Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial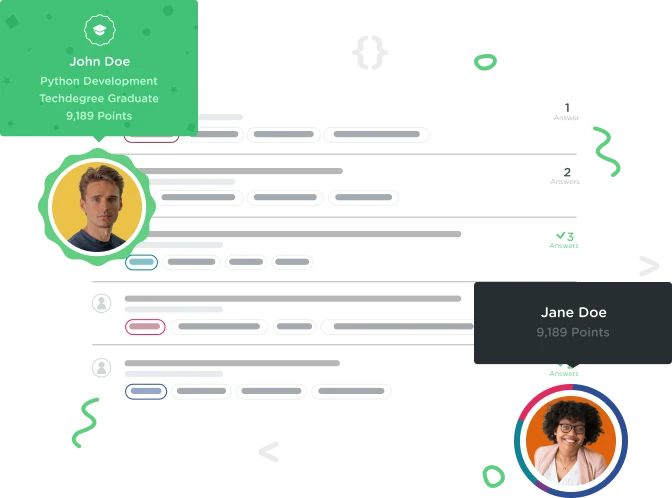

Nidhi Saini
2,627 PointsProblem with this code challenge
Inside the done() method of the callback, add an if statement to check if the ParseException e is null. If it is not null, log the message "ERROR" using the Log.e() method. Donβt forget to use TAG as the first parameter!
import android.app.Activity;
import android.util.Log;
import com.parse.ParseUser;
import com.parse.ParseException;
import com.parse.SignUpCallback;
public class SignUpActivity extends Activity {
public static final String TAG = SignUpActivity.class.getSimpleName();
protected SignUpCallback mSignUpCallback = new SignUpCallback() {
@Override
public void done(ParseException e) {
}
};
// onCreate() and other code has been omitted
protected void signUp(String username, String password) {
ParseUser newUser = new ParseUser();
newUser.setUsername(username);
newUser.setPassword(password);
newUser.signUpInBackground(new SignUpCallback(){
public void done(ParseException e)
{
if(e == null)
{
//success
}
else{
Log.e(TAG , "ERROR");
}
}
});
}
}
http://teamtreehouse.com/library/creating-a-new-user-on-parse
2 Answers

Nidhi Saini
2,627 PointsThanks Ernest for redirecting me . your reply was really helpful.

Ernest Grzybowski
Treehouse Project ReviewerNidhi Saini you are really close! There is only one issue that I can see here.
First, is that it's asking you to type this code in the done()
callback. You went ahead and tried to create your own. Type the code in the callback that is set up for you.
Secondly, It asks you to do a check to see If it is not null
. (This is just a helpful tip. Your if else
should pass the challenge.) You could do something like this.
The boolean comparator !=
means: not equal to.
if (e!=null){
//Not null code here
//Log.e()...
}
Hope this helps. Keep coding!
Ronny Perez
29,956 PointsRonny Perez
29,956 PointsHey you think you can help me I'm stuck in the same problem. I'm not asking for the answer all I need is some direction. Please!
Ernest Grzybowski
Treehouse Project ReviewerErnest Grzybowski
Treehouse Project ReviewerStart a new question in the Android Forum. I'll keep an eye out for a question from you.