Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial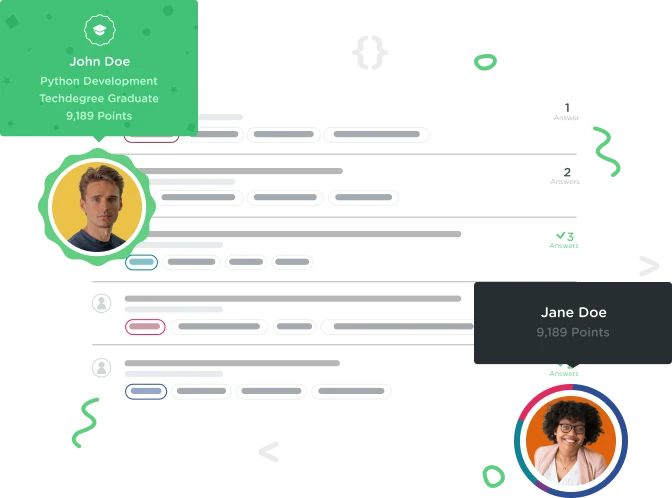

Endrin Tushe
4,591 PointsProblem with random.randint() in extra credit Numbers game challenge
Hello, I am trying to build the opposite version of the numbers game where the player picks the number and the computer tries to guess it five times. I think I am really close to getting the script right, however, my problem is that the computer picks the same random number. How do I fix that: Below is my script:
import random comp_guess = random.randint(1,10) guess_list=[]
def my_game(): my_secret_number = int(input(''' Enter a secret number between 1 - 10 the computer will try five times to guess your number >>>>: ''')) while len(guess_list) < 5: if comp_guess == my_secret_number: print("Your number is {}.I knew I could get it!".format(my_secret_number)) break else: print("I am guessing {}".format(comp_guess))
guess_list.append(comp_guess)
else:
print ("Shucks! I didn't get it!")
reveal_prompt = input("Will you tell me what your number is? Press Y/n.")
if reveal_prompt == 'Y':
print ("The secret number is: {}".format(my_secret_number))
else:
second_chance = input("Okay---I see how it is! Will you let me play again? Press Y/n: ")
if second_chance == 'Y':
my_game()
else:
print("Well fine be that way...I hope you and your secret number are happy together!")
my_game()
4 Answers
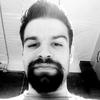
Jeffery Austin
8,128 PointsThis seems to work, really cool idea, had fun playing with the code:
import random
def my_game():
guess_list=[]
my_secret_number = int(input(''' Enter a secret number between 1 - 10 the computer will try five times to guess your number >>>>: '''))
while len(guess_list) < 5:
comp_guess = random.randint(1,10)
if comp_guess in guess_list:
continue
if comp_guess == my_secret_number:
print("Your number is {}.I knew I could get it!".format(my_secret_number))
break
else:
print("I am guessing {}".format(comp_guess))
guess_list.append(comp_guess)
print ("Shucks! I didn't get it!")
reveal_prompt = input("Will you tell me what your number is? Press Y/n.")
if reveal_prompt == "Y":
print ("The secret number is: {}".format(my_secret_number))
else:
continue
second_chance = input("Okay---I see how it is! Will you let me play again? Press Y/n: ")
if second_chance == "Y":
my_game()
else:
print("Well fine be that way...I hope you and your secret number are happy together!")
my_game()

Hanfei Zhu
2,884 PointsHere's my attempt at the solution. The logic here allows the program to narrow the range down as you tells it whether the number is too big or too small.
SPOILER ALERT: solution below
import random
## initiates list
guesses_too_large = []
guesses_too_small = []
def initiate_game(a = 1, b = 50):
'''
function initiates the game with lower bound, a, and upper bound, b.
by default a = 1 and b = 50.
'''
## prompt the human player to choose a number
print("Please think of a number from {} to {}.".format(a, b))
input("When ready, press enter to begin.")
## sets the upper and lower limit for computer_guess() later on.
guesses_too_large.append(b)
guesses_too_small.append(a)
## computer starts to guess
computer_guess(a, b)
def computer_guess(a, b):
'''
function gets the computer to start guessing with a random number between a and b, inclusive.
'''
## guesses and requires human input to check whether the guess is correct.
guess = random.randint(a, b)
print("Is your number {}?".format(guess))
option = check_answer()
## when guess is correct, provide user with option to restart game.
## when user forgets the number, restart game.
if option == "1" or option == "4":
restart_game()
## when guess is too small
elif option == "2":
## collects the value into a list so that later on the range can be narrowed.
guesses_too_small.append((guess + 1))
## this try and except sequence is to prevent an error where the user
## forgets the number and the guessing converges.
try:
## uses recurrence to guess again
computer_guess((guess + 1), guesses_too_large[-1])
except ValueError:
print("You must have made a mistake!")
restart_game()
## when guess is too large
elif option == "3":
## collects the value into a list so that later on the range can be narrowed.
guesses_too_large.append((guess - 1))
## this try and except sequence is to prevent an error where the user
## forgets the number and the guessing converges.
try:
## uses recurrence to guess again
computer_guess(guesses_too_small[-1], (guess - 1))
except ValueError:
print("You must have made a mistake!")
restart_game()
def check_answer():
'''
function takes input from the human player to check whether the guess is correct.
'''
option = input("""1: Yes
2: No. Try a larger number.
3: No. Try a smaller number.
4. Uh-oh, I forgot my number.
> """)
if option == "1":
print("I am such a genius :)!")
return(option)
elif option == "2" or option == "3":
print("I will get it next time!!!")
return(option)
elif option == "4":
print("Write it down next time!")
return(option)
else:
## When the user inputs anything other than 1, 2, 3, or 4.
print("Error. Input not recognised. Select 1, 2, 3, or 4.")
check_answer()
def restart_game():
'''
function allows user option to restart the game or to quit.
'''
option = input("""How about we play another round?
1: Yes
2: No
> """)
if option == "1":
## reinitiates the lists to empty lists
del guesses_too_large[:]
del guesses_too_small[:]
initiate_game()
elif option == "2":
## quit game
print("Thanks for playing with me!")
else:
## When the user inputs anything other than 1 or 2.
print("Error. Input not recognised. Select 1 or 2.")
restart_game()
initiate_game()
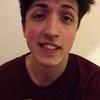
samuel fiori
4,497 PointsFirst of all, really nice code. One question: how can you return a function before you defined it like you've done?? Just got obsessed by that so I had to ask. Thank's for reply :) """
computer starts to guess
computer_guess(a, b)
def computer_guess(a, b): """

Endrin Tushe
4,591 PointsThanks Jeff,
This is very helpful can you explain the logic around: '''if comp_guess in guess_list: continue ''' The way I understand it is if comp_guess random number is already in my guess list then skip and guess another number. Is that correct? If that's the case how does the guess_list.append work? If guess_list is defined outside of while loop how does append method work if it's applied within the else block of the while loop?
A couple of other things I formatted the bottom part of my code that asks the player if they want to reveal the number and if they want to play again. In the first version, if the computer guesses correctly, the reveal prompt showed up which is obviously not what I wanted. The other thing I added was to restrict the user from entering a number that was not in range 1-10. If the player does something like that the computer prints out a statement and ends the game. Check out my new code and let me know what you think. If you have any other ideas for improvements let me know:
''' import random
def my_game(): guess_list=[] my_secret_number = int(input(''' Enter a secret number between 1 - 10 the computer will try five times to guess your number >>>>: '''))
while my_secret_number in list(range(1,11)):
while len(guess_list) < 5:
comp_guess = random.randint(1,10)
if comp_guess in guess_list:
continue
elif comp_guess == my_secret_number:
print("Your number is {}.I knew I could get it!".format(my_secret_number))
break
elif comp_guess < my_secret_number:
print("I am guessing {}, but I think that's too low. Let me try higher".format(comp_guess))
else:
print("I am guessing {} but I think that's too high. Let me try lower".format(comp_guess))
print ("Shucks! I didn't get it!")
guess_list.append(comp_guess)
else:
reveal_prompt = input("Will you tell me what your number is? Press Y/n.")
if reveal_prompt.upper() == "Y":
print ("The secret number is: {}".format(my_secret_number))
break
else:
second_chance = input("Okay---I see how it is! Will you let me play again? Press Y/n: ")
if second_chance.upper() == "Y":
my_game()
else:
print("Well fine be that way...I hope you and your secret number are happy together!")
break
else:
print("Are you trying to trick me?! We both know that {} is not in range 1-10".format(my_secret_number))
my_game() '''
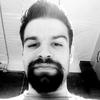
Jeffery Austin
8,128 PointsSure, I would love to explain. Because this piece of code below is nested in your while loop, what happens is it is evaluating if the comp_guess is already in guess_list, if it is it will get to continue, continue tells Python to continue with the while loop, so it goes back to the top of the while loop. Each time through it will evaluate and continue on if the guess already exists. It won't append if comp_guess is already in guess_list because continue automatically jumps you back to the top of the while loop.
if comp_guess in guess_list:
continue
Love the new code, I was thinking about that check to see if the input is in the range of 1-10. Good call. Another great check would be to make sure someone doesn't enter something other than a number. Here is a great video on how to catch exceptions in Python: https://teamtreehouse.com/library/python-basics/logic-in-python/the-exception-to-the-rule

i4mfrank
Courses Plus Student 6,872 PointsHere is my approach: I tried to to make the guessing "smarter" by putting numbers that can be excluded after a guess into a list. Before generating a new guess I check if the guesses or excluded_numbers list contains the new guessed number, and if so, it guesses again.
import random
def game():
try:
player_number = int(input("Give the Computer a Number to guess between 1 and 20: "))
except ValueError:
print("Thats not a number!")
else:
comp_guesses = []
excluded_numbers = []
max_value = 20
tries = 5
guess = random.randint(1, max_value)
while tries > 0:
comp_guesses.append(guess)
if guess == player_number:
print("I got it! Number is {}".format(player_number))
break
else:
print("Oh now i got it wrong: {}".format(guess))
if guess > player_number:
for i in range(guess, max_value + 1):
if i not in excluded_numbers:
excluded_numbers.append(i)
if guess < player_number:
for i in range(1, guess +1):
if i not in excluded_numbers:
excluded_numbers.append(i)
while guess in comp_guesses or guess in excluded_numbers:
guess = random.randint(1, max_value)
tries -= 1
else:
print("I could not solve it...")
play_again = input("Do you want to play again? Y/n")
if play_again.lower() != 'n':
game()
else:
print("Bye!")
game()