Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial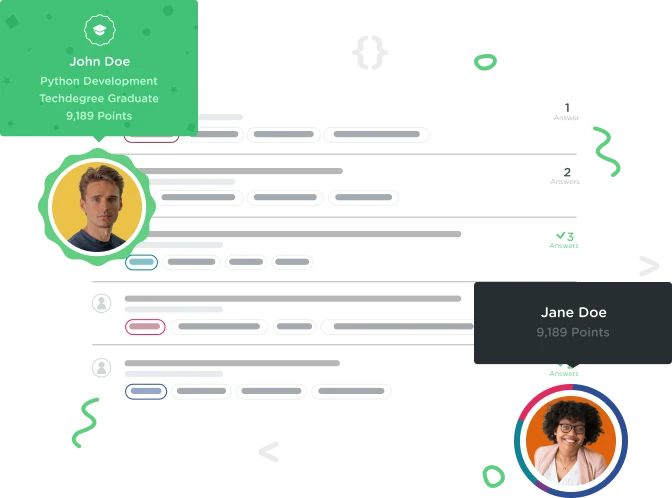

Sevak Ohanian
930 Pointsproblem with scrollview (does not show me image and text)
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".ui.StoryActivity">
<ImageView
android:id="@+id/storyImageView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:adjustViewBounds="true"
android:scaleType="fitXY"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:src="@drawable/page0" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:lineSpacingExtra="8sp"
tools:text="@string/page0"
android:textSize="16sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/storyImageView" />
<Button
android:id="@+id/choice2Button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/white"
android:textColor="#3A8AEC"
android:textSize="16sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
tools:text="@string/page0_choice2" />
<Button
android:id="@+id/choice1Button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/white"
android:textColor="#3A8AEC"
android:textSize="16sp"
app:layout_constraintBottom_toTopOf="@+id/choice2Button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
tools:text="@string/page0_choice1" />
<ScrollView
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintBottom_toTopOf="@+id/choice1Button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical" />
</ScrollView>
</android.support.constraint.ConstraintLayout>
When i move the image and text to the scrollview, everything turns white. I have checked and made sure all of my layout bounds are correct and do not know what is causing this problem.
2 Answers

Lauren Moineau
9,483 PointsThe main problem is the width of the 2 views (ImageView and TextView), which is set to 0dp. While, in a ConstraintLayout, "0dp" makes the view match its constraints, in a LinearLayout (without a combination with a weight attribute), setting a width to 0dp will just make the view vanish, as you set the width to nothing. So, when you move the ImageView and the TextView inside the ScrollView:
- Set the layout_width for both to "match_parent"
- Remove any layout_constraint
- Set the layout_height of the ScrollView to 0dp as well, so it can adjust well.
You should achieve the following:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".ui.StoryActivity">
<Button
android:id="@+id/choice1Button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/white"
android:textColor="#3A8AEC"
android:textSize="16sp"
app:layout_constraintBottom_toTopOf="@+id/choice2Button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
tools:text="@string/page0_choice1" />
<Button
android:id="@+id/choice2Button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:background="@android:color/white"
android:textColor="#3A8AEC"
android:textSize="16sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
tools:text="@string/page0_choice2" />
<ScrollView
android:layout_width="0dp"
android:layout_height="0dp"
android:fillViewport="true"
app:layout_constraintBottom_toTopOf="@+id/choice1Button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:background="@android:color/white">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<ImageView
android:id="@+id/storyImageView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:adjustViewBounds="true"
android:scaleType="fitXY"
tools:srcCompat="@drawable/page0" />
<TextView
android:id="@+id/storyTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginLeft="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginRight="16dp"
android:lineSpacingExtra="8sp"
android:textSize="16sp"
tools:text="@string/page0" />
</LinearLayout>
</ScrollView>
</android.support.constraint.ConstraintLayout>
Hope this helps
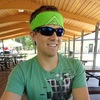
Ryan Hamblin
6,575 PointsI had the same issue and this fixed it. Thank you Elle!

Lauren Moineau
9,483 PointsYou're welcome Ryan! Happy it helped you :)
Sevak Ohanian
930 PointsSevak Ohanian
930 PointsThank you, i changed both to match_parent and the problem was fixed :)
Lauren Moineau
9,483 PointsLauren Moineau
9,483 PointsYou're welcome. Glad it's fixed :)
Oshedhe Munasinghe
8,108 PointsOshedhe Munasinghe
8,108 PointsYet this is old post but I just wanna say that this solution helps! Thanks, Lauren!