Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial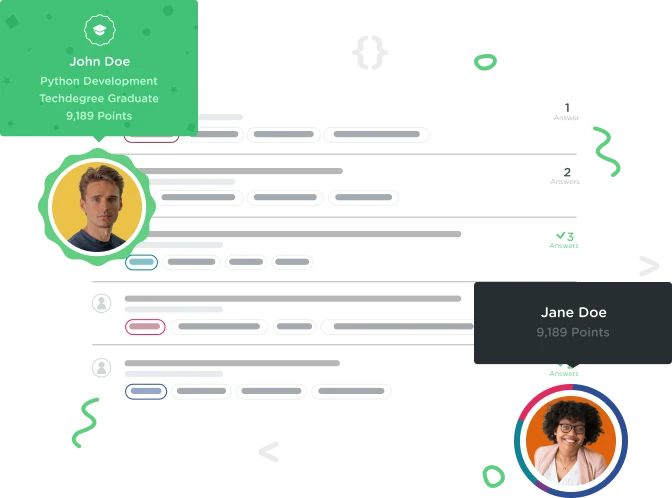
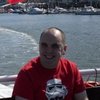
Sean Flanagan
33,235 PointsProblem getting unit
Hi. I can't get 'sq ft' to show on the console. Does anyone know why please?
Here's my JS:
function getRandomNumber(upper) {
var randomNumber = Math.floor( Math.random() * upper ) + 1;
return randomNumber;
}
/*
console.log(getRandomNumber(6));
console.log(getRandomNumber(100));
console.log(getRandomNumber(10000));
console.log(getRandomNumber(2));
*/
function getArea(width, length) {
var area = width * length;
return area + " " + unit;
}
console.log(getArea(10, 20, "sq ft"));
Thanks.
Sean
3 Answers
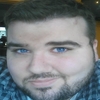
Marcus Parsons
15,719 PointsHi Sean,
The reason why you can't get "sq ft" to show is that you are only using 2 arguments for the function when you need to use 3. If you add in the 3rd argument for unit, you'll be able to see "sq ft" in your console.log
command.
Edit: P.S. You can see better syntax highlighting by adding the language you are pasting to the forums beside the first 3 backticks. In this case you'd put 3 backticks and then javascript. Like this: '''javascript
function getRandomNumber(upper) {
var randomNumber = Math.floor( Math.random() * upper ) + 1;
return randomNumber;
}
/*
console.log(getRandomNumber(6));
console.log(getRandomNumber(100));
console.log(getRandomNumber(10000));
console.log(getRandomNumber(2));
*/
//add unit to argument list for function so that it can be used
function getArea(width, length, unit) {
var area = width * length;
return area + " " + unit;
}
console.log(getArea(10, 20, "sq ft"));
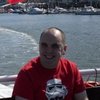
Sean Flanagan
33,235 PointsJust copied and pasted your code into my workspace. I opened my console and it says "200 sq ft". So here arguments[2] represents sq ft?
Have I done it right?
Thanks Marcus.
Sean
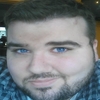
Marcus Parsons
15,719 PointsAbsolutely! Just keep in mind that arguments
is an array representing anything that is passed into the function. It starts with 0 at the first argument, 1 for the second, and so on.
You could even redo your entire function to use arguments
(this can definitely come in handy when using functions that need to take in any number of arguments the user wishes):
//just to show how you can use arguments
//to complete a task
//although the code should always be intuitive and easy to read
function getArea() {
var area = arguments[0] * arguments[1];
return area + " " + arguments[2];
}
console.log(getArea(10, 20, "sq ft"));
I would keep your function as it is, though, because using arguments
only is less intuitive for this function because you can't really read what's going on here.
I used this type of method of writing functions to write a mean
function that can accept any number of arguments and return the mean/average of those numbers. It comes in handy when you can't name every single argument that can go into a function.
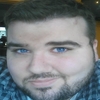
Marcus Parsons
15,719 PointsPart of the power of using arguments
is that it is an array and can be looped through like any other array. Keep that in mind when writing future functions, and you can write some awesome stuff. :)
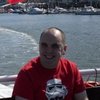
Sean Flanagan
33,235 PointsOkay thanks. :-)
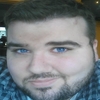
Marcus Parsons
15,719 PointsTo increase your experience in JS, try writing a function that can get the average of any amount of numbers passed into it. :) No problem, man! Happy Coding!
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsThanks Marcus. I added unit into the function getArea parentheses and it worked. I've upvoted your reply and given you the Best Answer.
Thank you
Sean :-)
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsJust remember that when you need to pass in extra values to put an argument for those values in the function, as well.
There are other ways to do it, though, too. A neat object to use for functions is the
arguments
object. Thearguments
objects acts like an array that can access any number of arguments passed into a function without them being named. So, you could leaveunit
out of the argument list and still access theunit
value by usingarguments
. Copy and paste this into your console and see it in action: