Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial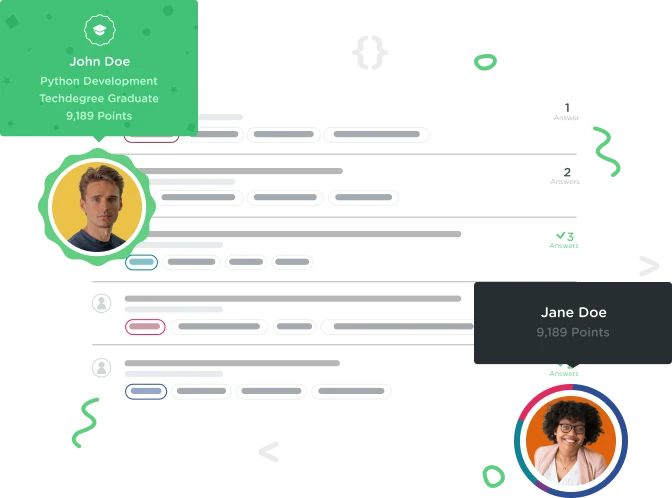

Matt Joyce
643 PointsPrints DONE instead of list, even though code is verbatim to video
So, I have gone back and double checked my script, making sure it was exactly as the video shows it, however, when I run my program, it works perfectly until I type 'DONE', at which point DONE is displayed in place of my shopping list items. Could this be a bug in the python shell? Or am I missing something?
5 Answers
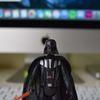
Alex Watts
8,396 PointsHello again,
In Python the placement of your code is very important. It does not show the list items in shopping_list = []
because some of your code needed to be indented into your while
loop like this...
# make a list that will hold onto our items
shopping_list = []
# print out instructions on how to use the app
print("What should we pick up to the store?")
print("Enter 'DONE' to stop adding items.")
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
break
# add new items to our list
shopping_list.append(new_item)
Hope this has helped :)

Matt Joyce
643 PointsThank you for the response! I def understand the placement in your revision, if makes sense syntactically, so I don't understand how (in the video) he breaks the loop right after {if new_item == 'DONE':} and has it still run correctly. Any idea?

Ken Alger
Treehouse TeacherMatt;
Can you post the code you are using, it will help tremendously in trying to debug the issue.
Thanks,
Ken

Matt Joyce
643 Points# make a list that will hold onto our items
shopping_list = []
# print out instructions on how to use the app
print("What should we pick up to the store?")
print("Enter 'DONE' to stop adding items.")
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
# add new items to our list
shopping_list.append(new_item)
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
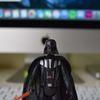
Alex Watts
8,396 PointsHello Matt,
This is my solution (see below). It would be good to see your code so we/I can identify the problem and give you a better answer. You can add your code by typing ``` before and after your code.
#Help command
def user_help():
print("Type to add item to list. To see items in list run \"show\". If you have completed your list run \"done\".")
#Show command
def show():
for items in shopping_list:
print(items)
#Add item to list
def add_item():
shopping_list.append(response)
print("Your list has {} items in it!".format(len(shopping_list)))
#Create shopping list
shopping_list = []
#Main code
def menu():
print("Welcome to shopping list v.2.0.")
menu()
while True:
response = input("> ")
response.upper()
if response == "done":
print(shopping_list)
break
elif response == "help":
user_help()
continue
elif response == "show":
show()
continue
add_item()
:)

Matt Joyce
643 Points# make a list that will hold onto our items
shopping_list = []
# print out instructions on how to use the app
print("What should we pick up to the store?")
print("Enter 'DONE' to stop adding items.")
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
# add new items to our list
shopping_list.append(new_item)
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
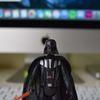
Alex Watts
8,396 PointsYeah,
Kevin's approach works in the same manner. However, his code prints the list after the while
loop has been broken out of. Whilst my method prints the list items before break. Until DONE
is entered the items will be appended because the program has not received a command to end the program yet through the if
statement.
I hope that makes sense?
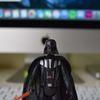
Alex Watts
8,396 PointsThis is the code from the video, which works the same way :)
# make a list that will hold onto our items
shopping_list = []
# print out instructions on how to use the app
print("What should we pick up to the store?")
print("Enter 'DONE' to stop adding items.")
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
# add new items to our list
shopping_list.append(new_item)
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
You had just not indented shopping_list.append(new_item)
which was outside of the while loop.

Matt Joyce
643 PointsYes, that make sense. It was exasperating to find that my issue was the indention, but I suppose that's coding for ya. Thanks again for the help! :)
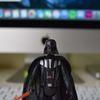
Alex Watts
8,396 PointsThat's alright! Glad to help :)
Matt Joyce
643 PointsMatt Joyce
643 Points