Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial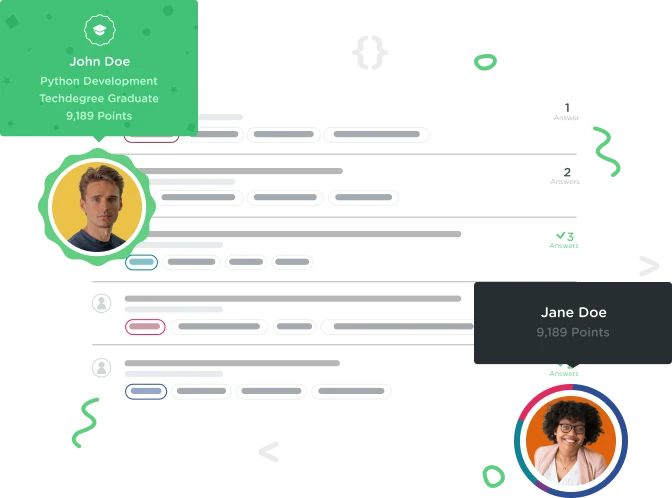

Riccardo Pressiani
Courses Plus Student 508 PointsProblem with Safari
Please, could someone tell me why this code works perfectly on Chrome and not on Safari? If I run it on Safari, the browser refresh the page with the result only once I enter "quit".
Thanks a lot!
var student;
var html = '';
String.prototype.capitalizeFirstLetter = function() {
this.toLowerCase();
return this.charAt(0).toUpperCase() + this.slice(1);
}
while(true){
student = (prompt("Type in the name of the student you want to search or type [quit] to end the research.")).capitalizeFirstLetter();
console.log(student);
if (student === null || student === 'Quit') {
html = '';
break;
}
for (var i = 0; i<students.length; i++) {
if (students[i].name === student) {
student = students[i];
html =
'<h2>Student: ' + student.name + '</h2>' +
'<p>Track: ' + student.track + '</p>' +
'<p>Achivements: ' + student.achivements + '</p>' +
'<p>Points: ' + student.points + '</p>';
print(html);
}
}
}

Riccardo Pressiani
Courses Plus Student 508 PointsYes, and here it is:
function print(message) {
var ouputHTML = document.getElementById('output');
ouputHTML.innerHTML = message;
};

John Simoneau
Courses Plus Student 8,105 PointsI agree. This is frustrating not knowing. It seems scary to learn how to properly do such a script if it won't function correctly in one of the top browsers. Hopefully someone chimes in with the answer so we know...
4 Answers
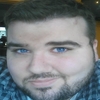
Marcus Parsons
15,719 PointsI did a little bit of testing with the Safari code with v5 Safari (all I have access to because I have Windows) and it seems that Safari uses window.print()
function to print the contents of an HTML page, which works differently than every other browser in existence because no other browser has a JavaScript function for that.
By simply changing the print function to be something else such as printst
(which I dubbed for print student), I was able to get it to successfully change the information in the output div.
I noticed, however, in your code that your print function doesn't allow for more than one student to be displayed, and this is very easily remedied. Change the line in the now printst
function from:
function printst(message) {
var ouputHTML = document.getElementById('output');
ouputHTML.innerHTML = message;
};
To this:
function printst(message) {
var ouputHTML = document.getElementById('output');
//Added a + to = sign so that each student searched for will be added to the HTML already present
ouputHTML.innerHTML += message;
};
One more error that seems to be strictly Safari is that you can't hit cancel because Safari doesn't return null for an empty prompt. It returns an empty string. So, you can either add an extra or statement for the empty string or go the route that takes care of both null and empty strings (and any other potential wonky prompt returns in other browsers):
if (!student || student === 'Quit') {}
//Or:
//The above is the same as this
//if (student === null || student === 'Quit' || student === "" || student === false || student === undefined || student === 0) { }

John Simoneau
Courses Plus Student 8,105 PointsThe safari print fix didn't work for me. I've pasted what I tried below. You are correct that hitting the cancel button in Safari doesn't work either but unfortunately your fixes caused other issues like causing the prompt to close after entering "Jody" for instance when it should continue to remain open for additional name searches. Also, if I entered the wrong name it would just close the prompt box returning nothing.
var message = '';
var student;
var search;
function printst(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if ( student.name === search ) {
message = getStudentReport( student )
printst(message);
}
}
}

John Simoneau
Courses Plus Student 8,105 PointsOk, I just realized the code I'm working on is different from the original person posting. My bad. I'm sure the prompt cancel button issue wasn't resolved by your fix because I was putting it in a different place with the search var rather then student...LOL. Well, I found just adding the code below with the rest of the if statement worked fine to get the cancel button to work. Think it's just a different way to say your student === 0 suggestion anyhow.
search.length === 0 ||
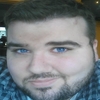
Marcus Parsons
15,719 PointsI didn't suggest to put that, though. I said that "!student" was equal to most of those things in the commented out if.
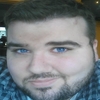
Marcus Parsons
15,719 Points"!student" checks to make sure that the "student" variable is not equal to any falsey values: null, undefined, "", NaN, 0, or false. Therefore, you wouldn't actually use that bottom if statement lol.

John Simoneau
Courses Plus Student 8,105 PointsMy bad again. Well, that solution was doomed to fail in my case regardless since I was working with the word search and not students.
I just don't want anyone to think your suggestion was wrong. I was just being a dope pasting the wrong var etc
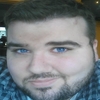
Marcus Parsons
15,719 PointsThe code was adapted to fit the questioner's code. But, all you have to do is replace "student" with "search" and it'll work just the same. "search === null" is too specific and doesn't cover the various ways that prompts return a cancel action. I would stick with "!search" for you.
Also, if you run your code with Safari, your print statement is not going to work. As I said, you shouldn't use that function name.

John Simoneau
Courses Plus Student 8,105 PointsBut in my code example I used printst as the function name.... not print. I thought that's what you said to do?

John Simoneau
Courses Plus Student 8,105 PointsRight. I understood that point before posting. It's why I felt stupid. I copy pasted your code so mine looked like this which uses the wrong word student rather then the correct word search.
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (!student || student === 'Quit') {
break;
}
which was a bonehead thing to do. No wonder it wasn't working and causing new issues right?...LOL.
So instead I searched my own solution in google and came up with doing this since Safari is returning an empty string:
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.length === 0 || search.toLowerCase() === 'quit') {
break;
}
But yes, doing your instructions with this works. Probably better with less code also (I added the lowercase part)
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (!search || search.toLowerCase() === 'quit') {
break;
}
Might be a cool idea for the instructor to include a fix in the notes since it appears a repeated problem for us Safari users. Then again, who knows how many browsers have various differences so guess the notes could get tedious if those differences add up.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsDid you implement the print function in another js file?