Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial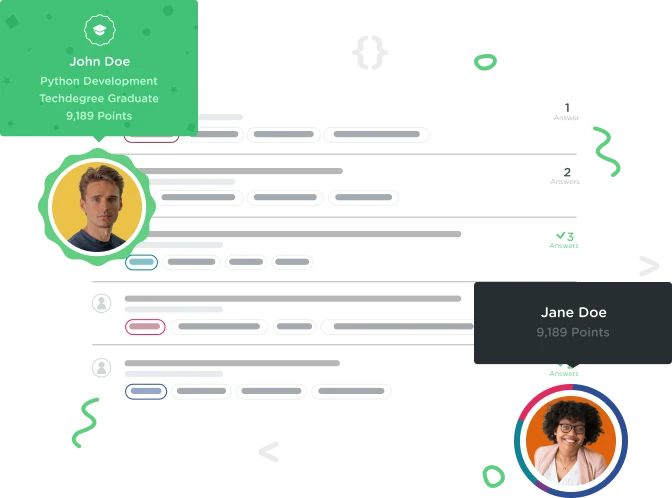

Alvaro Sanmartin Cid
Python Web Development Techdegree Student 1,065 PointsProblem deleting a character in a string
I am trying to delete a character inside a word but I am not sure why it is not being deleted. ...
def disemvowel(word):
for item in range(len(word)):
if (word[:item].upper() == "A" or
word[:item].upper() == "E" or
word[:item].upper() == "I" or
word[:item].upper() == "O" or
word[:item].upper() == "U"):
del (word[:item])
return word
4 Answers

Ray Karyshyn
13,443 PointsHi Alvaro,
There are a few issues with your code.
- Strings are immutable. So when you try to delete a vowel from the string, you get an error. To fix this first change the string to a list. (Simply use the list() method)
- word[:list] returns the splice from the zeroth index up to (but not including) the index given by the variable list. To just return the letter at the index of item, simply remove the colon -> word[list]
- The range method is inclusive and python indexing starts at zero. When you use range(len(word)), it returns the numbers from zero to the length of your word. Zero is needed, however, you get an extra index at the end. To fix this, simply subtract the list length by 1 -> range(len(word) - 1))
- Remember that the del command does weird things when performed in a loop. To solve this, I created a new list in which non-vowels will be placed -> new_list.
- This does not affect how your code runs but it is common in multi-line conditionals to have the operators at the beginning of each new line (see in the answer).
If you are still struggling, here is your code modified to work:
def disemvowel(word):
string_as_list = list(word)
new_list = []
for item in range(len(word)):
if (string_as_list[item].upper() != "A"
and string_as_list[item].upper() != "E"
and string_as_list[item].upper() != "I"
and string_as_list[item].upper() != "O"
and string_as_list[item].upper() != "U"):
new_list.append(string_as_list[item])
return ''.join(new_list)

Ray Karyshyn
13,443 PointsI apologize Alvaro, I am also new to python. Remember that the del command does weird things when performed in a loop. To solve this, I created a new list in which non-vowels will be placed -> new_list.
I have updated my code to work.

Alvaro Sanmartin Cid
Python Web Development Techdegree Student 1,065 PointsThanks! really useful A

Alvaro Sanmartin Cid
Python Web Development Techdegree Student 1,065 PointsOops I am getting
IndexError: list index out of range
When I use your code, Ray