Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial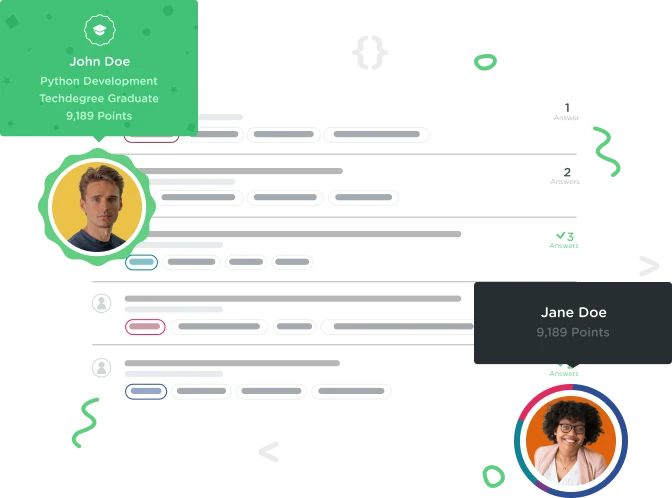

Todd Alcock
5,516 PointsProblem when creating createLi function
When I created a function I got an error saying the li undefined did i miss a li declaration? I noticed the teacher copied the li declaration away from the submit click event.
const form = document.getElementById('registrar');
const input = document.querySelector('input');
let ul = document.getElementById('invitedList');
function createLi(text) {
let li = document.createElement('li');
li.textContent = text;
let confirm = document.createElement('input');
let label = document.createElement('label');
let remove = document.createElement('button');
remove.type = 'button';
remove.textContent ='Remove';
label.textContent = 'Confirmed';
confirm.type = 'checkbox';
li.appendChild(label);
label.appendChild(confirm);
li.appendChild(remove);
return li;
};
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = '';
createLi(text);
ul.appendChild(li)
});
ul.addEventListener('change', (e) => {
const checkbox = event.target;
const checked = checkbox.checked;
const listItem = checkbox.parentNode.parentNode;
if (checked) {
listItem.className = "responded";
}
else {
listItem.className = "";
}
});
ul.addEventListener('click', (e) => {
if (e.target.tagName === 'BUTTON') {
const li = e.target.parentNode;
const ul = li.parentNode;
console.log(ul)
ul.removeChild(li);
}
});
2 Answers
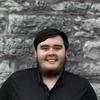
Michael Hulet
47,913 PointsYou've defined/used several li
symbols in your code, so I'm guessing at which one is giving you the error, but I'd be willing to bet it's in the submit event listener on form
. In that listener, you properly call your createLi
function, but you discard the new element it return
s immediately. However, on the next line, you try to reference a symbol called li
which you haven't yet defined in that scope. I think all you should have to do to fix it is to assign the result of your createLi
call to a constant called li
, and you should be good to go
const li = createLi(text);
ul.appendChild(li);

Erik Young
15,016 PointsI had a similar question to the one posted here, but I'm not clear what is going on. I know we created a function to clean up our code a bit. This makes sense. After that it we would need to call that function in the event handler, just as Todd did in the example above. Where I'm getting confused though is why do we need to create a const li? Is it because that is what was returned in the function?