Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial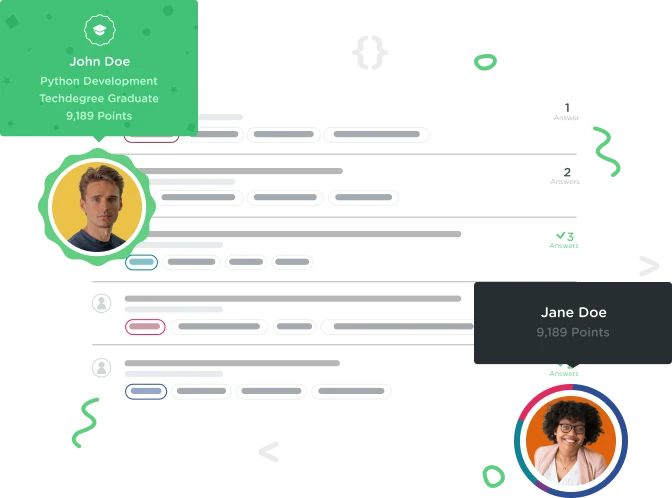
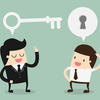
Eleni Minadaki
3,687 PointsProblem with if statement
Hi, I would like to ask about my if statement, I am new in java and can't figure it out. My app has 2 buttons, previous and next. Needs to hide the previous Button when app first loads, when the user see the first article there is no need to see the previous button. Thanks.
public class StoryActivity extends AppCompatActivity {
public static final String TAG = StoryActivity.class.getSimpleName();
private Story mStory = new Story();
private ImageView mImageView;
private TextView mTextView;
private Page mCurrentPage;
private String mName;
private Button mPreviousButton;
private Button mNextButton;
private ScrollView mScrollView;
private Button mStartButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story2);
//Intent intent = getIntent();
//mName = intent.getStringExtra(getString(R.string.key_name));
if(mName == null){
mName = "";
}
Log.d(TAG, mName);
mImageView = (ImageView)findViewById(R.id.storyImageView);
mTextView = (TextView)findViewById(R.id.storyTextView);
mPreviousButton = (Button)findViewById(R.id.previousButton);
mNextButton = (Button)findViewById(R.id.nextButton);
mTextView.setMovementMethod(new ScrollingMovementMethod());
loadPage(0);
}
private void loadPage(int choice) {
mCurrentPage = mStory.getPage(choice);
Drawable drawable = getResources().getDrawable(mCurrentPage.getImageId());
mImageView.setImageDrawable(drawable);
String pageText = mCurrentPage.getText();
//add the name if placeholder included.
//pageText = String.format(pageText,mName);
mTextView.setText(pageText);
if(mCurrentPage.isSingle()){
mPreviousButton.setVisibility(View.VISIBLE);
mNextButton.setText("Start From The Beginning");
mNextButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mNextButton.setVisibility(View.VISIBLE);
loadPage(0);
}
});
}else{
mPreviousButton.setText(mCurrentPage.getChoices().getText1());
mNextButton.setText(mCurrentPage.getChoices().getText2());
mPreviousButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int previousPage = mCurrentPage.getChoices().getPreviousPage();
loadPage(previousPage);
}
});
mNextButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int nextPage = mCurrentPage.getChoices().getNextPage();
loadPage(nextPage);
//test if for 0page
//if (page == 0) {
/** int page = 0;
mPreviousButton.setVisibility(View.INVISIBLE);
//till here
} else
mPreviousButton.setVisibility(View.VISIBLE);
*/
}
});
}}
}
3 Answers
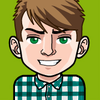
Harry James
14,780 PointsHey there Eleni!
When mCurrentPage.isSingle(), you want to hide the previous button, and just show one button to proceed:
mPreviousButton.setVisibility(View.INVISIBLE);
You should replace the line where you set the View.VISIBLE
to View.INVISIBLE
here.
Then, you want to check if the page is the first page, which means we should proceed to the next page when tapping on the Next Button, or if it is a last page (Last page is a single button page which is not first page), which means we should finish the activity when tapping on the Next Button. We can do that as follows:
if (mCurrentPage.getPageId() == 0) {
// This is the first page...
mNextButton.setText("PROCEED");
mNextButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// IMPORTANT: The button should be set to visible again, ready for the future story pages.
mPreviousButton.setVisibility(View.VISIBLE);
loadPage(1);
}
});
} else {
// This is one of the last pages...
mNextButton.setText("PLAY AGAIN");
mNextButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// There's no need to re-show the button here. The activity will be destroyed and buttons will be re-created.
finish();
}
});
}
And, as you have already done, if the page is not single, you should just load the corresponding page based on the user's choice.
Hope it helps and if you have any more questions/problems, feel free to give me a shout :)
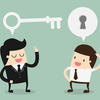
Eleni Minadaki
3,687 PointsHi Harry! Hope you are fine! Your answer is very detailed but I am confused enough with the code and for me is not so clear. Look, when the app first loads the first page the user see is page 0 and my last page is page 50. There is 2 problems with the previous button: 1)only when the 0page loads need to hide the previousButton 2)previousButton doesn't work when is on last page(50)
Could you tell me what should been written in my previous code? Do you need to post all the classes from the app? Sorry for the trouble...
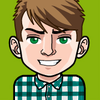
Harry James
14,780 PointsHey again Eleni,
The code above will hide the previous button on page 0 and the last page(s) (To define a last page in your code, you should use the appropriate constructor to create a new Page() that DOES NOT take in any Choices). On all other pages, the button will be shown.
The first line of code I mentioned in the above post should go in the loadPage() method at the top of the if(mCurrentPage.isSingle()) method, REPLACING the line that sets it to VISIBLE (We don't want it to be visible, as this line of code only runs if the current page is a single page, and hence should have a single button).
The second chunk of code I attached will do the checking of the single page, to see whether it is a first or last page. It should fit in underneath the line that sets the visibility to INVISIBLE. You should also replace any code up to, but NOT including, the else { } statement under if(mCurrentPage.isSingle()).
So, here's what your loadPage() method should look like with all of the changes in place:
private void loadPage(int choice) {
mCurrentPage = mStory.getPage(choice);
Drawable drawable = getResources().getDrawable(mCurrentPage.getImageId());
mImageView.setImageDrawable(drawable);
String pageText = mCurrentPage.getText();
//add the name if placeholder included.
//pageText = String.format(pageText,mName);
mTextView.setText(pageText);
if(mCurrentPage.isSingle()){
// Commented code = Deleted code:
// mPreviousButton.setVisibility(View.VISIBLE);
// mNextButton.setText("Start From The Beginning");
// mNextButton.setOnClickListener(new View.OnClickListener() {
// @Override
// public void onClick(View v) {
// mNextButton.setVisibility(View.VISIBLE);
// loadPage(0);
//
// }
// });
// ** NEW CODE STARTS HERE **
mPreviousButton.setVisibility(View.INVISIBLE);
if (mCurrentPage.getPageId() == 0) {
// This is the first page...
mNextButton.setText("PROCEED");
mNextButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// IMPORTANT: The button should be set to visible again, ready for the future story pages.
mPreviousButton.setVisibility(View.VISIBLE);
loadPage(1);
}
});
} else {
// This is one of the last pages...
mNextButton.setText("PLAY AGAIN");
mNextButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// There's no need to re-show the button here. The activity will be destroyed and buttons will be re-created.
finish();
}
});
}
// ** NEW CODE ENDS HERE **
}else{
mPreviousButton.setText(mCurrentPage.getChoices().getText1());
mNextButton.setText(mCurrentPage.getChoices().getText2());
mPreviousButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int previousPage = mCurrentPage.getChoices().getPreviousPage();
loadPage(previousPage);
}
});
mNextButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int nextPage = mCurrentPage.getChoices().getNextPage();
loadPage(nextPage);
//test if for 0page
//if (page == 0) {
/** int page = 0;
mPreviousButton.setVisibility(View.INVISIBLE);
//till here
} else
mPreviousButton.setVisibility(View.VISIBLE);
*/
}
});
}}
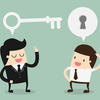
Eleni Minadaki
3,687 PointsHey Harry!! Thanks a lot for your reply again. Your answer is very helpful for me, I understand some things I haven't. Thank you.