Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial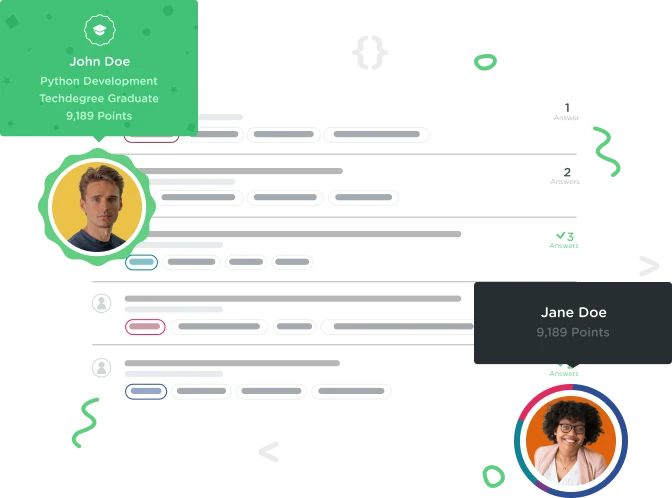
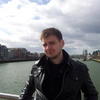
Andrej Fokin
2,057 PointsProblem with the challenge...
The task looked easy enough to do, I think. But no matter what a put in the com/teamtreehouse/KaraokeMashine.java it always replies with the syntax errors... I think it is something to do with the package imports, but a tried various imports and it just refuses to give me passage or a normal hint what did a do wrong...
Please help me, thank you.
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
import java.util.ArrayDeque;
import java.util.Map;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.ArrayList;
import java.util.Queue;
public class KaraokeMachine {
private SongBook mSongBook;
private BufferedReader mReader;
private Queue<SongRequest> mSongRequestQueue;
private Map<String, String> mMenu;
public KaraokeMachine(SongBook songBook) {
mSongBook = songBook;
mReader = new BufferedReader(new InputStreamReader(System.in));
mSongRequestQueue = new ArrayDeque<>();
mMenu = new LinkedHashMap<String, String>();
mMenu.put("add", "Adding a new song to the song book.");
mMenu.put("choose", "Choose an artist for you to sing.");
mMenu.put("play", "Play next song in the queue.");
mMenu.put("quit", "Exit the program.");
}
private String promptAction() throws IOException {
System.out.printf("There are %d song available and %d in the queue. Your options are: %n%n", mSongBook.getSongCount(), mSongRequestQueue.size());
for (Map.Entry<String, String> option : mMenu.entrySet()) {
System.out.printf("%s - %s %n", option.getKey(), option.getValue());
}
System.out.printf("%nWhat do you whant to do: ");
String choice = mReader.readLine();
return choice.trim().toLowerCase();
}
public void run() {
String choice = "";
do {
try {
choice = promptAction();
switch (choice) {
case "add":
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("New song %s is added to the song book. %n%n%n", song);
break;
case "choose":
String singerName = promptForSingerName();
String artist = promptArtist();
Song artistSong = promptSongForArtist(artist);
SongRequest songRequest = new SongRequest(singerName, artistSong);
if (mSongRequestQueue.contains(songRequest)) {
System.out.printf("%n%nWhoops! %s already requested %s! %n%n%n", singerName, artist);
break;
}
mSongRequestQueue.add(songRequest);
System.out.printf("You chose: %s %n%n%n", artistSong);
break;
case "play":
playNext();
break;
case "quit":
System.out.println("Thank you come again!");
break;
default:
System.out.printf("Unknown command '%s'. Try again. %n%n%n", choice);
break;
}
} catch (IOException ioe) {
System.out.println("Problem with input.");
ioe.printStackTrace();
}
} while (!choice.equals("quit"));
}
private String promptForSingerName() throws IOException {
System.out.printf("Enter the singer's name: ");
return mReader.readLine();
}
public Song promptNewSong() throws IOException {
System.out.printf("%nEnter the artists name: ");
String artist = mReader.readLine();
System.out.printf("Enter the title: ");
String title = mReader.readLine();
System.out.printf("Enter the video URL: ");
String videoUrl = mReader.readLine();
return new Song(artist, title, videoUrl);
}
private String promptArtist() throws IOException {
System.out.printf("%nAvailable artists: %n");
List<String> artists = new ArrayList<>(mSongBook.getArtists());
int index = promptForIndex(artists);
return artists.get(index);
}
private Song promptSongForArtist(String artist) throws IOException {
List<Song> songs = mSongBook.getSongsForArtist(artist);
List<String> songTitles = new ArrayList<>();
for (Song song : songs) {
songTitles.add(song.getTitle());
}
System.out.printf("%nAvaliable songs for %s: %n", artist);
int index = promptForIndex(songTitles);
return songs.get(index);
}
private int promptForIndex(List<String> options) throws IOException {
int counter = 1;
for (String option : options) {
System.out.printf("%d. ) %s. %n", counter, option);
counter++;
}
System.out.printf("%nYour choice: ");
String optionAsString = mReader.readLine();
int choice = Integer.parseInt(optionAsString.trim());
return choice - 1;
}
public void playNext() {
SongRequest songRequest = mSongRequestQueue.poll();
if (songRequest == null) {
System.out.printf("%n%n%nThere are no more songs in the queue! %n%n%n%n");
} else {
Song song = songRequest.getSong();
System.out.printf("%n%n%nReady %s? Open %s to hear %s by %s. %n%n%n%n", songRequest.getSingerName(), song.getVideoUrl(), song.getTitle(), song.getArtist());
}
}
}
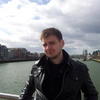
Andrej Fokin
2,057 PointsNo... I don't know where is the problem. I think I tried everything.

Iain Diamond
29,379 PointsAndrej,
I can't tell by looking at the compile errors what the problem is, however, if you could post all your project files (perhaps in a workspace, and post it as a snapshot) then I can take a closer look.
In the meanwhile, one strategy I use when fixing bugs is: Simplify.
That is, comment out/remove/delete your code until you have something that works. Assuming you're working iteratively, comment out all your new changes, and progressively add new sections of code back, testing as you go.
The sorry truth is, if you have a huge pile of mess that doesn't work there's no point in tweaking parts of your code hoping the solution will magically happen. Simply, until something works then move forward.
Hope this helps, iain
2 Answers
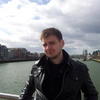
Andrej Fokin
2,057 PointsThank you all for your time and help, I solved the challenge by simply importing Craigs class files to the KaraokeMachine class like this:
package com.teamtreehouse;
import com.teamtreehouse.model.Song; // needed this.
import com.teamtreehouse.model.SongBook; // this.
import com.teamtreehouse.model.SongRequest; // and this!

Iain Diamond
29,379 PointsHi Andrej, I replaced your source in my working version with no errors found.
What compiler errors are you getting?
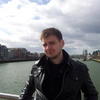
Andrej Fokin
2,057 Points./com/teamtreehouse/KaraokeMachine.java:15: error: cannot find symbol private SongBook mSongBook; ^ symbol: class SongBook location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:17: error: cannot find symbol private Queue mSongRequestQueue; ^ symbol: class SongRequest location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:20: error: cannot find symbol public KaraokeMachine(SongBook songBook) { ^ symbol: class SongBook location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:94: error: cannot find symbol public Song promptNewSong() throws IOException { ^ symbol: class Song location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:113: error: cannot find symbol private Song promptSongForArtist(String artist) throws IOException { ^ symbol: class Song location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:54: error: cannot find symbol Song song = promptNewSong(); ^ symbol: class Song location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:61: error: cannot find symbol Song artistSong = promptSongForArtist(artist); ^ symbol: class Song location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:62: error: cannot find symbol SongRequest songRequest = new SongRequest(singerName, artistSong); ^ symbol: class SongRequest location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:62: error: cannot find symbol SongRequest songRequest = new SongRequest(singerName, artistSong); ^ symbol: class SongRequest location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:102: error: cannot find symbol return new Song(artist, title, videoUrl); ^ symbol: class Song location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:114: error: cannot find symbol List songs = mSongBook.getSongsForArtist(artist); ^ symbol: class Song location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:117: error: cannot find symbol for (Song song : songs) { ^ symbol: class Song location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:143: error: cannot find symbol SongRequest songRequest = mSongRequestQueue.poll(); ^ symbol: class SongRequest location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:148: error: cannot find symbol Song song = songRequest.getSong(); ^ symbol: class Song location: class KaraokeMachine 14 errors
Caleb Hagans
7,378 PointsCaleb Hagans
7,378 PointsAndrej, Did you get it working yet?