Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial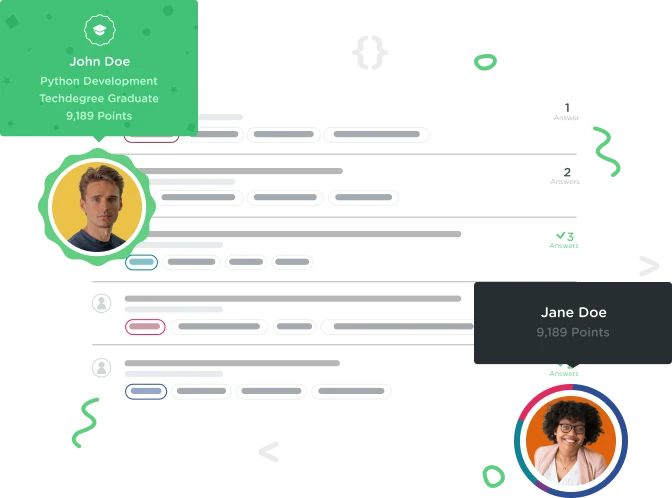
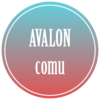
AVALONcomu Profile
36,146 PointsProblem: if you log out and sign up as new user - tableview of friends remain still, with friends of previous user!
Problem:
- user1 is logged in and has some friends.
- user1 logged out.
- sign up as a new user2.
- tableview of friendsviewcontroller does not change, so you can see all friends of user1 untill you edit your friends!
#import "FriendsViewController.h"
#import "EditFriendsViewController.h"
@interface FriendsViewController ()
@end
@implementation FriendsViewController
- (void)viewDidLoad {
[super viewDidLoad];
self.friendsRelation = [[PFUser currentUser] objectForKey:@"friendsRelation"];
}
-(void)viewWillAppear:(BOOL)animated{
PFQuery* query = [self.friendsRelation query];
[query orderByAscending:@"username"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if (error) {
NSLog(@"error: %@ %@",error,[error userInfo]);
}else{
self.friends = objects;
[self.tableView reloadData];
}
}];
}
-(void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender{
if ([segue.identifier isEqualToString:@"showEditFriends"]) {
EditFriendsViewController* viewController = (EditFriendsViewController*)segue.destinationViewController;
viewController.friends = [NSMutableArray arrayWithArray:self.friends];
}
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
// Return the number of rows in the section.
return self.friends.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"Cell"
forIndexPath:indexPath];
PFUser* user = [self.friends objectAtIndex:indexPath.row];
cell.textLabel.text = user.username;
return cell;
}
@end
2 Answers
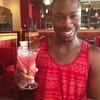
Matthew Reed
Courses Plus Student 17,986 PointsI think whenever the friends list changes you have to call reloadData
on the table view
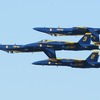
colinli
5,073 PointsInstead of using the viewDidLoad
method, use the viewWillAppear
method or the viewDidAppear
method. This was explained in a previous video.