Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial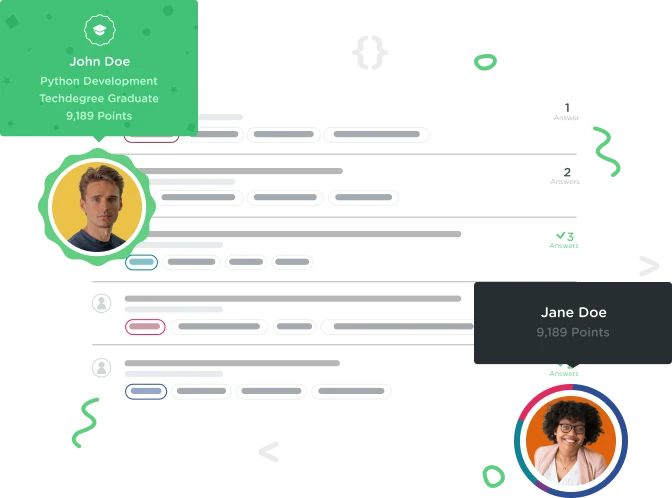
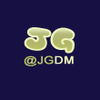
Jonathan Grieve
Treehouse Moderator 91,253 PointsProblem with Traversing.
Well for my own part I'll probably just go back to my forked workspace for third time lucky, but I thought I'd open it up for the forum anyway. I've got some HTML and CSS. This is from the Interactive Web Pages with Javascript course. Stage 3.
<!DOCTYPE html>
<html>
<head>
<title>Todo App</title>
<link href='http://fonts.googleapis.com/css?family=Lato:300,400,700' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="style.css" type="text/css" media="screen" charset="utf-8">
</head>
<body>
<div class="container">
<p>
<label for="new-task">Add Item</label><input id="new-task" type="text"><button>Add</button>
</p>
<h3>Todo</h3>
<ul id="incomplete-tasks">
<li><input type="checkbox"><label>Pay Bills</label><input type="text"><button class="edit">Edit</button><button class="delete">Delete</button></li>
<li class="editMode"><input type="checkbox"><label>Go Shopping</label><input type="text" value="Go Shopping"><button class="edit">Edit</button><button class="delete">Delete</button></li>
</ul>
<h3>Completed</h3>
<ul id="completed-tasks">
<li><input type="checkbox" checked><label>See the Doctor</label><input type="text"><button class="edit">Edit</button><button class="delete">Delete</button></li>
</ul>
</div>
<script type="text/javascript" src="app.js"></script>
</body>
</html>
//PLANNING:
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var taskIncompleteTasksHolder = document.getElementById("incomplete-task"); //incomplete-tasks;
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//Add a new task
var addTask = function() {
console.log("Add Task...");
//#new-task
//when button is pressed //create a task
//(createe a new list item with the text from #new-task.
//input checkbox
//label
//input[text]
//button .edit
//button .delete
//Each elent will need to be modified and appended.
}
//Edit an exisiting task
var editTask = function() {
console.log("Edit Task...");
//when the edit button is pressed , //if the class of the parent is .editmode
//switch from .editMode
//label text become the input's value
//switch to .editMode
//input value becomes the label's text. //toggle .editMode
}
//Delete an existing task.
var deleteTask = function() {
console.log("Delete Task...");
//when the delete button is pressed
//remove the parent list item (li)
//
}
var taskCompleted = function() {
console.log("Completed Task...");
//Mark a task as complete.
//when the checkbox is checked
//append the task list item to the #completed-tasks
}
var taskIncomplete = function() {
console.log("Incompleted Tasks. Task...");
//Mark a task as incomplete.
//when the checkbox is unchecked
//append the task to incomplete tasks.
}
//Set the click handler to the addTask Handler.
addButton.onclick = addTask;
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("bind list items...");
//select its children
// bind the editTask to edit button
// bind the deleteTask to the delete button
// bind the taskCompleted to the checkbox
}
//Cycle over the incompletesTaskHolder ul list items
for (var i=0; i < incompleteTasksHolder.children.length; i++) {
//bind events to list items children(taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//Cycle over the completeTasksHolder ul list items
//for each li
for (var i=0; i < completedTasksHolder.children.length; i++) {
// bind events to list items children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
The console keeps saying ** Uncaught ReferenceError: incompleteTasksHolder is not defined. app.js: 79**
It doesn't seem to like incompleteTasksHolder but I've tried everything I can think of to fix it.
2 Answers
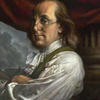
Jeff Busch
19,287 PointsHi Jonathan,
Two things: 1 & 2) The variable incompleteTaskHolder is not defined. At the top of the js code change
var taskIncompleteTaskHolder = document.getElementById("incomplete-task"); //incomplete-tasks;
To
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
Take note that Task is Tasks and incomplete-task is incomplete-tasks. The id in the html is plural.
Jeff
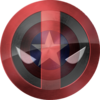
J Scott Erickson
11,883 PointsI'd have to pull down your repo and look at it to be 100% positive. And this is they type of thing that I have literally stared at for an hour trying to understand why its not working to find out I made a REALLY small mistake like this. But here is your definition of that specific variable which you then reference as "incompleteTasksHolder" which would be undefined.
var taskIncompleteTasksHolder = document.getElementById("incomplete-task"); //incomplete-tasks;
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsThanks Jeff Busch it seems to be working now. :)
I think what must have happened was I didn't reference it properly in the getElementById so as you said, "incomplete-tasks" not task..
I tried messing around with the variable declaration for what seemed like hours last night and getting nowhere and making sure it matched in the loop reference. Nothing seemed to work but alas I think I now have my answer. Woohoo! :)