Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial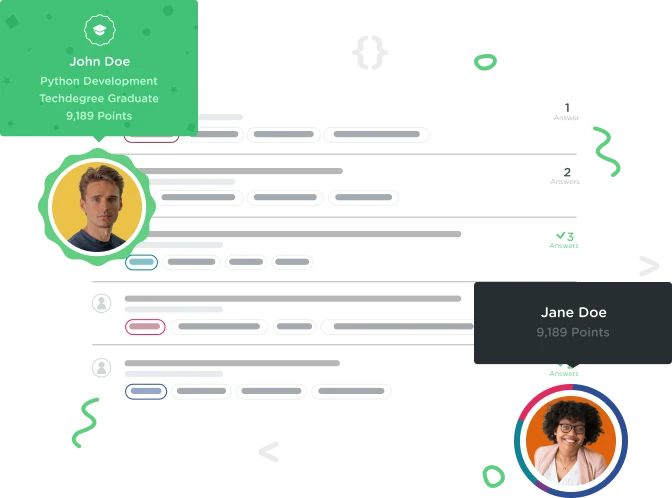

Warren Harding
1,942 PointsProblem with code when trying to amend the Treehouse FunFacts app.
I've been playing around with the FunFacts app, trying to make some changes and get it to do different things. I'm trying to get the text and background colours of the label to change depending on whether the index value of the fun fact pulled from the array is odd or even. I've tried to do that by defining a new method which assigns a colour based on whether the random number generate is odd or even. My problem is that the compiler doesn't recognise 'randomNumber'. I thought that because it's all within the same struct, that it would be within scope. I suspect it's not recognised because it was not 'returned' from the previous method within the struct. Is this correct? Any ideas on how I can make it work? Code copied below.
Thanks for your help. Warren
struct FactProvider {
var facts = [
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the Earth when the Great Pyramid was being built."
]
func randomFact() -> String {
let randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: facts.count)
return facts[randomNumber]
}
func changeColors() {
let txtColor: UILabel
let bckColor: UILabel
if randomNumber % 2 == 0 {
txtColor.textColor = UIColor.black
bckColor.backgroundColor = UIColor.white
} else {
txtColor.textColor = UIColor.white
bckColor.backgroundColor = UIColor.black
}
}
}
2 Answers
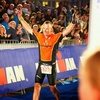
Steve Hunter
57,712 PointsHi Warren,
You're correct that randomNumber
is out of scope. It exists only within the method where it is created. It isn't returned out of the method, it is just used to set the index of the array.
You can move it so that it is in scope across the whole struct. Move it above the array (or after, but not in a method) but use it in the same way inside the method without creating it again, i.e. don't use let
or var
again else you'll create another variable of the same name with restricted scope. As you're going to change its value, declare it as a var
.
Something like:
struct FactProvider {
var facts = [
"Ants stretch when they wake up in the morning.",
// snip!
]
var randomNumber: Int
func randomFact() -> String {
randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: facts.count)
// etc
I hope that 1. makes sense and 2. works OK!
Let me know how you get on.
Steve.

Warren Harding
1,942 PointsThanks a lot Steve... That does make sense. I'd gone on in the lessons and actually found what I was trying to do - albeit in a very different (and much simpler) way. I will be playing around again today though and will try this. Thanks again for your help. Much appreciated. Warren
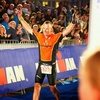
Steve Hunter
57,712 PointsNo problem - happy to try to help!