Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial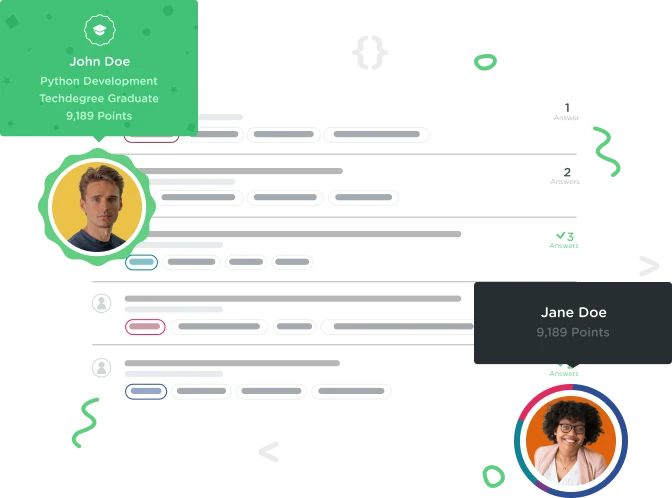

Nick Fite
11,168 PointsProblem displaying a profile.
Hey guys! I'm having a problem rendering a users profile, avatar and badges. I think it may be a problem with the code in profile.html. Here's what I have:
<div id="profile">
<img src="{{avatarUrl}}" alt="Avatar" id="avatar">
<p><span>{{username}}</span></p>
<ul>
<li><span>{{badges}}</span> Badges earned</li>
<li><span>{{javaScriptPoints}}</span> JavaScript points</li>
<li><a href="/">search again</a></li>
</ul>
</div>
And the code in renderer.js that iterates over the profile object:
function mergeValues(values, content) {
//Cycle over the keys
for(var key in values) {
//Replace all {{key}} with value from the values object
content = content.replace("{{" + key + "}}", values[key]);
}
//return merged content
return content;
}
any suggestions?
1 Answer
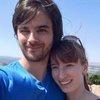
Matt Milburn
20,786 PointsHi Nick,
I believe you will need to build a regular expression from a string in order to do this. You may also want to make the regex greedy so it will replace all occurrences of key
instead of just the first occurrence. Here is a code example below. Hope it helps!
var regex = new Regex('{{' + key + '}}', 'g');
content = content.replace(regex, values[key]);