Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial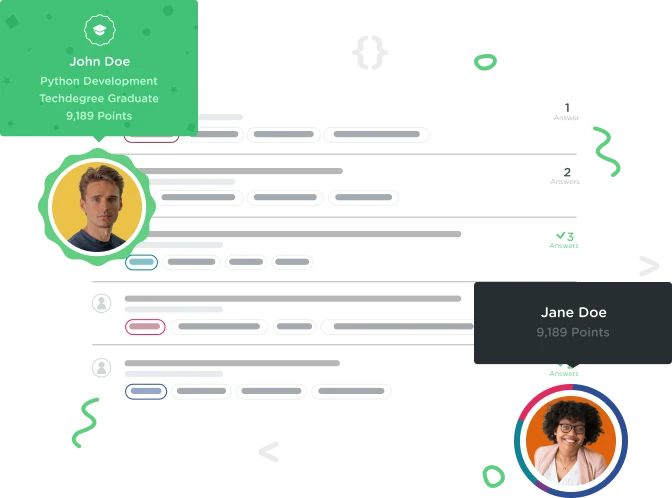

Orla McGreal
1,625 PointsProblem executing currentWeather code with debugger. Going from setSummary to timezone debugger executes JSON Exception.
Basically when I go through the code in the currentWeather setting the icon etc it all works until setSummary which returns null. The next step over the debugger jumps to JSON Exception e and returns :no value for summary. Running through the exception in MainActivity code in Call class gets run then a NamedRunnable class.
I've checked the Current Weather class and summary looks to be set up fine. Can anyone help? I'm VERY Confused!
Thanks in advance!!
Here is the code from MainActivity:
public class MainActivity extends Activity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "64fd6c2dd5fb383c62e0e523f7b27fd9";
double latitude = 37.8267;
double longitude = -122.423;
String forecastURL = "https://api.forecast.io/forecast/" + apiKey +
"/" + latitude + "," + longitude;
/// network issues
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
/// clients request for the info
Request request = new Request.Builder()
.url(forecastURL)
.build();
// putting the request into the call
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
// the response
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
} else {
alertUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught:", e);
}
catch (JSONException e) {
Log.e(TAG, "Exception caught:", e);
}
}
});
}
else {
Toast.makeText(this, getString(R.string.network_unavailable_message),
Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main UI Code is running!");
}
// throwing an exception to where you want it
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException{
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON:" + timezone);
///// setting a new json object for the currently key
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("Summary"));
currentWeather.setTimezone(timezone);
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected())
{
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
/////////////////////////////////////////
/////And now from CurrentWeather
public class CurrentWeather {
private String mIcon;
private long mTime;
private double mTemperature;
private double mHumidity;
private double mPrecipChance;
private String mSummary;
private String mTimezone;
public String getIcon() {
return mIcon;
}
public String getTimezone() {
return mTimezone;
}
public void setTimezone(String timezone) {
mTimezone = timezone;
}
public void setIcon(String icon) {
mIcon = icon;
}
public long getTime() {
return mTime;
}
public String getFormattedTime (){
SimpleDateFormat formatter = new SimpleDateFormat("h:mm a");
formatter.setTimeZone(TimeZone.getTimeZone(getTimezone()));
Date dateTime = new Date(getTime()*1000);
String timeString = formatter.format(dateTime);
return timeString;
}
public void setTime(long time) {
mTime = time;
}
public double getTemperature() {
return mTemperature;
}
public void setTemperature(double temperature) {
mTemperature = temperature;
}
public double getHumidity() {
return mHumidity;
}
public void setHumidity(double humidity) {
mHumidity = humidity;
}
public double getPrecipChance() {
return mPrecipChance;
}
public void setPrecipChance(double precipChance) {
mPrecipChance = precipChance;
}
public String getSummary()
{
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
}