Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial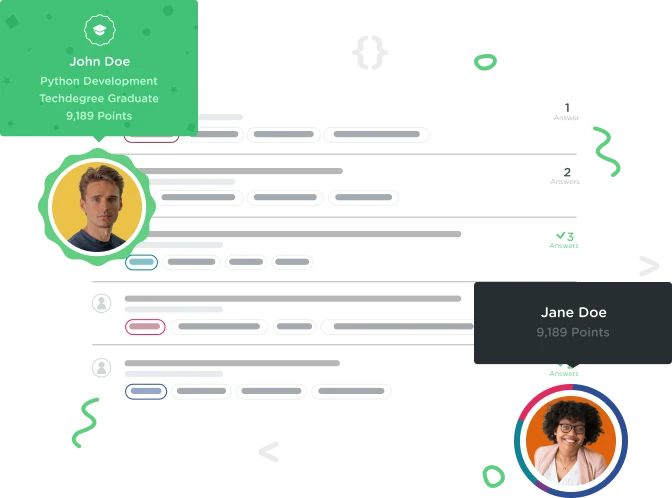
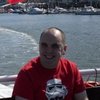
Sean Flanagan
33,235 PointsProblem with closing parenthesis
Hi there.
My Java program won't work - again.
Apparently a line in my Example.java containing just a closing parenthesis is to blame.
I'll post the code below:
import java.util.Date;
import com.teamtreehouse.Treet;
public class Example {
public static void main(String []args) {
Treet treet = new Treet(
"craigsdennis",
"Feeling overwhelmed by learning to #code?" +
"Try out this thought hack I whipped up: http://buff.ly/1LjAZrJ #development #webdev",
new Date(1437084178)
)
System.out.printf("This is a new Treet: %s %n", treet);
}
}
The repl is off. The error message is:
Example.java:12: error: ';' expected
)
^
Once again, I'd appreciate any help.
Sean :-)
3 Answers
Christopher Augg
21,223 PointsHello Sean,
Looks like the ; is missing:
Treet treet = new Treet(
"craigsdennis",
"Feeling overwhelmed by learning to #code?" +
"Try out this thought hack I whipped up: http://buff.ly/1LjAZrJ #development #webdev",
new Date(1437084178)
); <-----------
System.out.printf("This is a new Treet: %s %n", treet);
Let me know if this helps man
Regards,
Chris
Christopher Augg
21,223 PointsHello Sean,
Yep, it is your Treet class that is the issue. You have an empty tree class. You will need to complete it before it can be instantiated within your Example class. Do you know where this class is under the com ? Here are some hints. I hope they help.
Regards,
Chris
package com.teamtreehouse;
public class Treet {
//Do you need any variable to hold values passed into the constructor?
//How many arguments do you need to pass to the constructor? What will be their types?
//Do you need to override the toString method?
}
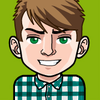
Harry James
14,780 PointsChristopher Augg is right here - you need to fill in the constructor for your Treet class.
Also +1 to Christopher for the hints - they're awesome :)
You learn so much better by just being given hints than by someone just posting the correct code without an explanation :D
Edit: Also, I don't want to take credit for Christopher's work - just wanted to give kudos :)
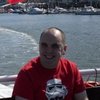
Sean Flanagan
33,235 PointsHere's what I've done since:
package com.teamtreehouse;
import java.util.Date;
public class Treet implements Comparable {
private String mAuthor;
private String mDescription;
private Date mCreationDate;
public Treet(String author, String description, Date creationDate) {
mAuthor = author;
mDescription = description;
mCreationDate = creationDate;
}
@Override
public String toString() {
return "Treet: \"" + mDescription + "\" - @" + mAuthor;
}
public String getAuthor() {
return mAuthor;
}
public String getDescription() {
return mDescription;
}
public Date getCreationDate() {
return mCreationDate;
}
public String[] getWords {
return mDescription.toLowerCase().split("[^\\w#@']+");
}
}
Thank you both. :-)
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsHi Chris. Thanks for your efforts. I've changed Example.java to:
I got this error message:
I've forked my work space and taken a snapshot.
I would appreciate any further help - sorry to be a nuisance.
Sean
Christopher Augg
21,223 PointsChristopher Augg
21,223 PointsSean,
No problem... Your Example.java is totally fine now. It is complaining about your Treet constructor within your Treet class. Did you pass the arguments into the Treet constructor with something similar to: