it. It processes the request until it hits a yield. A yield will return the result, but not exit out of the function. It remembers the input you gave it. So if you gave it a list. It processes the first element in the list. Then it stops. It stops when it hits yield. Except the object is still alive. It returns the first result to you via the yield. Then when I call next. Next tells the generator object, to process the next element in the list it received and return the results. Then call next again and it processes the next one. A generator object rememers the list you gave it. And can process individual requests.
An iterator object just filters through the entire list and stops only when it's finished. Returning you the entire list.
I have found use for generators when using partials. They seem to go hand in hand, at least for the stuff I program.
It's not an easy thing to discuss. I would suggest googling it. Or opening an interpreter and playing with it like i have done above.
If you have a more direct specific question after reading what I wrote. Please do ask, it's a tuff topic to cover via a forum. Although I am happy to assist you anyway I can.
Thanks
Ryan Ruscett
23,309 PointsRyan Ruscett
23,309 PointsAre you asking how to convert a number to a string or are you asking that you get "Numbers" as a string and you want to split it up into individual letters like N, u, m, b, e, r?
If you can claify what you want and or post the code. It will be much easier to help you.