Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial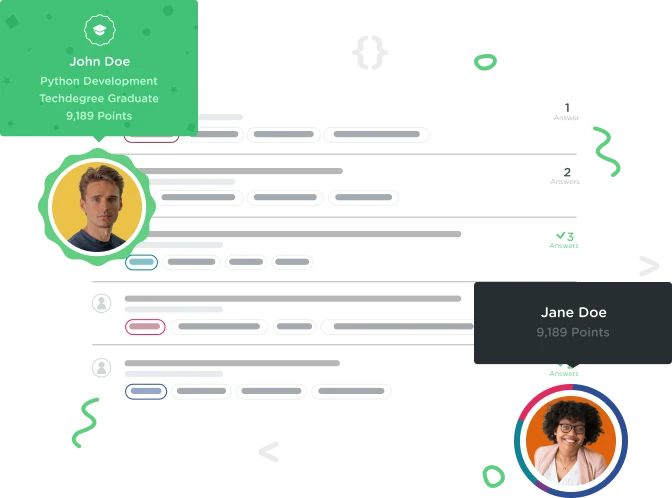

Ian Cosman
2,205 PointsProblem generating the random numbers
As shown in the video, I am trying to generate the random three integers (0,1,2) fro the code below; however, every time I hit the button the app returns the number 0...not sure what I am doing wrong.
public void onClick(View v) {
// The button was clicked so, update the answer label with an answer.
String answer = "";
// Randomly Select one of three answers: Yes, No, Maybe.
Random randomGenerator = new Random(); // Construct a new Random Generator
int randomNumber = randomGenerator.nextInt(3);
answer = Integer.toString(randomNumber);
//Update the Label with our dynamic answer.
answerLabel.setText(answer);
}
3 Answers

Ernest Grzybowski
Treehouse Project ReviewerYour code is correct. I'm guessing it's a problem with your onClick(View v)
and it's connection to your xml. Three things:
Add
Toast.makeText(this, "Hello World", Toast.LENGTH_LONG).show()
to the bottom of your onClick method. You'll be able to see if your onClick is actually being fired.Paste your xml from your layout here/make sure the
onClick
attribute in your xml is set toonClick
It's technically possible for you to return 0 a bunch of times in a row. Unlikely, but technically a chance =)
EDIT:
Most likely the problem is the this
keyword in my Toast
example. It's referring to your onClickListener instead of your activity. Simply change this
to your classname .this
(Example: MainActivity.this
) or just use getApplicationContext()
.

Ian Cosman
2,205 PointsHi Ernest,
Thanks for the reply. When I add the code you asked me to, to the bottom of the onClick Method, makeText is underlined in red with the following message. "The method makeText(Context, CharSequence, int) in the type Toast is not applicable for the arguments (new View.OnClickListener(){}, String, int)". Any thoughts? Also, I am brand new at programming apps and even using Java....not quite sure how to make sure the onClick attribute in my xml is set to onClick.
Thanks for the help!
Ian

Ernest Grzybowski
Treehouse Project ReviewerSorry, I thought you were using the onClick
attribute in xml set to a method called onClick
. My mistake. Could you please just post your entire code? It would help so everyone can get to the bottom of your issue a little bit faster. You can do that in a pastebin if it helps!
Go to http://pastebin.com/ and Submit
the code, copy the link, and paste it back here!
I updated my original answer to work with the code you most likely have written.

Ian Cosman
2,205 PointsHere it is!
package com.example.crystal.ball;
import java.util.Random;
import android.app.Activity; import android.os.Bundle; import android.view.Menu; import android.view.View; import android.widget.Button; import android.widget.TextView; import android.widget.Toast;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declare our View variables and assign them the views from the layout file
final TextView answerLabel = (TextView) findViewById(R.id.textView1);
Button getAnswerButton = (Button) findViewById(R.id.button1);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// The button was clicked so, update the answer label with an answer.
String answer = "";
// Randomly Select one of three answers: Yes, No, Maybe.
Random randomGenerator = new Random(); // Construct a new Random Generator
int randomNumber = randomGenerator.nextInt(3);
answer = Integer.toString(randomNumber);
//Update the Label with our dynamic answer.
answerLabel.setText(answer);
Toast.makeText(getApplicationContext(), "Hello World", Toast.LENGTH_LONG).show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}

Ernest Grzybowski
Treehouse Project ReviewerThanks! Please check my original answer for an edit on the Toast
method I'd like you to try out.
Edit:
Or if it's easier just copy and paste this code and tell me what happens.
package com.example.crystal.balll;
import java.util.Random;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declare our View variables and assign them the views from the layout file
final TextView answerLabel = (TextView) findViewById(R.id.textView1);
Button getAnswerButton = (Button) findViewById(R.id.button1);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// The button was clicked so, update the answer label with an answer.
String answer = "";
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(3);
answer = Integer.toString(randomNumber);
//Update the Label with our dynamic answer.
answerLabel.setText("Ernest was here");
Toast.makeText(getApplicationContext(), "Hello World", Toast.LENGTH_LONG).show();
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}

Ernest Grzybowski
Treehouse Project ReviewerAnother update:
I just implemented your code very quickly just to see if there was something obvious I was missing, but... nope! Your code worked fine, and I have a random number generator working fine on my emulator. Let me know if you are still having trouble. The last thing I can think of is that your project isn't being loaded onto your emulator or device. That's why if you use my code and don't see my name... then we have a problem!

Ian Cosman
2,205 PointsSomething must be wrong as you mentioned....I assume I should see "Ernest was here" :-), however, the app still returns 0.
Also, I am seeing a message in the console tab which says "ActivityManager: Warning: Activity not started, its current task has been brought to the front"...not sure what that means

Ernest Grzybowski
Treehouse Project ReviewerMy guess is that the app is simply not being loaded onto your device. Are you using an emulator or a physical device?

Ian Cosman
2,205 PointsI am using an emulator (eclipse) I downloaded from the files associated with the Android courses on the treehouse website

Ernest Grzybowski
Treehouse Project ReviewerMake sure you click/select your project in the left of eclipse (in the file tree/directory) and then press the "Green" play button.
EDIT:
Another option is to just right click the project folder on the left side of Eclipse, and go down to Run As...
and select Android Project
.

Ian Cosman
2,205 Pointsok, I am getting a new message now...
[2013-07-03 00:06:34 - Crystal Balll] The connection to adb is down, and a severe error has occured. [2013-07-03 00:06:34 - Crystal Balll] You must restart adb and Eclipse. [2013-07-03 00:06:34 - Crystal Balll] Please ensure that adb is correctly located at 'E:\Android development\android_dev_environment_windows_64bit\android_dev_environment\android-sdk-windows\platform-tools\adb.exe' and can be executed.

Ernest Grzybowski
Treehouse Project ReviewerOkay, well adb is the Android Device Bridge, and if it "goes down" then you basically lose your connection from Eclipse to your device/emulator. This doesn't happen to often anymore (especially when simply working with an emulator) but you should simply:
- Open Task Manager (Windows: Ctrl + Shift + Esc)
- Go to Processes
- Find adb.exe and End Process
- Then restart eclipse

Ian Cosman
2,205 Pointsstill not working.....back to the previous message

Ernest Grzybowski
Treehouse Project ReviewerThis is a common "problem". Here are some solutions: http://stackoverflow.com/questions/4072706/the-connection-to-adb-is-down-and-a-severe-error-has-occured
If you really don't want to mess around with the command line or adb process, you can simply restart your computer.

Ian Cosman
2,205 Pointsok, I will give that a try for now....I will get back to you if I need further help with this...thanks for helping me out.

Ernest Grzybowski
Treehouse Project ReviewerNot a problem. You had "adb" running before, because you were able to get the app onto the emulator, but something went wrong somewhere. Heh. It happens. A restart may be the simplest solution. Good luck!

Ian Cosman
2,205 PointsHi Ernest, I tried restarting and still, the program returns 0....hehe, not sure what I am doing wrong here. However, I noticed a couple of messages in the logCat..... 1 - Not Late enabling check JNI (already on) 2 - Error opening trace file: No such file or directory! 3 - Emulator without GPU emulation detected

Ernest Grzybowski
Treehouse Project ReviewerNext step... create a new Android Application project and put in a simple toast message (Like the one above) and see if you can run that one successfully.

Allen Mackey
841 PointsI'm having the same issue. My logcat - 11-03 11:53:41.246: E/Trace(604): error opening trace file: No such file or directory (2)